var functionName = function() {} vs function functionName() {}
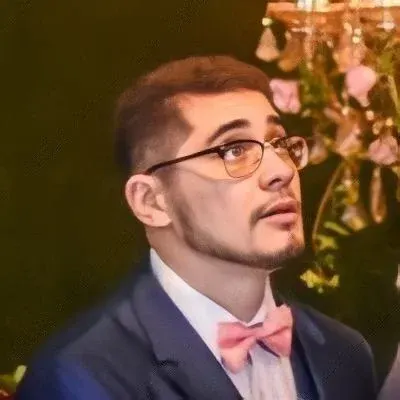
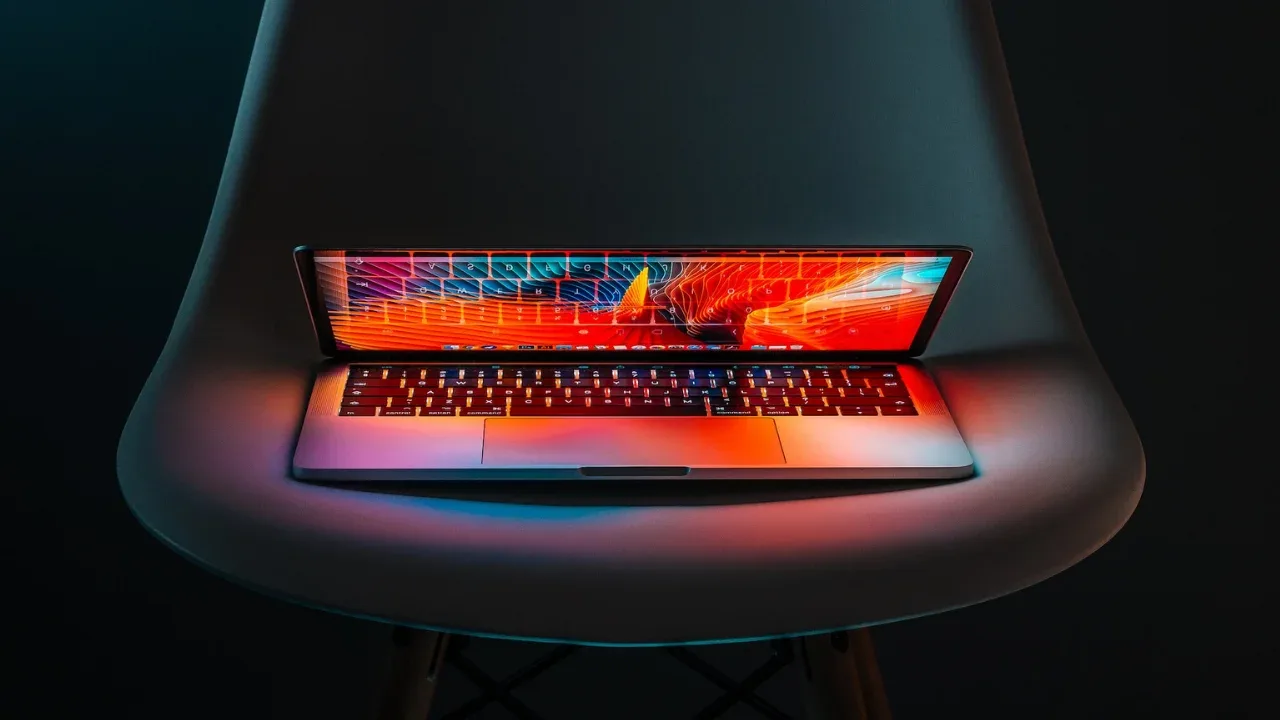
📝 Function Declaration vs Function Expression
So, you've just inherited a JavaScript codebase and stumbled upon two different ways of declaring functions: var functionName = function() {}
and function functionName() {}
. 🤔
You're scratching your head, wondering if there's any meaningful difference between these two approaches. Well, you're in luck! In this blog post, we'll dive into the reasons behind these two methods, their pros and cons, and when to use each. Let's get started! 💪
📘 Function Declaration
First, let's look at the second method: function functionName() {}
. This is called a function declaration. It's a straightforward way of defining a function. It's hoisted, which means you can call it anywhere in your code, even before its declaration.
Let's have a look at an example:
function sayHello() {
console.log("Hello, World!");
}
Here, we defined a new function named sayHello
that logs "Hello, World!" to the console. Pretty simple, right? 😎
Pros of Function Declarations:
Hoisted: You can call the function anywhere in your code, irrespective of its position.
Clearer Syntax: The function name comes before the parentheses, which makes it easy to identify and read.
Cons of Function Declarations:
Limited Flexibility: The function name must be valid as an identifier, adhering to variable naming rules.
📙 Function Expression
Now, let's move on to the first method: var functionName = function() {}
. This is called a function expression. It involves assigning a function to a variable, hence the "expression" part.
Check out this example:
var calculateSum = function(num1, num2) {
return num1 + num2;
};
In this case, we assigned an anonymous function to the variable calculateSum
. The function takes in two parameters, num1
and num2
, and returns their sum.
Pros of Function Expressions:
Flexibility: You can assign the function to any variable, allowing for more dynamic behavior.
Anonymous Functions: You can create functions without a name, useful for callbacks or immediately invoked function expressions (IIFE).
Cons of Function Expressions:
Not Hoisted: Unlike function declarations, function expressions are not hoisted. You must declare them before using them.
Slightly Confusing Syntax: The variable name comes before the assignment operator (
=
), making it slightly less readable.
✨ Which Should You Use?
Now that we've covered the basics of both function declarations and function expressions, you might be wondering, "Okay, but which one should I use?" Well, it depends on your specific use case. Here are a few guidelines to consider:
Use function declarations if you want hoisting and cleaner syntax.
Use function expressions if you need more flexibility or want to create anonymous functions.
Remember, in most cases, it's a matter of personal preference and coding style. Stick to one convention within your codebase to maintain consistency.
So, armed with this knowledge, go forth and conquer that JavaScript codebase! ✊
🤔 Did We Answer Your Question?
We hope this blog post cleared up any confusion around the differences between var functionName = function() {}
and function functionName() {}
. If you have any more questions or need further clarification, drop a comment below!
And if you found this post helpful, don't forget to share it with your fellow developers who might also be struggling with function declarations and expressions. Sharing is caring, after all! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
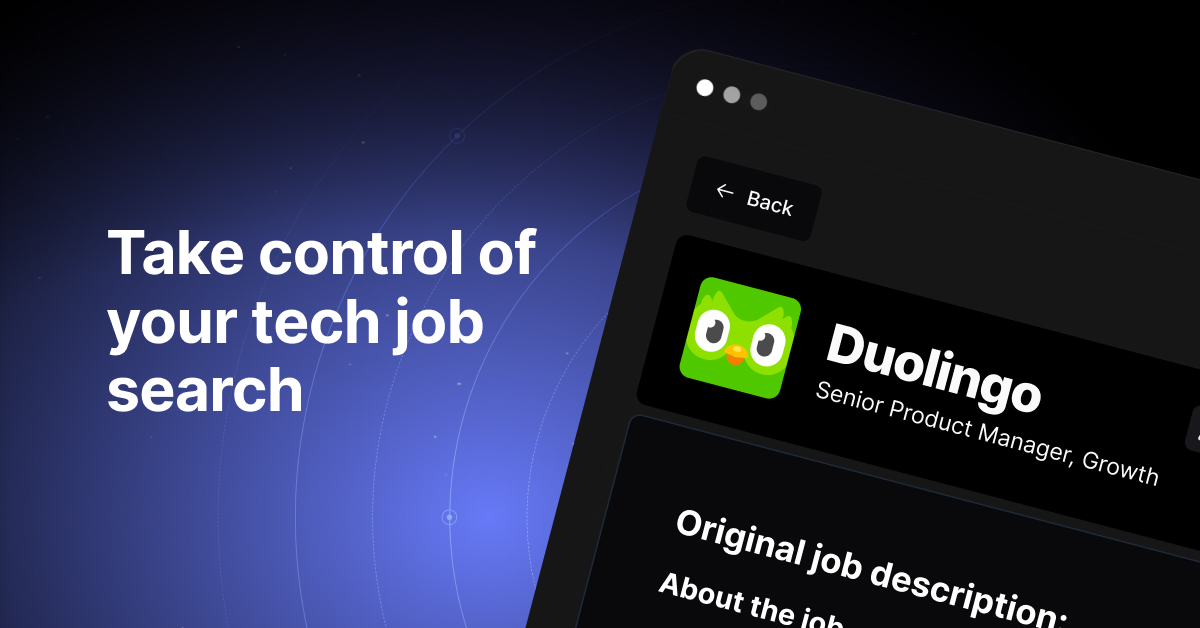