Uncaught Error: Invariant Violation: Element type is invalid: expected a string (for built-in components) or a class/function but got: object
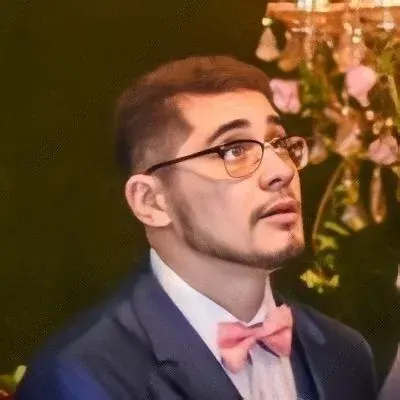
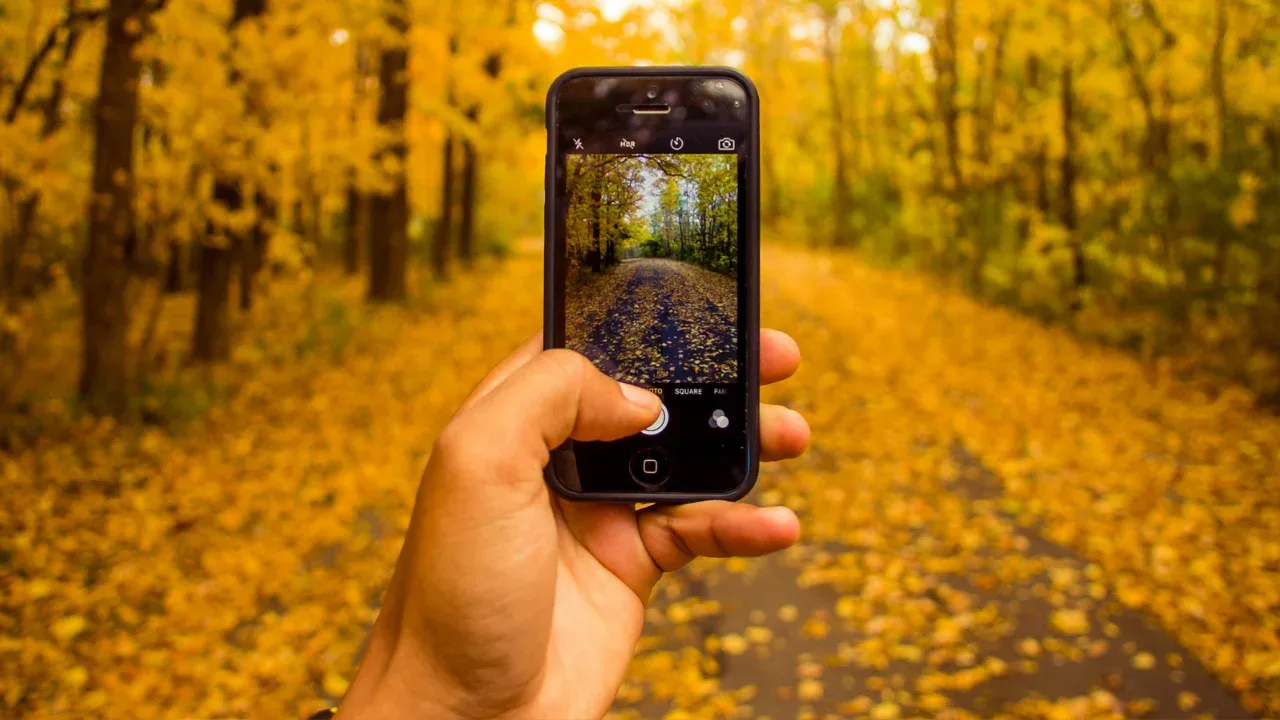
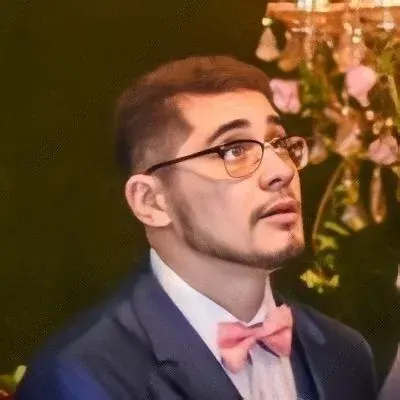
🚨 Fixing the "Uncaught Error: Invariant Violation" in React 🚨
So, you're trying to build an awesome React app, but suddenly, this annoying error pops up: Uncaught Error: Invariant Violation: Element type is invalid: expected a string (for built-in components) or a class/function but got: object.
😱
Don't worry, you're not alone! This error is quite common when working with React, especially when importing components or modules. In this blog post, we'll dive into the most common causes of this error and provide you with easy solutions to fix it. Let's get started! 💪
What causes this error?
The error message itself gives us a clue about what's causing the problem: "Element type is invalid." This means that React is expecting a certain type of element, either a string for built-in components like div
or span
, or a class/function for composite components.
When React encounters an unexpected object instead of a valid element, it throws this error. Now let's explore some possible causes and how to fix them.
1️⃣ Issue: Importing components incorrectly
In your code, you have imported the About
component from ./components/Home
:
var About = require('./components/Home')
But it seems like you have mistakenly imported the wrong component. This mismatch is causing the error. In the given code snippet, Home
is the appropriate component to import, not About
.
Solution:
Update your code to import the correct component:
var Home = require('./components/Home')
2️⃣ Issue: Incorrect usage of imported components
In your Home.jsx
file, you are using the RaisedButton
component provided by the material-ui
library incorrectly.
var RaisedButton = require('material-ui/lib/raised-button');
// ...
<RaisedButton label="Default" />
The RaisedButton
component needs to be used with JSX syntax, but you are using it without the corresponding JSX syntax.
Solution:
Use the import
statement to import the RaisedButton
component correctly:
import RaisedButton from 'material-ui/RaisedButton';
// ...
<RaisedButton label="Default" />
🎉 Problem solved! 🎉
Now that we've addressed the common causes of the "Uncaught Error: Invariant Violation" in React, you should be able to run your app without any issues. By fixing these small mistakes, you'll be back on track to building your awesome React application. 🙌
If you come across any other issues or have any questions, let us know in the comments below. We'd love to help you out! 😊
Happy coding! 💻✨