URL Encode a string in jQuery for an AJAX request
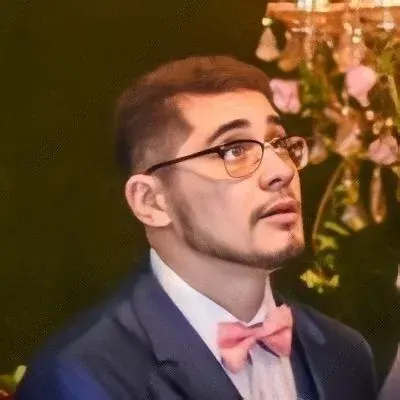
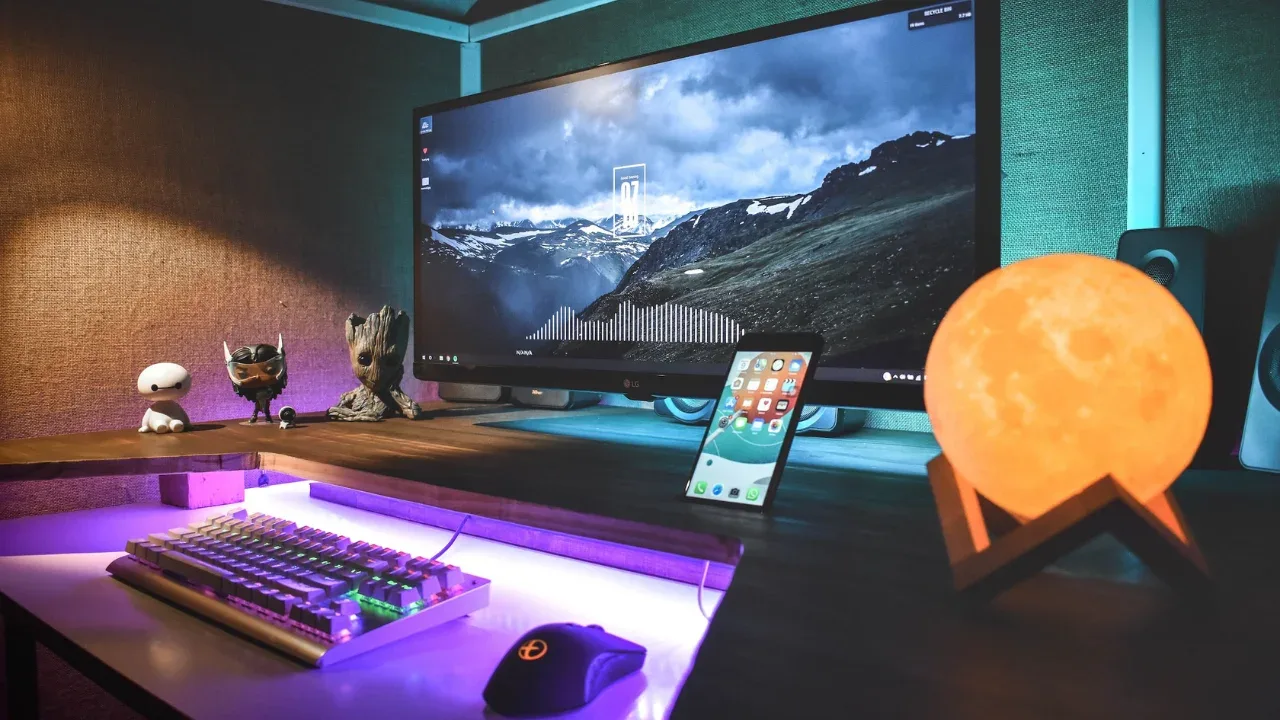
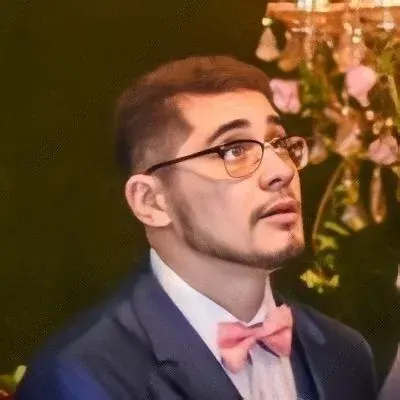
📝 Blog Post: URL Encode a string in jQuery for an AJAX request
Are you working on implementing Google's Instant Search in your application? Are you facing an issue where spaces in between first and last names are not being encoded as a "+" sign, thus breaking the search? If so, then you're in the right place! In this blog post, we'll discuss how to safely URL encode a string in jQuery for an AJAX request, so your search functionality works flawlessly. 🚀✨
Understanding the Issue
Let's first understand the problem at hand. When the user types in the text input, you want to fire off HTTP requests. However, the space between first and last names is not being encoded as a "+" sign. This can lead to incorrect search results and a broken search experience. 😬
Solution 1: Replacing Spaces with "+"
One way to solve this issue is by replacing the spaces with a "+" sign. jQuery provides a built-in method called encodeURIComponent()
that can URL encode a string. We can leverage this method to replace spaces with "+" signs in your AJAX request URL. 🔄
Here's an updated version of your code with the necessary changes:
$("#search").keypress(function() {
var query = "{% url accounts.views.instasearch %}?q=" + encodeURIComponent($('#tags').val().replace(/\s/g, '+'));
var options = {};
$("#results").html(ajax_load).load(query);
});
In the code above, we're using the replace()
method with a regular expression (/\s/g
) to match all occurrences of spaces. We then replace each space with a "+". Finally, we pass the modified string to encodeURIComponent()
to URL encode it correctly for the AJAX request.
Solution 2: Safely URL Encoding the String
Alternatively, you can directly URL encode the entire string, including spaces, using the encodeURIComponent()
method. This approach ensures that all special characters are encoded correctly, not just spaces. 🌟
Here's an updated version of your code using this approach:
$("#search").keypress(function() {
var query = "{% url accounts.views.instasearch %}?q=" + encodeURIComponent($('#tags').val());
var options = {};
$("#results").html(ajax_load).load(query);
});
With this code, the entire user input will be properly URL encoded, ensuring a safe and reliable search functionality in your application. 🔒💡
Time to Enhance Your Search!
Now that you've learned how to URL encode a string in jQuery for an AJAX request, it's time to implement it in your application and enhance your search functionality! 🔍✅
Whether you choose to replace spaces with "+" signs or simply URL encode the entire string, these solutions will help you avoid broken searches and ensure accurate results for your users. 🎯🔎
If you found this blog post helpful, let us know in the comments below! We'd love to hear about your experience and any other related questions you may have. Happy coding! 💻😊
Note: Don't forget to handle the decoding of the URL on the server-side for a complete and secure implementation. 🛡️🔓
[INSERT CALL-TO-ACTION HERE]
[INSERT RELATED ARTICLES/RESOURCES HERE]
[INSERT SOCIAL SHARING BUTTONS HERE]