Uploading both data and files in one form using Ajax?
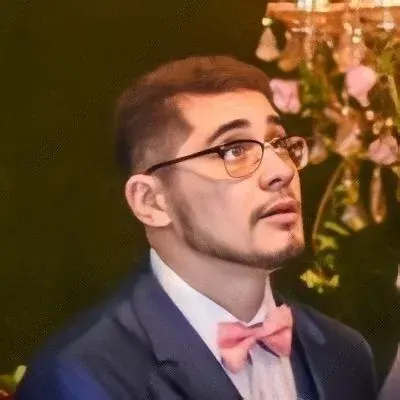
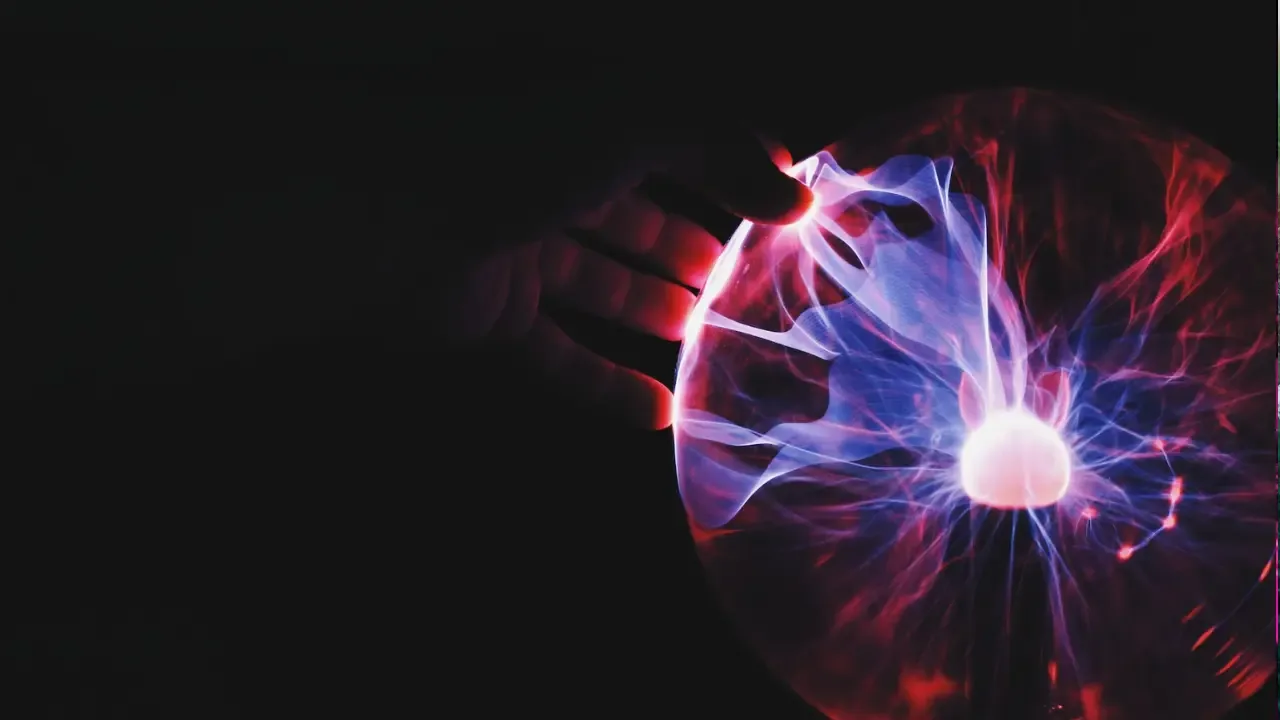
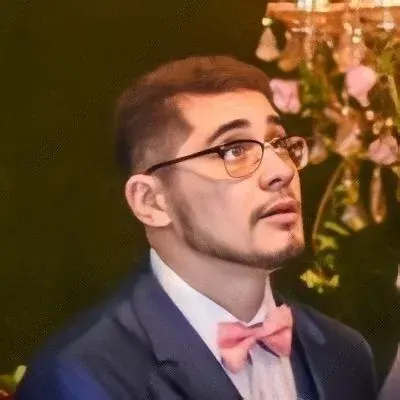
Uploading both data and files in one form using Ajax? 📁💻
Are you struggling with uploading both data and files in one form using Ajax? 🤔 Don't worry, I've got you covered! In this guide, I'll walk you through the common issues you might encounter and provide easy solutions to help you overcome them. 💪
The Challenge: Combining Data and Files in One Form via Ajax
So, you're using jQuery and Ajax to submit your forms, but you're not sure how to send both data and files in one form. 😕 Let's first take a look at your current approach:
<form id="data" method="post">
<input type="text" name="first" value="Bob" />
<input type="text" name="middle" value="James" />
<input type="text" name="last" value="Smith" />
<button>Submit</button>
</form>
<form id="files" method="post" enctype="multipart/form-data">
<input name="image" type="file" />
<button>Submit</button>
</form>
And your corresponding jQuery Ajax code:
$("form#data").submit(function(){
var formData = $(this).serialize();
// Ajax code here...
return false;
});
$("form#files").submit(function(){
var formData = new FormData($(this)[0]);
// Ajax code here...
return false;
});
Now you're wondering if it's possible to combine these methods and upload both data and files in one form via Ajax. 🤷♀️
The Solution: Combining Data and Files in One Form with Ajax
Yes, it is possible to achieve this! 🙌 Here's how you can modify your code to send data and files in one form using Ajax:
<form id="datafiles" method="post" enctype="multipart/form-data">
<input type="text" name="first" value="Bob" />
<input type="text" name="middle" value="James" />
<input type="text" name="last" value="Smith" />
<input name="image" type="file" />
<button>Submit</button>
</form>
And your updated jQuery Ajax code:
$("form#datafiles").submit(function(){
var formData = new FormData($(this)[0]);
// Ajax code here...
return false;
});
By combining both sets of form fields into one form with the id
attribute set to "datafiles", you can now send all the data and files in one Ajax request. 📥🚀
Conclusion: You're Ready to Go!
You've learned how to upload both data and files in one form using Ajax. 💥 With the modified code, you can now effortlessly send all the form's data and files via Ajax in a single request. 🙌
Try implementing this solution and let me know how it goes for you! If you have any questions or face any issues, don't hesitate to leave a comment below. Happy coding! 😊👩💻