Wait until all jQuery Ajax requests are done?
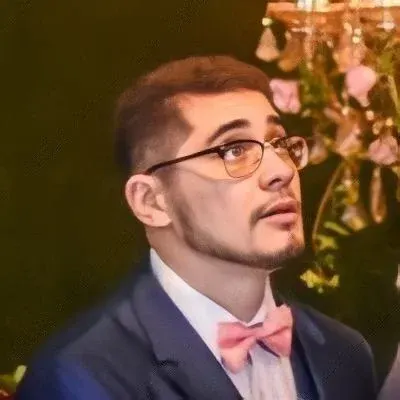
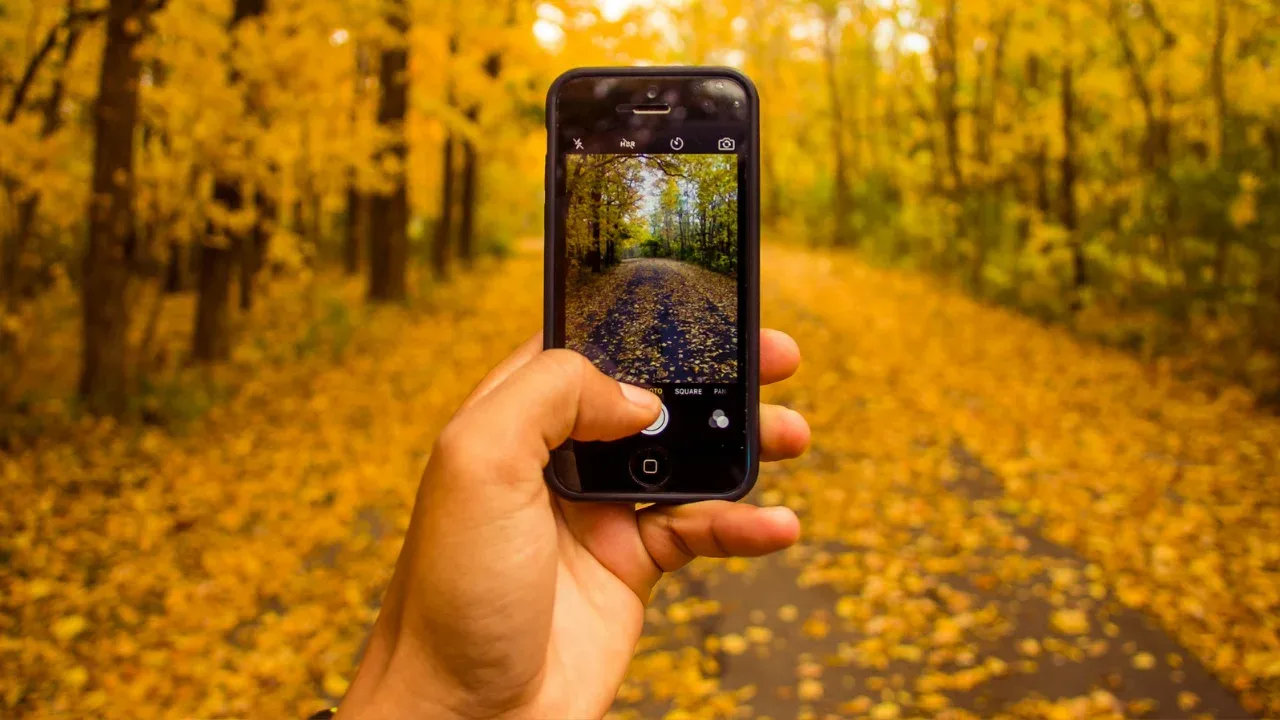
šš” Wait Until All jQuery Ajax Requests Are Done: A Guide for You! š
š Hey there, tech enthusiasts! Today, we're diving into a common issue that many developers face: how to make a function wait until all jQuery Ajax requests are done inside another function. š¤
So, imagine this situation: you have a function that triggers multiple Ajax requests, but you want to ensure that the function waits until all requests are completed before moving forward. Fear not, my friend, we've got you covered with some easy, yet powerful solutions! šŖ
The Problem Statement š
To put it simply, you need to hold off executing your next piece of code until all Ajax requests have been completed. It's crucial to avoid any race conditions and ensure that all data has been properly fetched or updated.
The Solution(s) š”
1. Callbacks (Old School) šļø
One way to tackle this is by using callbacks. Instead of writing code that sequentially progresses, you can define a function as a callback parameter, which will be triggered once the Ajax request is finished. This ensures proper order execution.
function makeAjaxRequest(url, callback) {
$.ajax({
url: url,
success: function (data) {
// Do something with the data
// Call the callback function
callback();
},
error: function (error) {
// Handle the error
},
});
}
function handleMultipleRequests() {
// Request 1
makeAjaxRequest("https://your-url1.com", function () {
// Request 2
makeAjaxRequest("https://your-url2.com", function () {
// All requests finished, execute next code here
});
});
}
2. Promises (Modern Approach) š
If you prefer more modern and elegant code, Promises are here to save the day! This approach allows you to chain Ajax requests and handle them in a clean, synchronous manner. It also provides error handling along the way.
function makeAjaxRequest(url) {
return new Promise(function (resolve, reject) {
$.ajax({
url: url,
success: function (data) {
// Do something with the data
resolve();
},
error: function (error) {
reject(error);
},
});
});
}
function handleMultipleRequests() {
// Request 1
makeAjaxRequest("https://your-url1.com")
.then(function () {
// Request 2
return makeAjaxRequest("https://your-url2.com");
})
.then(function () {
// All requests finished, execute next code here
})
.catch(function (error) {
// Handle any errors occurred during the requests
});
}
The Power of Choice āØ
Both the callback and Promises approaches have their strengths, and your choice depends on your project's needs and your personal coding style.
Conclusion š
Waiting until all jQuery Ajax requests are done is a common challenge, but with callbacks or Promises, you can easily overcome it. So don't let those asynchronous requests intimidate you - instead, embrace them and use these solutions to streamline your code! š
Now it's your turn! Have you faced any hurdles in dealing with multiple Ajax requests? Share your experiences and thoughts in the comments below. Let's learn and grow together! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
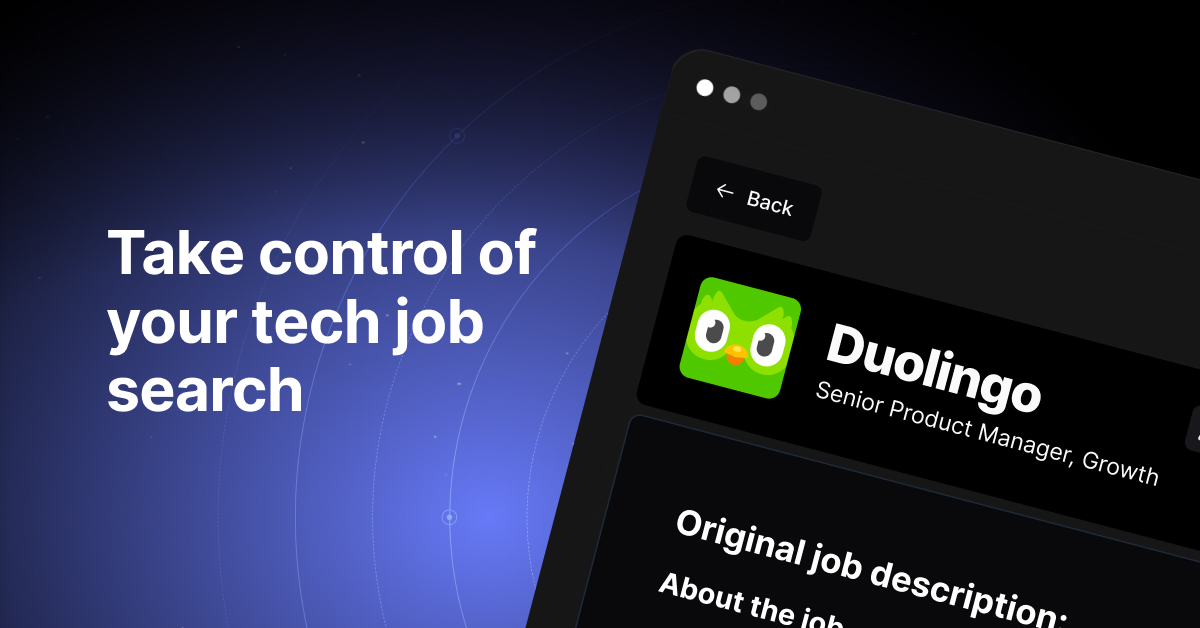