Vue JS returns [__ob__: Observer] data instead of my array of objects
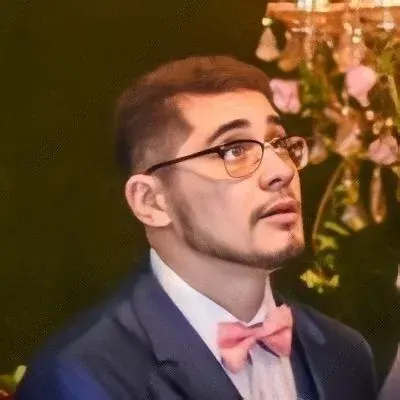
![Cover Image for Vue JS returns [__ob__: Observer] data instead of my array of objects](https://images.ctfassets.net/4jrcdh2kutbq/4LzpjqnGluxbuQcWmbAGX0/5d51a487df5b06a3f30146f2e7a52efb/Untitled_design__3_.webp?w=3840&q=75)
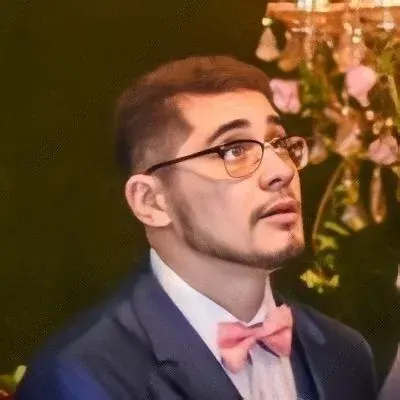
🐦 Solving the Vue JS [ob: Observer] Issue
So, you're new to Vue JS and JavaScript, and you are facing an issue where instead of getting an array of objects from your API call, you are getting [__ob__: Observer]
data. Fret not! In this guide, we will address this common issue, provide easy solutions, and get you flying in no time! ✨
The Scenario
Let's start by understanding the context. You have a page where you want to fetch data from the database using an API call. You've tested the API call with Postman, and it returns the correct JSON data. However, when you try to use Vue JS to display the data, you encounter the dreaded [__ob__: Observer]
instead of your array of objects. Let's fix that!
Understanding the Issue
To understand the issue, let's take a look at the code you shared. In your Vue template file, you have a data
section where you define your pigeons
array. Inside the created
lifecycle hook, you call the fetchPigeons
method to fetch the data from the API endpoint. However, when you log this.pigeons
to the console, you see the Observer data instead of your expected array.
The Root Cause
The issue you are facing is due to Vue's reactivity system. Vue wraps your data objects with an Observer to track changes and facilitate reactivity. This is what causes the [__ob__: Observer]
to appear in your output.
The Solution
To access your actual array of objects, you need to use the this.pigeons
array within your Vue component methods or computed properties. It will automatically unwrap the Observer and give you the desired array. The [__ob__: Observer]
is only shown when you directly log the entire data
object to the console.
For example, in your fetchPigeons
method, you correctly assign res.data
to this.pigeons
, so accessing it within that method will give you the expected array. However, when you log it outside the method or in the created
hook, you will see the Observer object.
Updated Code
To summarize, here's an updated version of your Vue template file:
<template>
<div>
<h2>Pigeons in the racing loft</h2>
<div class="card-content m-b-20" v-for="pigeon in pigeons" :key="pigeon.id">
<h3>{{ pigeon.id }}</h3>
</div>
</div>
</template>
<script>
export default {
data() {
return {
pigeons: [],
pigeon: {
// your pigeon properties
},
pigeon_id: ''
}
},
created() {
this.fetchPigeons();
},
methods: {
fetchPigeons() {
fetch('api/racingloft')
.then(res => res.json())
.then(res => {
this.pigeons = res.data; // Unwrapped array
console.log(this.pigeons); // Expected array
})
}
}
}
</script>
With this updated code, you should now be able to retrieve and display your array of objects correctly.
Expanding the Horizon
Congratulations on fixing the Vue JS [__ob__: Observer]
issue! Now that you have a better understanding of Vue's reactivity system, you can continue exploring and building amazing applications with confidence. If you encounter any issues or have further questions, don't hesitate to reach out and seek support.
Remember, the key to success is to keep learning, experimenting, and sharing your knowledge with the community. Let's soar to new heights together! 🚀
Now, it's your turn! Have you ever encountered Vue JS reactivity issues? How did you solve them? Share your experiences and insights in the comments below and let's make Vue JS development a breeze for everyone. Happy coding! 💻✨