Using Razor within JavaScript
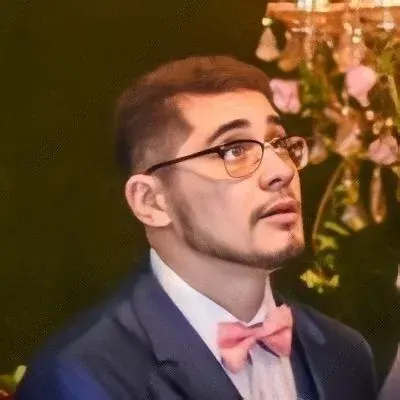
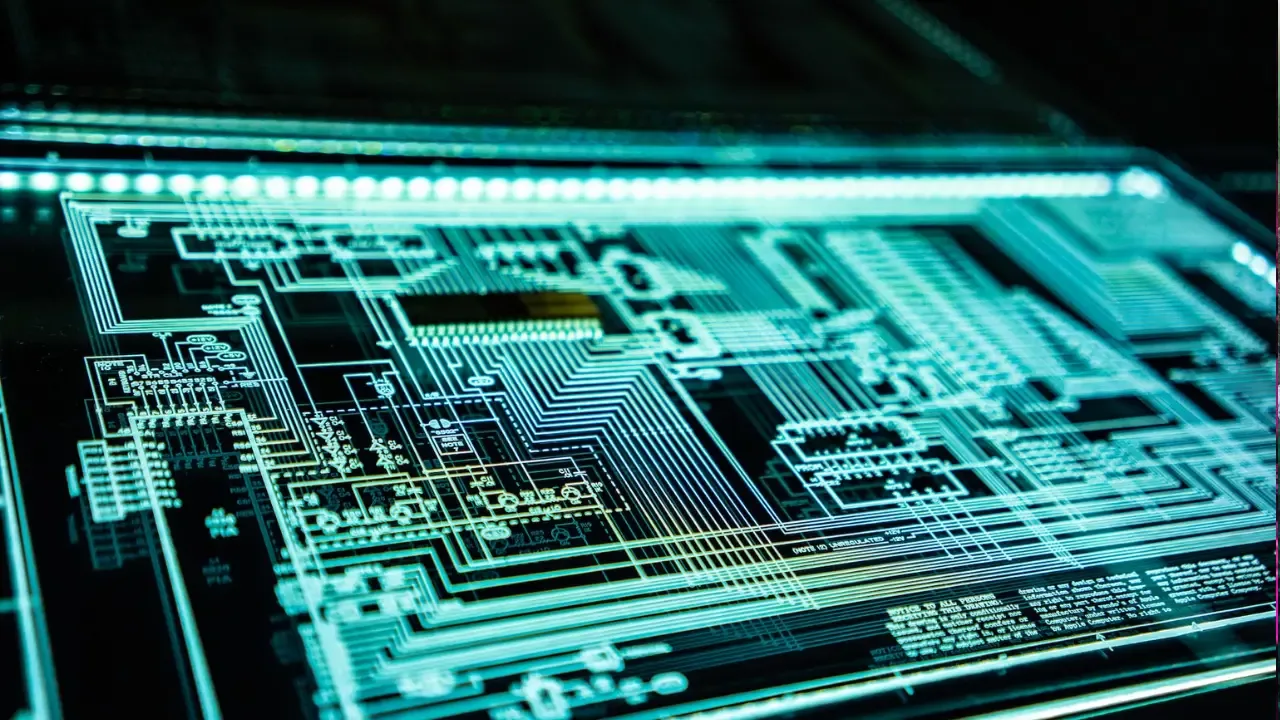
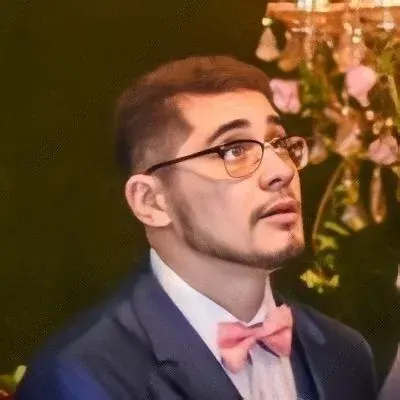
Using Razor within JavaScript: Solving the Marker Compilation Errors 😎
Have you ever wondered if it's possible to use Razor syntax within JavaScript in a view (.cshtml)? Well, look no further! In this blog post, we'll discuss a common issue when trying to add markers to a Google map using Razor within JavaScript and provide you with easy solutions to solve those pesky compilation errors.
The Problem: Compilation Errors Galore 😱
So, you're trying to add markers to a Google map using JavaScript, but you also want to utilize some Razor syntax to dynamically populate the marker information from your model. Something like this:
<script type="text/javascript">
// Some JavaScript code here to display the map, etc.
// Now add markers
@foreach (var item in Model) {
var markerLatLng = new google.maps.LatLng(@(Model.Latitude), @(Model.Longitude));
var title = '@(Model.Title)';
var description = '@(Model.Description)';
var contentString = '<h3>' + title + '</h3>' + '<p>' + description + '</p>'
// Rest of the marker code
}
</script>
However, when you try to compile this code, you're greeted with a barrage of compilation errors. 😫
The Solution: Escaping Razor Syntax 🚀
Fear not! There's an easy solution to this problem. To successfully use Razor syntax within JavaScript, you simply need to escape the Razor code using the @Html.Raw()
method. This method allows you to output raw HTML or JavaScript without any encoding.
Let's apply this solution to our marker code:
<script type="text/javascript">
// Some JavaScript code here to display the map, etc.
// Now add markers
@foreach (var item in Model) {
var markerLatLng = new google.maps.LatLng(@(Html.Raw(Model.Latitude)), @(Html.Raw(Model.Longitude)));
var title = '@(Html.Raw(Model.Title))';
var description = '@(Html.Raw(Model.Description))';
var contentString = '<h3>' + title + '</h3>' + '<p>' + description + '</p>'
// Rest of the marker code
}
</script>
By applying Html.Raw()
to our Razor expressions, we ensure that the JavaScript parser interprets them as pure JavaScript and avoids any compilation errors. 🎉
The Call-to-Action: Share Your Success! 📣
Now that you have this easy solution at your fingertips, it's time to put it into action! Try implementing the code with the escaped Razor syntax and share your successful results with us. We'd love to hear about your experiences and any other tips and tricks you may have discovered along the way.
Don't forget to spread the word by clicking that share button! Help your fellow developers overcome the Razor within JavaScript hurdle as well.
Happy coding! 😄✨