Using Node.JS, how do I read a JSON file into (server) memory?
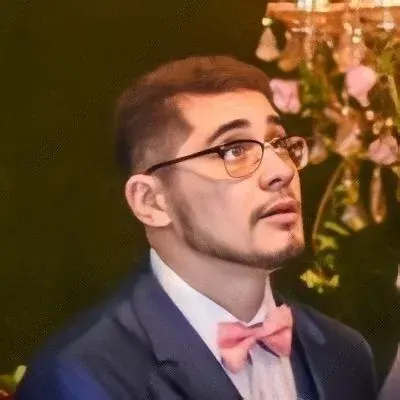
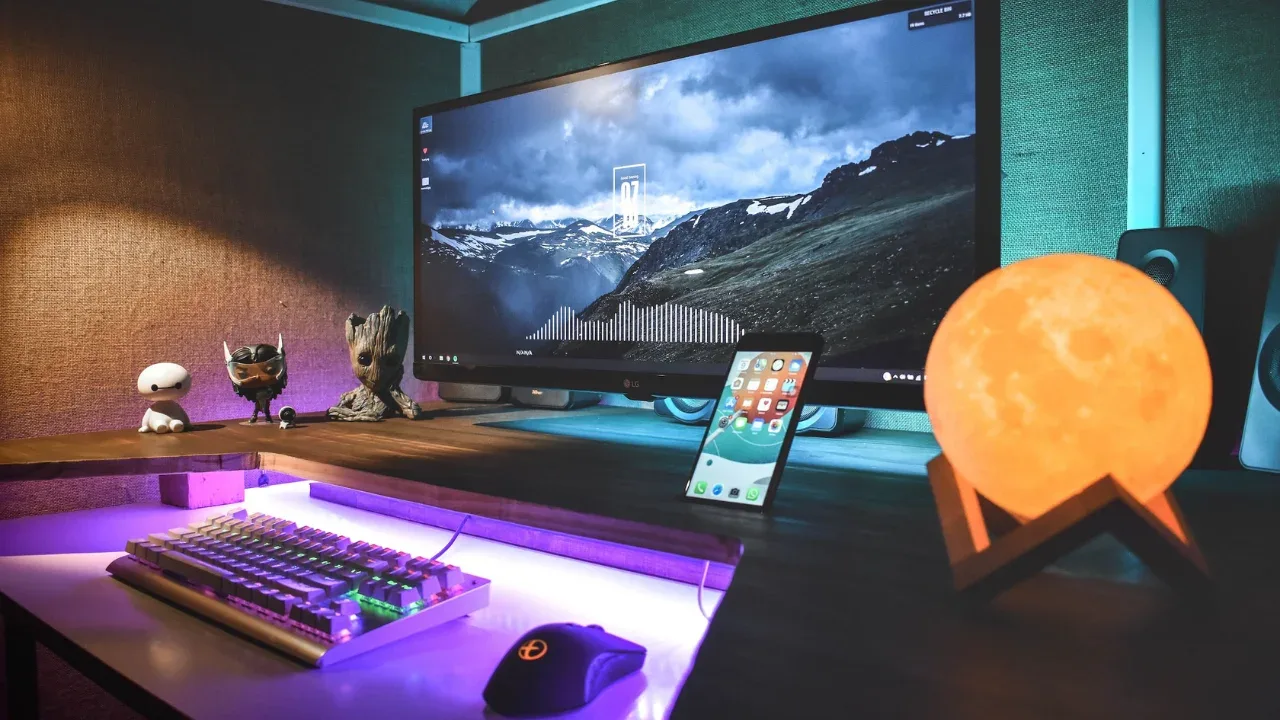
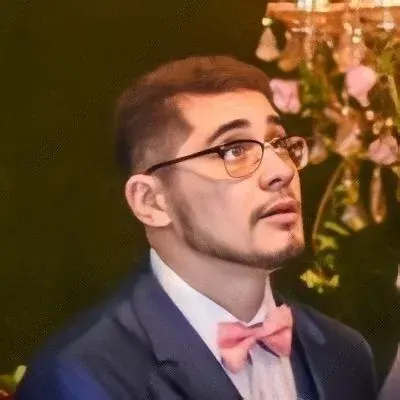
🤔 How to Read a JSON File into Memory Using Node.js? 📂
Are you experimenting with Node.js and want to read a JSON object into memory quickly? Look no further! In this blog post, we'll explore different methods to accomplish this task and provide easy solutions to potential issues. Let's get started! 💪
📝 Background
Before diving into the solutions, let's briefly discuss the background of the problem at hand. You're working with Node.js and want to access a JSON object from code efficiently. You have two options: reading from a text file or a .js file. But which one is better? Let's find out! 🕵️
🧐 Text File or .js File?
When it comes to storing JSON data, you can choose between a text file or a .js file. Each has its pros and cons, so it ultimately depends on your specific needs.
Text File
Using a text file to store your JSON data is a simple and straightforward approach. Here's how you can read a JSON object from a text file in Node.js:
const fs = require('fs');
fs.readFile('data.json', 'utf8', (err, data) => {
if (err) throw err;
const jsonData = JSON.parse(data);
console.log(jsonData);
});
In this example, we use the fs
module's readFile
function to read the contents of the data.json
file. Once we have the file's data, we can parse it using JSON.parse
to convert it into a JavaScript object.
.js File
Another option is to store your JSON data in a .js file. This approach allows you to directly export the JSON object as a module in Node.js. Here's an example:
// data.js
module.exports = {
name: 'John Doe',
age: 25,
city: 'Example City',
};
// main.js
const jsonData = require('./data');
console.log(jsonData);
In this example, we define a JSON object in the data.js
file and export it as a module using module.exports
. Then, in our main.js
file, we use require
to import the JSON object as jsonData
.
🚀 Reading JSON into Server Memory
Now that we've discussed the options, let's move on to the main question: how to read a JSON object into server memory using JavaScript/Node? The answer lies in our previous explanations:
If you choose to use a text file, utilize the
readFile
function from thefs
module to read the file's contents and parse it usingJSON.parse
.If you prefer using a .js file, simply use
require
to import the JSON object as a module.
Choose the method that suits your requirements best! 🌟
✨ Call to Action
Congratulations! You've learned how to read a JSON file into server memory using Node.js. Now it's time to put your knowledge into action and experiment with different JSON files. Share your experiences and any other tips you have in the comments below. Let's foster a community of Node.js enthusiasts! 🙌
Happy coding! 💻