Vanilla JavaScript equivalent of jQuery"s $.ready() - how to call a function when the page/DOM is ready for it
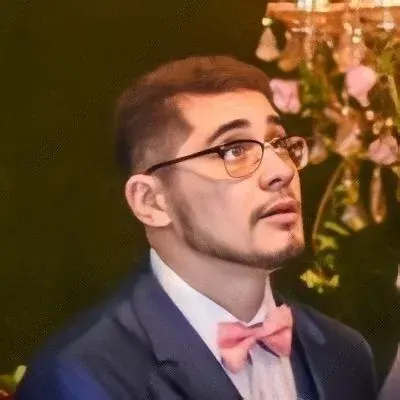
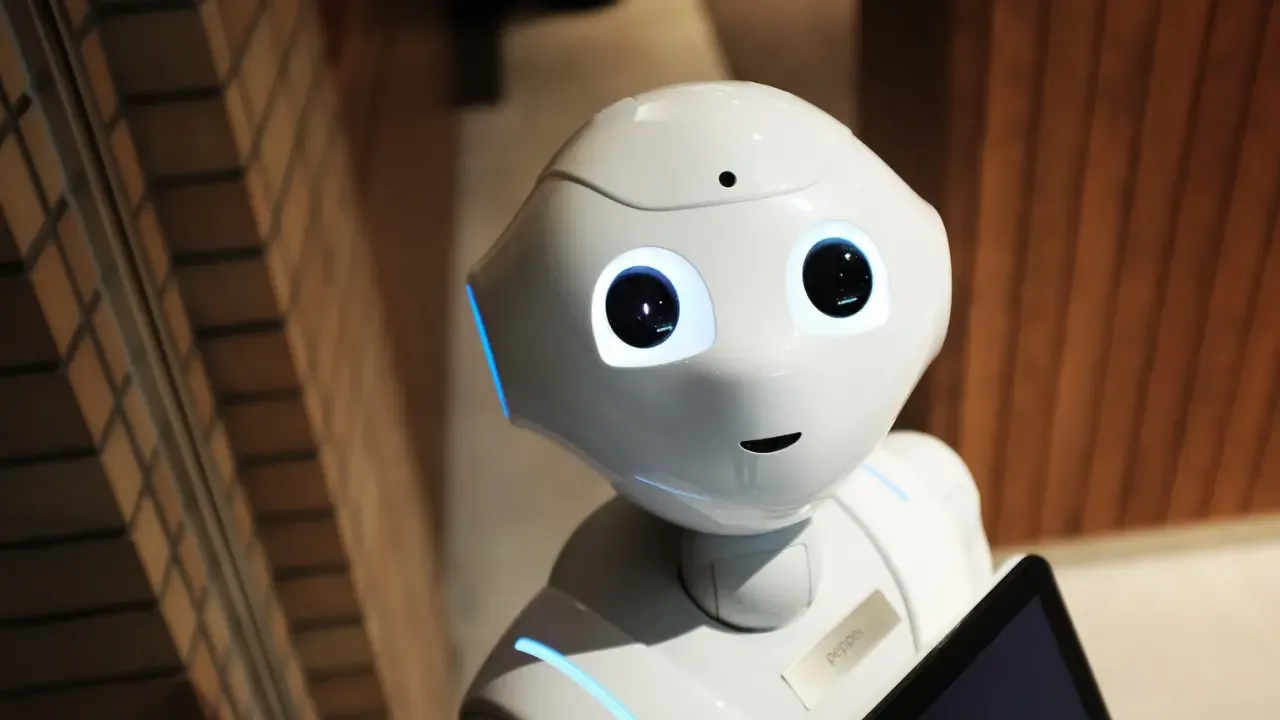
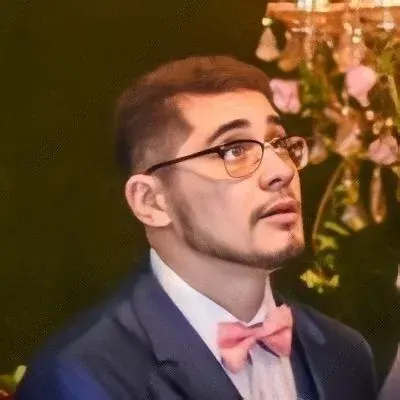
🌟 The Vanilla JavaScript Equivalent of jQuery's $.ready() 🌟
So you want to call a function when the page or DOM is ready, huh? 🤔 Fear not, my fellow JavaScript enthusiast! I've got you covered with some easy-peasy solutions. Let's dig in! 💪
The Classic Approach 🕶️
Before we explore other methods, let's start with the classic approach using window.onload
. This method ensures that your function gets executed when the entire page, including its external resources, finishes loading.
Here's an example:
window.onload = function() {
// Your code here
myFunction();
};
Easy, right? All you need to do is replace myFunction()
with your actual function, and you're good to go! 🚀
The Quick and Dirty 🔥
If you prefer a quicker way without defining an anonymous function, you can simply assign your function directly to window.onload
. Here's how it's done:
window.onload = myFunction;
Short and sweet! Just make sure to omit the parentheses when assigning your function to window.onload
. 😉
The Body Tag ➡️
Another popular approach is to use the onload
attribute on the body
tag. This way, your function will execute once the body element has finished loading. Here's an example:
<body onload="myFunction()">
This approach works well in most cases, but keep in mind that if you have multiple functions to execute, this method might get a bit messy. In that case, I recommend using one of the previous approaches.
The Bottom Script 💡
If you're more inclined towards organizing your JavaScript code at the bottom of the page, you can place your function call at the end, just before the closing body
or html
tag. Here's an example:
<script type="text/javascript">
myFunction();
</script>
With this approach, your function will be executed once the script is reached during the parsing of the HTML document.
The Cross-Browser Magic ✨
Now, it's important to make sure your solution works in all browsers, both old and new. To achieve this cross-browser compatibility, you can use the following function:
function DOMReady(fn) {
if (document.readyState === "complete" || document.readyState === "interactive") {
setTimeout(fn, 0);
} else {
document.addEventListener("DOMContentLoaded", fn);
}
}
DOMReady(myFunction);
This function checks if the DOM has already loaded. If it has, it calls the function using setTimeout
to ensure it runs asynchronously. If not, it attaches an event listener for the DOMContentLoaded
event, which fires once the DOM is ready to be manipulated.
Wrapping Up 🎁
You've learned several ways to achieve the same functionality as jQuery's $.ready()
using pure Vanilla JavaScript! 🎉 Choose the method that suits your needs and enjoy the simplicity of JavaScript without any additional libraries. 🚀
So go ahead, give it a try, and start unleashing your JavaScript magic on the web! 💪 And don't forget to share your thoughts or any other cool techniques you use in the comments below. Let's keep the conversation going! 💬
Keep coding and stay awesome! ✌️😎