Using Node.js require vs. ES6 import/export
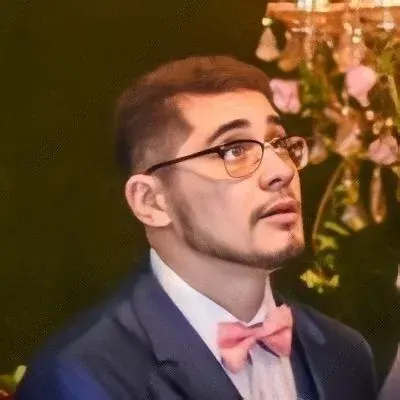
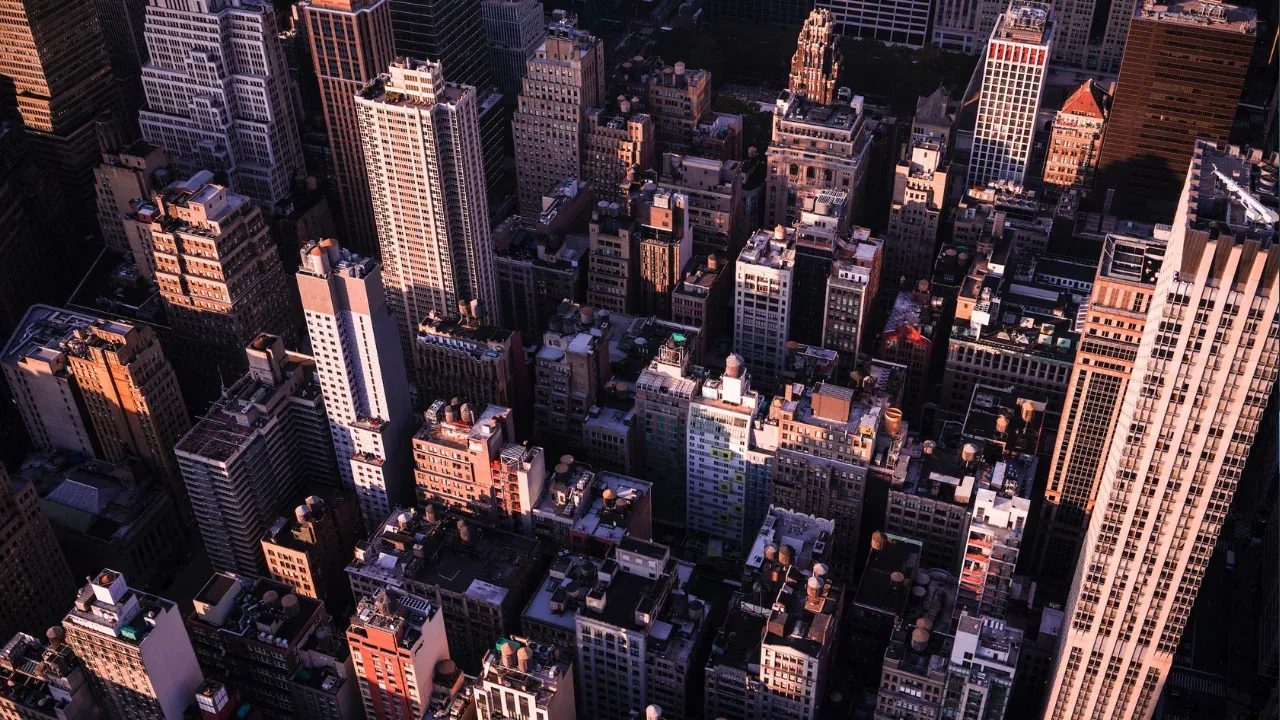
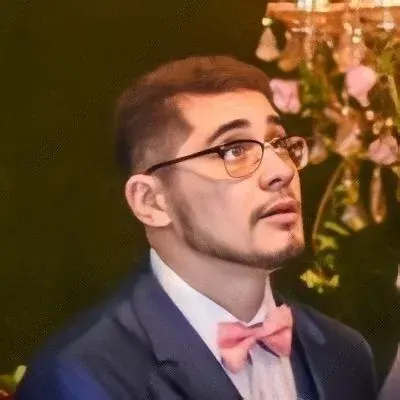
📜 Node.js require vs. ES6 import/export: Which Should You Choose?
In the world of Node.js, there are two module system options available to you: require
and ES6 import/export
. Each has its own quirks and benefits, but choosing the right one can make all the difference in your development experience. So, let's dive in and explore the common issues, easy solutions, and performance benefits associated with these options.
🤔 Common Issues and the Great Divide
When deciding between require
and ES6 import/export
, the primary concern boils down to compatibility and flexibility. The major issue you might face is that the two module systems can't work together directly. Mixing them in the same codebase leads to confusion and unexpected errors.
🐞 require
Limitations
The require
syntax is commonly used in Node.js projects and is compatible with CommonJS modules. However, it does lack certain capabilities provided by ES6 modules, such as named exports and tree-shaking. This can hinder the development of modern JavaScript applications, especially when you're working with front-end frameworks like React or Vue.js.
🌟 ES6 import/export
Advantages
ES6 modules, on the other hand, offer more features and advantages. They support both named and default exports and embrace a better syntax for importing and exporting modules. With ES6 modules, you can benefit from tree-shaking, which eliminates unused code during the bundling process, resulting in smaller file sizes and improved performance.
💡 Easy Solutions and the Right Way
To make the most informed decision, consider the following solutions and best practices when choosing between require
and ES6 import/export
:
✅ Solution 1: Using require
for Node.js-specific Code
If you're working on a Node.js-specific project without front-end frameworks involved, stick with the traditional require
syntax. This ensures compatibility and a seamless integration with the Node.js ecosystem.
Here's an example:
const express = require('express');
const logger = require('./logger');
// Your Node.js-specific code goes here...
✅ Solution 2: Transitioning to ES6 import/export
If you want to leverage ES6 features and take advantage of modern JavaScript development, consider migrating to ES6 modules. This option requires additional setup and tooling but offers future compatibility and enhanced development experience.
Here's an example:
import express from 'express';
import logger from './logger';
// Your ES6 module code goes here...
⚡️ Performance Benefits: A Glimpse into the Future
Although there may not be a significant performance difference between require
and ES6 import/export
in most scenarios, ES6 modules have an edge thanks to built-in tree-shaking capabilities. This means that during the bundling process, unused code can be eliminated, resulting in optimized and faster applications. However, it's important to note that the actual impact may vary depending on your project's complexity and size.
📣 Engage with Your Decision
Choosing the right module system for your project is vital for compatibility, productivity, and overall code health. Consider your project's unique needs and strive for a decision that aligns with your development goals. Remember, what might work for one project may not be ideal for another.
So, whether you remain loyal to require
or embrace the power of ES6 import/export
, make your decision confidently and propel your application towards the future of JavaScript development.
👉 What module system do you prefer? Share your thoughts in the comments below and let's build a vibrant discussion together! 🚀