Use async await with Array.map
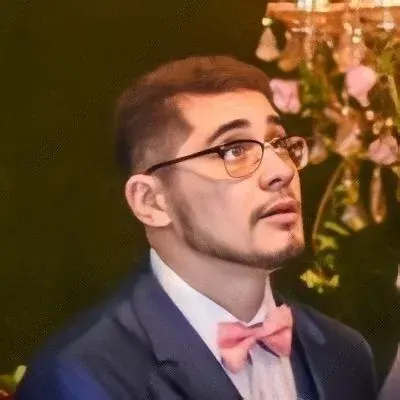
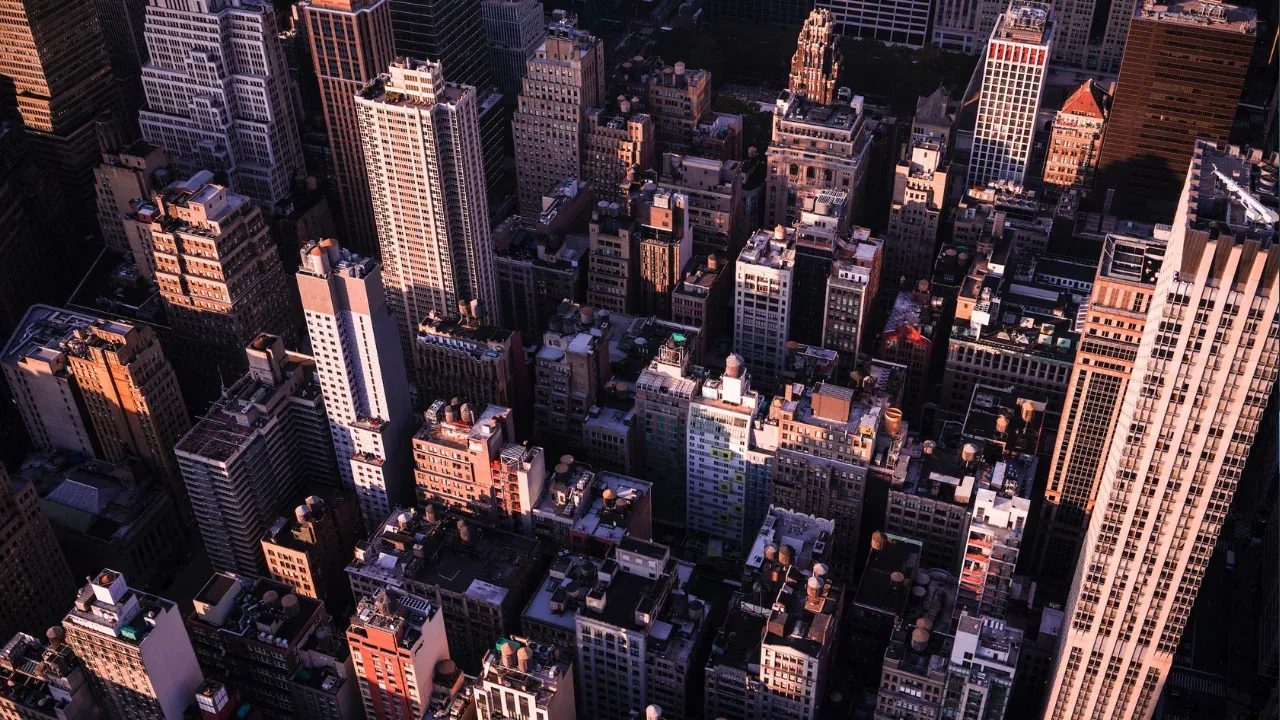
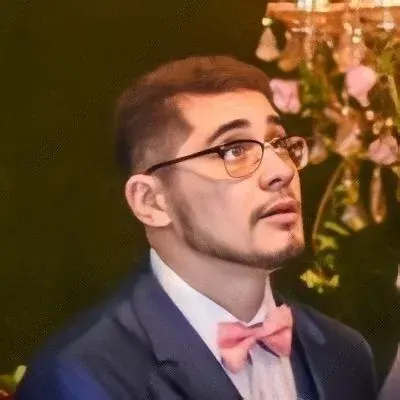
Using async/await with Array.map: A Complete Guide 😎✨
Do you find yourself scratching your head and wondering why async/await
and Array.map
don't seem to play nicely together? Don't worry, you're not alone! Many developers, especially those new to asynchronous programming, have faced this common issue. In this blog post, we'll tackle this problem head-on and provide you with easy solutions to make async/await
and Array.map
work together harmoniously. Let's dive in! 🚀
Understanding the Error
Before we jump into the solutions, let's first understand the error thrown by TypeScript in the given code snippet:
TS2322: Type 'Promise<number>[]' is not assignable to type 'number[]'.
Type 'Promise<number>' is not assignable to type 'number'.
This error is telling us that the type of the result returned by the arr.map
function is not compatible with the expected type (number[]
). TypeScript is warning us that we're trying to assign a Promise<number>[]
to a number[]
. But fear not, we have a fix for that! 😄
Solution 1: Using Array.prototype.map with Promise.all
One way to solve this issue is by combining the power of async/await
, Array.prototype.map
, and Promise.all
. Let's take a look at the modified code snippet:
var arr = [1, 2, 3, 4, 5];
var results = await Promise.all(arr.map(async (item) => {
await callAsynchronousOperation(item);
return item + 1;
}));
By wrapping the arr.map
function with Promise.all
, we can await the resolution of all the promises returned by the map
operation. This ensures that the await
only proceeds once all asynchronous operations have completed. The result is an array of the transformed values, ready to be assigned to the results
variable.
Solution 2: Parallel Execution using Promise.allSettled
If you prefer to process the array elements asynchronously in parallel, regardless of whether some of the promises get rejected, you can use Promise.allSettled
instead. Here's how you can modify the code:
var arr = [1, 2, 3, 4, 5];
var promises = arr.map(async (item) => {
await callAsynchronousOperation(item);
return item + 1;
});
var settledPromises = await Promise.allSettled(promises);
var results = settledPromises
.filter((promise) => promise.status === "fulfilled")
.map((promise) => (promise as PromiseFulfilledResult<number>).value);
In this solution, we make use of Promise.allSettled
to parallelize the execution of the promises returned by arr.map
. After awaiting the settled promises, we filter out any rejected promises using the status
property and then extract the fulfilled values using the value
property. Voila! 🎉
Call-to-Action: Share Your Experience
We hope this guide has helped you overcome the challenges of using async/await
with Array.map
. Now it's your turn to take action! Share your experience in the comments below. Have you encountered this issue before? Did these solutions work for you? Let's learn from each other and make the coding world a better place! 🌟💬
Remember to subscribe to our newsletter for more exciting tech tips and tricks. Happy coding! 👩💻👨💻
Disclaimer: The code examples in this blog post are written in JavaScript using TypeScript syntax.