Use basic authentication with jQuery and Ajax
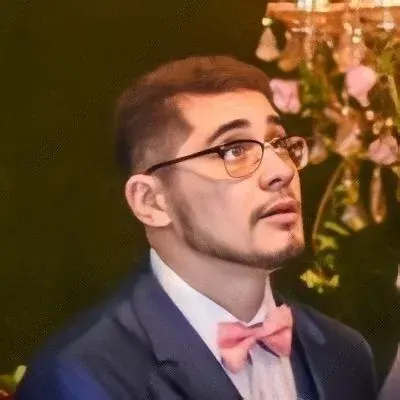
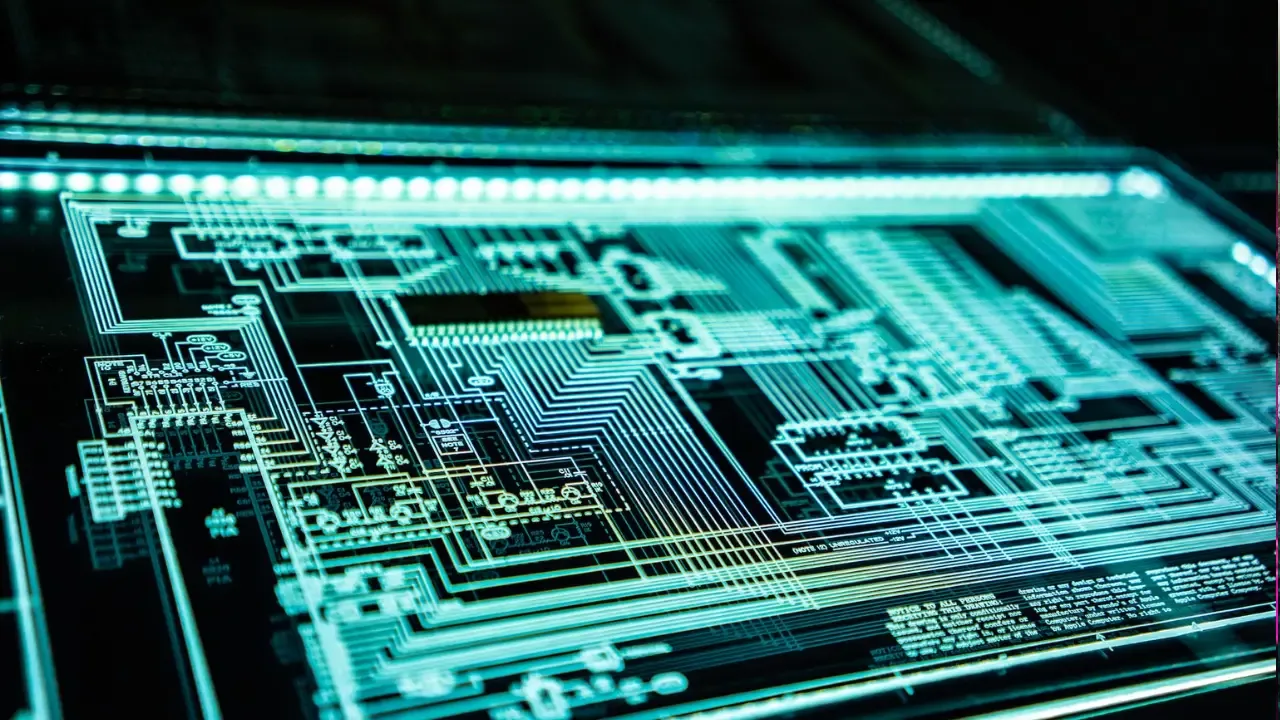
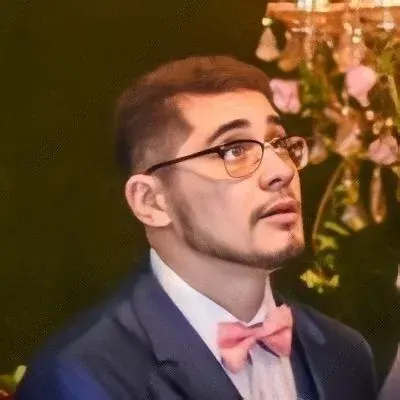
🤔 How to Use Basic Authentication with jQuery and Ajax?
Are you looking to implement basic authentication through your browser, but having trouble getting it to work? Don't worry, I've got you covered! In this blog post, we'll address the common issues and provide easy solutions for using basic authentication with jQuery and Ajax.
But first, let's understand the problem at hand.
The Problem
In basic authentication, the browser prompts the user to enter their credentials (username and password) to access a protected resource. However, you might want to handle this authentication programmatically instead of relying on the browser's default behavior. This is where jQuery and Ajax come into play.
The Solution
To handle basic authentication programmatically, you need to add the username and password directly to the URL you're requesting. Here's an example:
http://username:password@server.in.local/
Before we dive into the code, let's take a look at the HTML form and script provided to give you some context.
The HTML Form
<form name="cookieform" id="login" method="post">
<input type="text" name="username" id="username" class="text"/>
<input type="password" name="password" id="password" class="text"/>
<input type="submit" name="sub" value="Submit" class="page"/>
</form>
The Script
var username = $("input#username").val();
var password = $("input#password").val();
function make_base_auth(user, password) {
var tok = user + ':' + password;
var hash = Base64.encode(tok);
return "Basic " + hash;
}
$.ajax({
type: "GET",
url: "index1.php",
dataType: 'json',
async: false,
data: '{"username": "' + username + '", "password" : "' + password + '"}',
success: function (){
alert('Thanks for your comment!');
}
});
Now, let's break down the code for a better understanding.
The variables
username
andpassword
capture the values entered in the form.The
make_base_auth
function encodes the username and password using the Base64 encoding method. This encoded value is then used to generate the authentication header.The
$.ajax
function makes an Ajax request to the server with the following configuration:Type: GET (You can change it to POST if required)
URL: Specifies the endpoint where you want to send the request.
DataType: JSON (Change it according to your requirement)
Async: False (Change it if you want an asynchronous request)
Data: Passes the username and password as data in the request.
Success: A callback function that executes if the request is successful.
Putting it All Together
To use basic authentication with jQuery and Ajax, you can modify the provided script like this:
var username = $("input#username").val();
var password = $("input#password").val();
$.ajax({
type: "GET",
url: "http://" + username + ":" + password + "@server.in.local/",
dataType: 'json',
async: false,
success: function (){
alert('Thanks for your comment!');
}
});
That's it! Now you can make the authentication through the script without relying on the browser's default behavior.
Wrapping Up
Implementing basic authentication with jQuery and Ajax might seem daunting at first, but with a little bit of code and understanding, you can make it work seamlessly. Remember to modify the URL with your credentials and endpoint, and you'll be good to go!
So why wait? Give it a try and let us know how it goes in the comments below! We'd love to hear about your experiences. 😊