Using async/await with a forEach loop
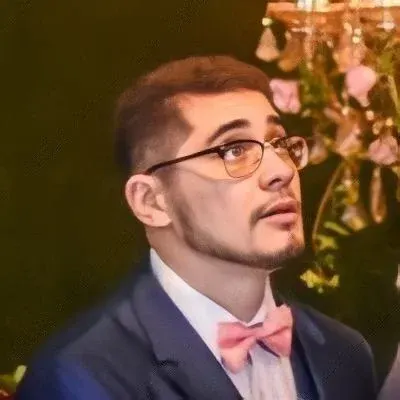
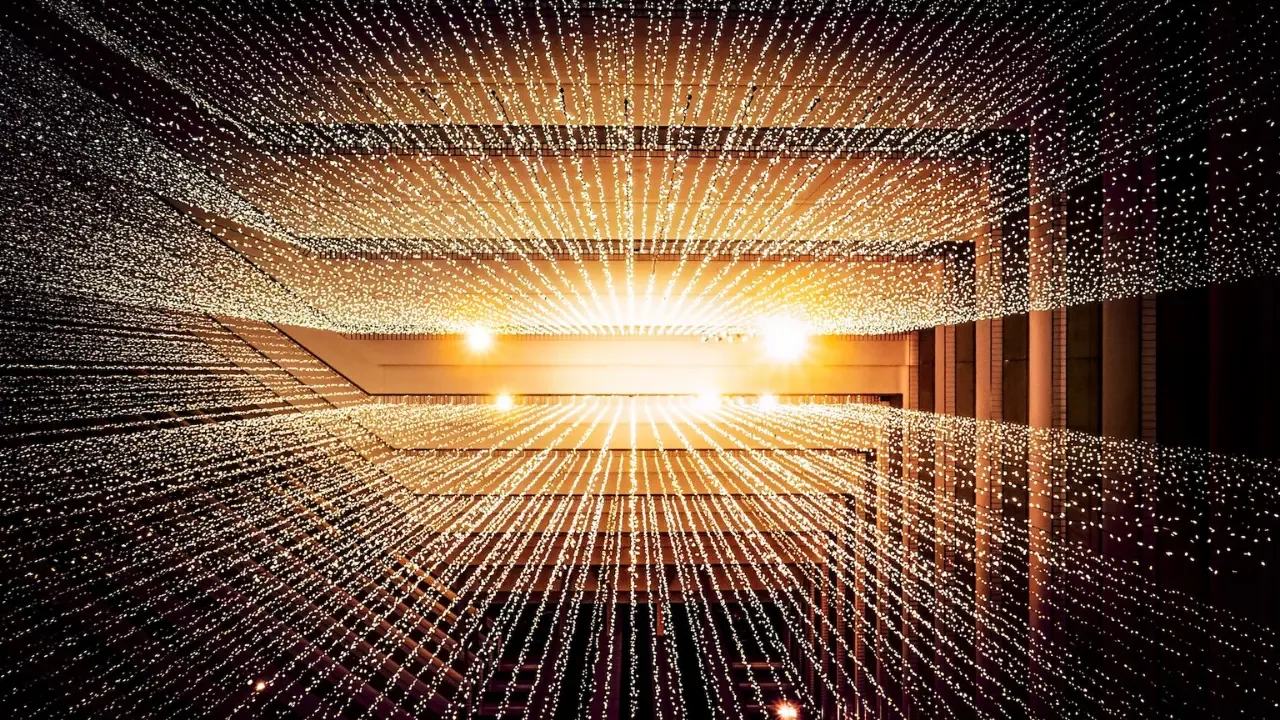
🔐 The Dangers of Using async/await in a forEach Loop 🔐
So, you want to use async/await
in a forEach
loop to loop through an array of files and await the contents of each file. It seems like a straightforward task, but beware! There are potential pitfalls to using async/await
in higher-order functions like forEach
. Let's dive into the common issues and easy solutions.
🔎 The Problem 🔎
Before we begin, let's understand the context. In the given code snippet, we have a function printFiles
that tries to print the contents of each file. It uses the fs-promise
library for file operations and await
to handle asynchronous calls.
💥 The Potential Issue 💥
The issue with this code arises from the fact that the forEach
loop doesn't respect the async/await
keywords. The loop doesn't pause for each await
statement to complete before moving on to the next iteration. Instead, it immediately continues execution, potentially causing unintended consequences.
🚩 The Consequence 🚩
In our code example, if there are multiple files, the forEach
loop will fire off multiple await
calls simultaneously. This results in a race condition, where the order of execution and console output becomes unpredictable. Yikes!
💡 The Solution 💡
To prevent this problem, we need to find an alternative approach. One way is to use a for...of
loop instead of forEach
. In this case, the for...of
loop respects the await
keyword and waits for each iteration to complete before moving on to the next one.
for (const file of files) {
const contents = await fs.readFile(file, 'utf8')
console.log(contents)
}
Now, the code executes as intended, ensuring that each file is processed before moving on to the next one.
💪 Take It Up a Notch 💪
If you're feeling adventurous, you can further optimize this code by using Promise.all
. This allows us to fire off multiple fs.readFile
calls concurrently while still maintaining order.
const filePromises = files.map(file => fs.readFile(file, 'utf8'))
const contentsArray = await Promise.all(filePromises)
for (const contents of contentsArray) {
console.log(contents)
}
With this approach, we can speed up the execution time when there are multiple files by utilizing the power of concurrent processing.
📣 The Call to Action 📣
Now that you understand the potential dangers and have learned the easy solutions, it's time to put your newfound knowledge to the test! Go ahead and try implementing the for...of
loop or the optimized version using Promise.all
. Share your experience and any other tips you might have in the comments below. Let's solve this async dilemma together! 💪💡
Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
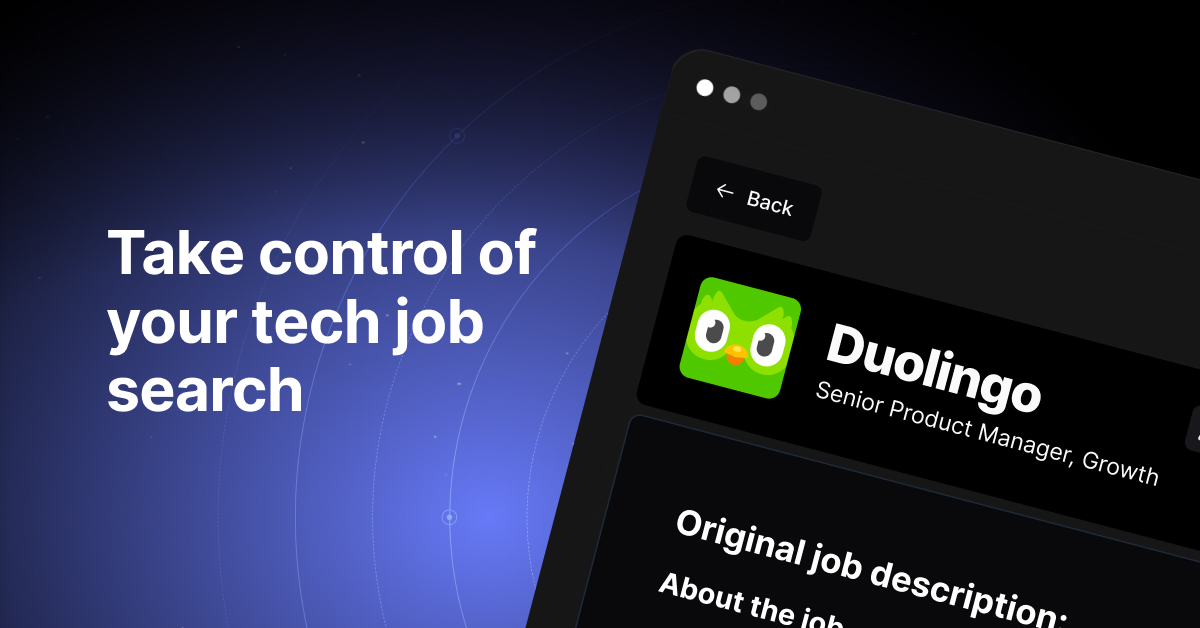