$watch an object
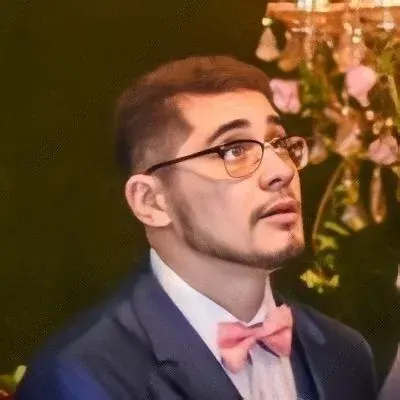
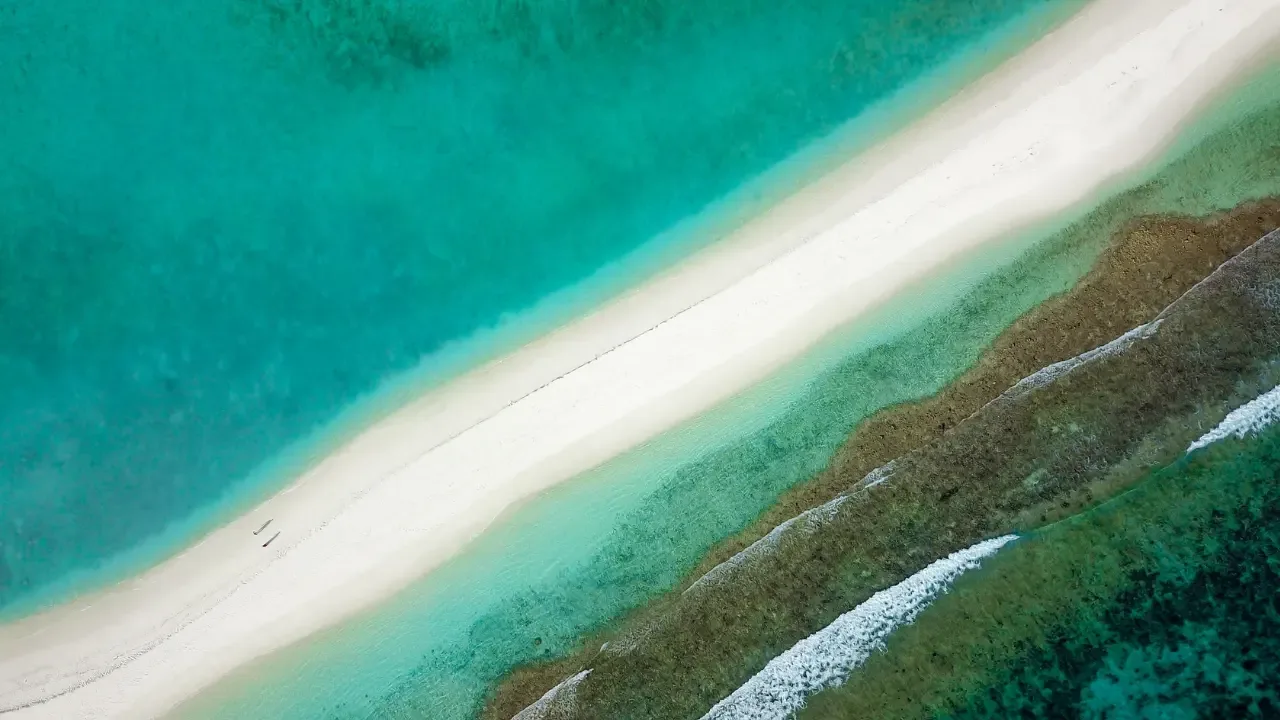
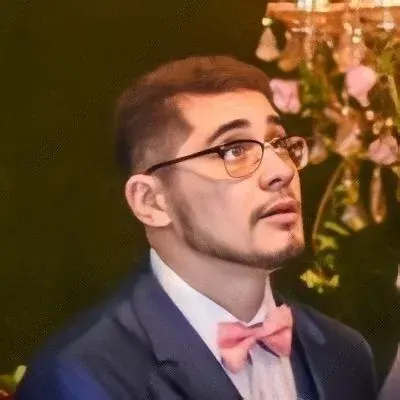
š Title: How to Properly $watch an Object in AngularJS
š Hey there, tech enthusiasts! š Are you having trouble getting the $watch
callback to work when trying to watch for changes in an object in AngularJS? š¤ Well, you're in luck because we're here to help you tackle this problem head-on! š
First, let's take a look at the code that's causing the issue:
function MyController($scope) {
$scope.form = {
name: 'my name',
surname: 'surname'
}
$scope.$watch('form', function(newVal, oldVal){
console.log('changed');
});
}
Here, the $watch
function is used to monitor changes in the form
object. The expectation is that the callback will be triggered whenever the name
or surname
properties change.
However, if you've noticed that the callback is not being called, fret not! We've got the solutions you need. š
Solution 1: Deep Watch
By default, $watch
performs a shallow comparison of the watched expression. In other words, it only checks if the reference to the form
object has changed, rather than comparing the properties within the object.
To overcome this, you can enable deep watching by passing true
as the third parameter to $watch
:
$scope.$watch('form', function(newVal, oldVal){
console.log('changed');
}, true); // Enable deep watch
By enabling deep watch, AngularJS will now perform a deep comparison of the form
object, taking into account changes in its properties. This should trigger the callback whenever name
or surname
is modified.
Solution 2: Watching Individual Properties
If you only want to monitor changes in specific properties of the form
object, you can use $watchGroup
or $watch
multiple times:
// $watchGroup example
$scope.$watchGroup(['form.name', 'form.surname'], function(newVal, oldVal){
console.log('changed');
});
// $watch example
$scope.$watch('form.name', function(newVal, oldVal){
console.log('name changed');
});
$scope.$watch('form.surname', function(newVal, oldVal){
console.log('surname changed');
});
Using $watchGroup
or multiple $watch
statements allows you to watch individual properties separately. Whenever a change occurs in either the name
or surname
property, the corresponding callback will be executed.
š Your Next Steps
Now that you've learned how to correctly use $watch
to monitor changes in an object, it's time to put your newfound knowledge into practice! š
Head over to this JSFiddle to see a live example showcasing the correct implementation of watching an object in AngularJS. Play around, make changes, and observe the magic happen! āØ
If you encounter any issues or have further questions, feel free to comment below or reach out to us on Twitter ā we're always here to help! š¤
Happy coding! š»āØ