Understanding unique keys for array children in React.js
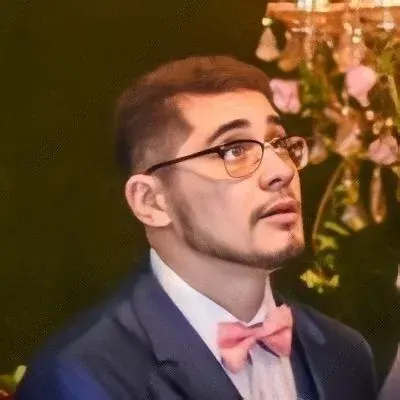
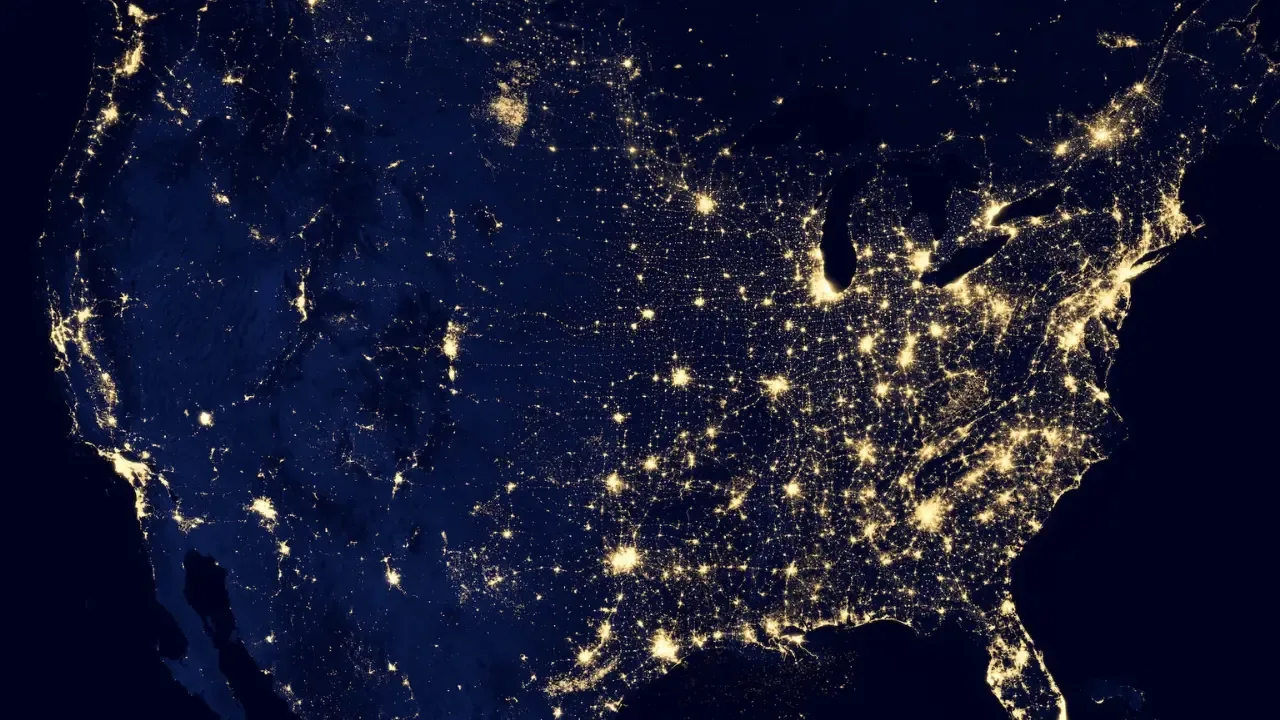
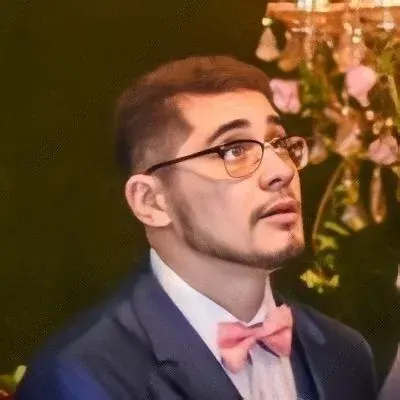
Understanding Unique Keys for Array Children in React.js
If you've encountered the error message "Each child in an array should have a unique 'key' prop" while working with React.js, don't worry - you're not alone. This error occurs when React is unable to differentiate between the items in an array, which can cause unexpected behavior or decrease performance. In this blog post, we'll explore what causes this error and provide easy solutions to address it.
The Problem
Let's start by understanding why this error occurs. In React, when rendering an array of components, each component needs a unique "key" prop. This prop helps React recognize each component and efficiently update the virtual DOM. Without a unique key, React may not properly reconcile the component hierarchy, leading to issues like incorrect rendering or updates.
In the provided context, the error message mentions that each child in the array should have a unique key prop. This means that both the <TableRowItem>
and <TableHeader>
components within the array should have unique keys.
The Solution
To fix the unique key prop error, you can follow these steps:
Assign a unique key prop to each child component in the array: In the provided code snippet, the
<TableRowItem>
component is built from an array calledrows
. To ensure eachTableRowItem
has a unique key, you should assign it a unique identifier. For example, you can use theid
property of theitem
passed to the component as the key:
<TableRowItem key={item.id} data={item} columns={columnNames} />
Ensure the parent component's key prop remains unchanged: In the code snippet, both the
<thead>
and<tbody>
components have akey
prop. However, these keys are static, and it's important to note that React's reconciliation algorithm relies on the keys changing when the underlying data changes. In most cases, you don't need to explicitly set keys for wrapper components like<thead>
and<tbody>
. Instead, use the key prop for the individual child components.
Once you've implemented these changes, the unique key prop error should no longer occur, and React will be able to efficiently manage the rendering and updating of array children.
Example Implementation
Let's take a look at an example implementation of the fixed code:
var TableComponent = React.createClass({
render: function() {
var { columnNames, rows } = this.props;
return (
<table>
<thead>
<TableHeader columns={columnNames} />
</thead>
<tbody>
{rows.map((item) => (
<TableRowItem key={item.id} data={item} columns={columnNames} />
))}
</tbody>
</table>
);
}
});
var TableRowItem = React.createClass({
render: function() {
var { data, columns } = this.props;
return (
<tr>
{columns.map((c) => (
<td key={data[c]}>{data[c]}</td>
))}
</tr>
);
}
});
In this implementation, we've used the rows.map()
function to iterate over the rows
array and create a unique TableRowItem
for each item. The key
prop is set to the id
property of each item
, ensuring uniqueness.
Conclusion
Understanding unique keys for array children in React.js is crucial to avoid the "Each child in an array should have a unique 'key' prop" error. By assigning unique keys to each child component within an array, you enable React to efficiently update the virtual DOM and provide a smooth user experience. Remember to assign unique keys to child components and avoid using static keys for wrapper components.
If you've encountered this error, we hope this guide has helped you understand the issue and provided easy solutions to address it. Now go ahead and debug those React components with confidence! 🚀
Have you encountered other React.js errors or have any tips to share? Let us know in the comments below! Together, we can level up our React skills. 💪