Using Razor, how do I render a Boolean to a JavaScript variable?
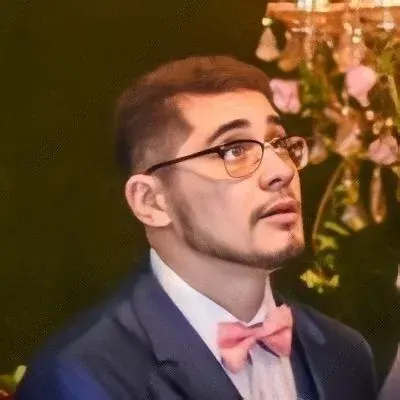
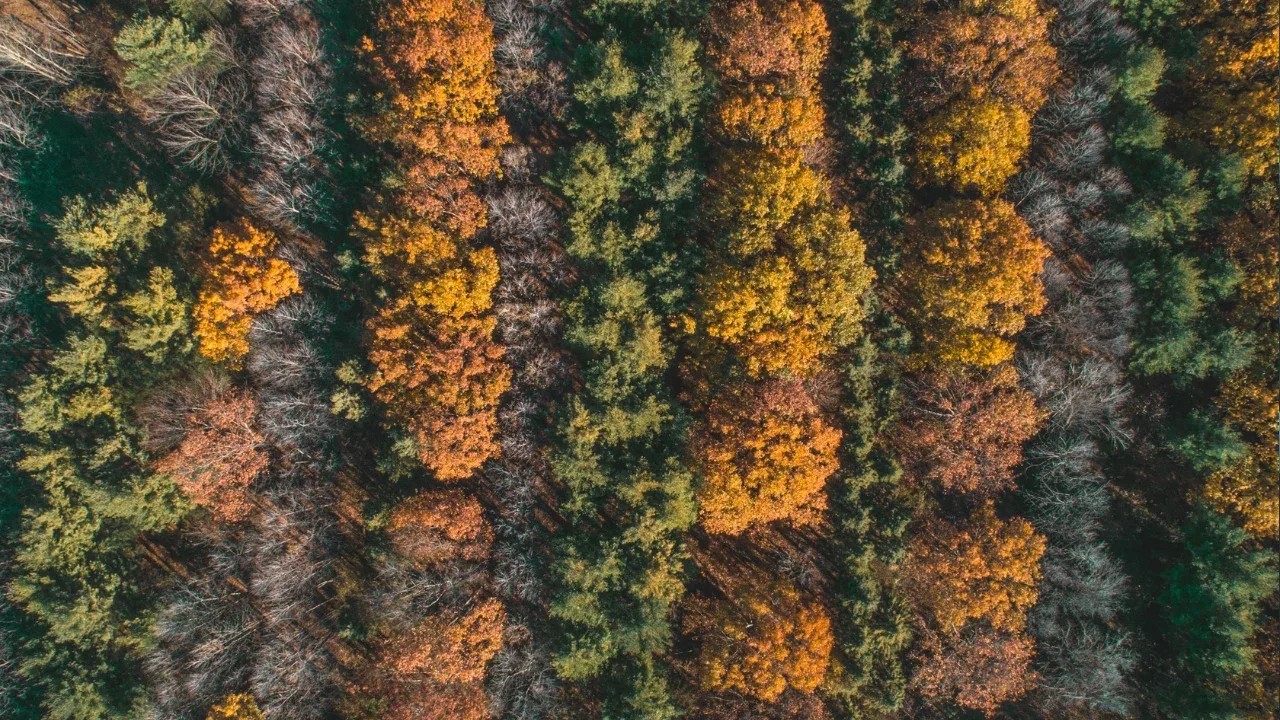
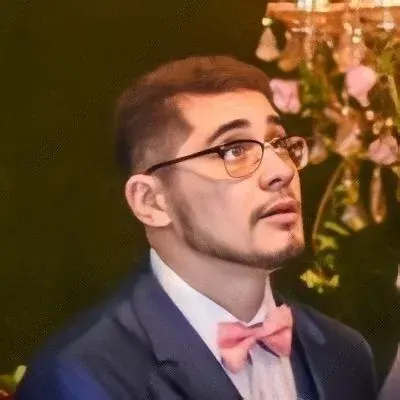
How to Render a Boolean to a JavaScript Variable Using Razor
š” Have you ever encountered a syntax error when trying to render a Boolean value to a JavaScript variable in a .cshtml
file? Don't worry, you're not alone! In this blog post, we'll address this common issue and provide easy solutions to help you overcome it. Let's dive in! š
The Problem
š¤ So, what's the problem here? The issue lies in mixing server-side C# code with client-side JavaScript code in the Razor view engine. When you try to assign a C# Boolean value directly inside a JavaScript variable, it throws a syntax error. This happens because Razor tries to interpret the Boolean value as JavaScript code, resulting in an invalid assignment.
š« Take a look at the code snippet below:
<script type="text/javascript">
var myViewModel = {
isFollowing: @Model.IsFollowing // This is a C# bool
};
</script>
āļø This will likely generate a syntax error in your .cshtml
file. Now, let's explore some easy solutions to solve this problem!
Solution 1: Using the @Html.Raw()
Method
ā
One way to overcome the syntax error is by using the @Html.Raw()
method, which prevents Razor from interpreting the Boolean value as JavaScript code. Follow the example below:
<script type="text/javascript">
var myViewModel = {
isFollowing: @Html.Raw(Json.Encode(Model.IsFollowing)) // This is a C# bool
};
</script>
š By using @Html.Raw()
, we can safely encode the Boolean value without triggering a syntax error. This ensures that Razor simply outputs the Boolean without any unnecessary interpretation.
Solution 2: Transforming the Boolean Value
ā Another solution is to transform the Boolean value into a string or integer explicitly. This way, the Razor engine won't get confused and can successfully render the value as expected. Check out this example:
<script type="text/javascript">
var myViewModel = {
isFollowing: @(Model.IsFollowing ? "true" : "false") // This is a C# bool
};
</script>
š By using the ternary operator ? :
, we convert the Boolean value into the corresponding string representation that JavaScript understands as a Boolean.
Solution 3: Utilizing JSON Serialization
ā Lastly, you can utilize JSON serialization to convert the Boolean value into a format that can be easily assigned to a JavaScript variable. Follow this example:
@{
var serializedBool = Newtonsoft.Json.JsonConvert.SerializeObject(Model.IsFollowing);
}
<script type="text/javascript">
var myViewModel = {
isFollowing: @Html.Raw(serializedBool)
};
</script>
š Here, we use the SerializeObject()
method from the popular JSON serialization library Newtonsoft.Json to convert the Boolean value into a JSON-encoded string. Then, we can safely render it into the JavaScript variable without any syntax errors.
Conclusion
š That's it! You've learned three easy solutions to render a Boolean value to a JavaScript variable using Razor. You can either use the @Html.Raw()
method, transform the Boolean value explicitly, or utilize JSON serialization. Choose the solution that fits your needs best and say goodbye to those pesky syntax errors!
š¬ We'd love to hear your thoughts. Have you experienced this issue before? Which solution worked best for you? Share your experiences and join the discussion in the comments below! š