Typescript: React event types
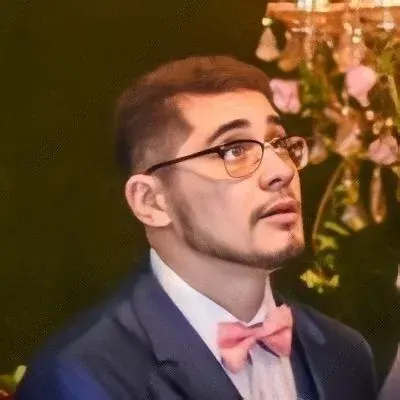
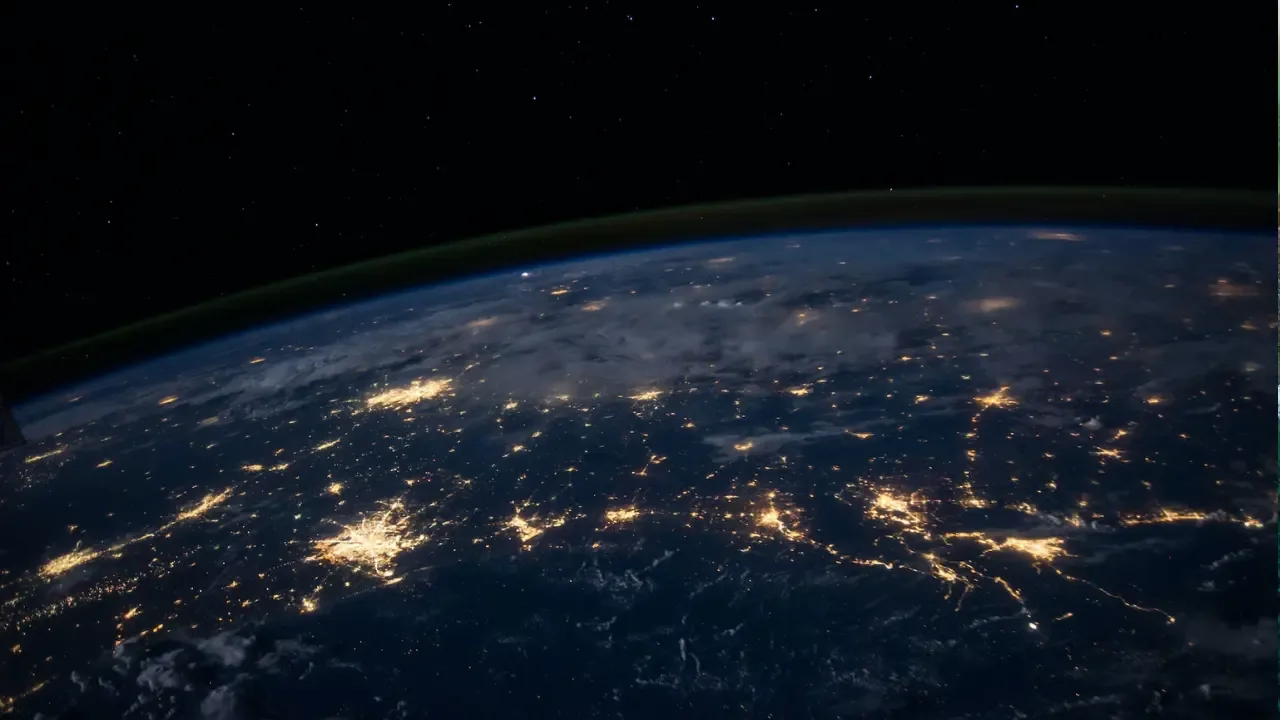
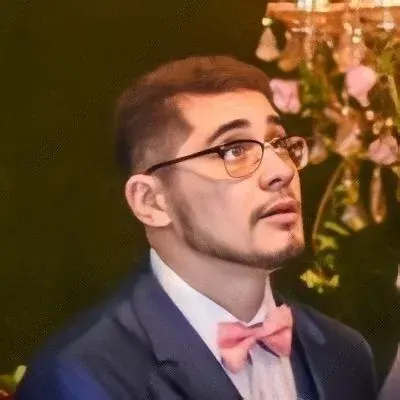
React Event Types in Typescript: Solving Common Issues 👨💻💻
Are you struggling to find the correct type for React events in Typescript? 🤔 Don't worry, you're not alone! Many developers face this challenge when trying to clean up their code and eliminate the use of the any
type. In this blog post, we'll explore some common issues and provide easy solutions to help you tackle this problem head-on. Let's dive in! 🚀
The Initial Approach: Using any
for Simplicity 🤷♂️
When we first start working with React events in Typescript, it's tempting to use the any
type. After all, it allows us to bypass type checking and quickly move forward with our development process. However, relying on any
can lead to potential issues down the line, such as hidden bugs and decreased code maintainability. It's always better to have strong typing in our codebase.
The Problem: Type Errors with React.SyntheticEvent<EventTarget>
❌
In the provided code snippet, the author used the type React.SyntheticEvent<EventTarget>
for the event type. However, this approach resulted in an error stating that name
and value
do not exist on target
. This is because EventTarget
doesn't include these properties by default.
The Solution: Using More Specific Event Types 🙌✅
To solve this problem, we need to use more specific event types. Instead of React.SyntheticEvent<EventTarget>
, we can utilize the ChangeEvent
type for input events and the FormEvent
type for form submission events. Let's update the code to reflect these changes:
update = (e: ChangeEvent<HTMLInputElement>): void => {
this.props.login[e.target.name] = e.target.value;
};
submit = (e: FormEvent<HTMLFormElement>): void => {
this.props.login.logIn();
e.preventDefault();
};
By using the appropriate event types, we can access the name
and value
properties directly from the e.target
object without any type errors. This approach improves the type safety of our code and makes it easier to catch potential mistakes during development.
A Generalized Approach: Leveraging the EventTarget
Type 🌟
If you're looking for a more generalized solution that can be applied to various event types, you can make use of TypeScript's type assertions. Here's an example:
update = (e: React.SyntheticEvent<EventTarget>): void => {
const target = e.target as HTMLInputElement;
this.props.login[target.name] = target.value;
};
By asserting e.target
as an HTMLInputElement
, we can access the name
and value
properties without any issues. This approach allows you to handle different event types within a single event handler method.
Your Turn to Take Action! 🚀💪
Now that you've learned how to correctly type React events in Typescript, it's time to apply this knowledge to your own projects. Start by identifying any occurrences of the any
type in your code and replace them with the appropriate event types. Don't forget to update your event handlers accordingly!
Remember, strong typing not only improves the reliability and maintainability of your code, but it also helps prevent bugs and enhances collaboration with other developers.
If you found this blog post helpful, consider sharing it with your fellow developers who might be facing similar challenges. Let's spread the knowledge and empower our community! 🌎🤝
Happy coding! 😄👩💻👨💻