TypeScript, Looping through a dictionary
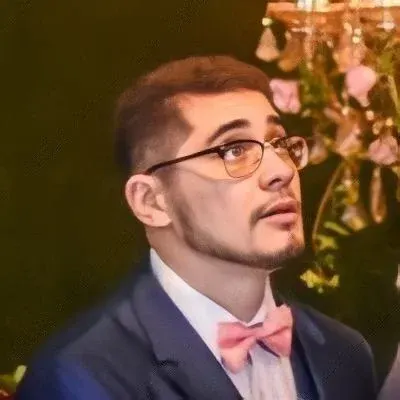

🚀 TypeScript Dictionary Looping: Easy Solutions for Your Code 🚀
Hey there, tech enthusiasts! 👋 Are you facing challenges with TypeScript's dictionaries? Don't worry, you're not alone! 🤔 Many developers have struggled with looping through dictionary keys or values when the dictionary is string indexed. But fear not! I'm here to provide you with some easy solutions. 💡
Understanding the Problem
As mentioned in the context, you have a dictionary in TypeScript with string indexing. Here's an example code snippet to visualize it:
myDictionary: { [index: string]: any; } = {};
The goal is to loop through the keys in this dictionary, or maybe you're interested in the values as well. Let's explore the possible solutions one by one. 🕵️♂️
Solution 1: Using Object.keys()
The Object.keys()
method in TypeScript provides an easy way to extract the keys from an object. We can leverage this method to loop through the dictionary keys. Here's an example:
for (const key of Object.keys(myDictionary)) {
console.log(key);
}
In this solution, we use a for...of
loop to iterate over each key returned by Object.keys()
. Feel free to replace console.log(key)
with any other logic you want to perform with the keys.
Solution 2: Utilizing for...in
Loop
The for...in
loop can also be used to traverse through the dictionary keys. Here's an example:
for (const key in myDictionary) {
if (myDictionary.hasOwnProperty(key)) {
console.log(key);
}
}
Using for...in
, we iterate over each key in the dictionary. Since for...in
also iterates over inherited properties, we add a check to ensure that the key belongs to the object itself, using hasOwnProperty()
method.
Engage with the Community! 🌟
Now that you have discovered two solutions to loop through TypeScript dictionaries, why not share your thoughts and experiences? Have you encountered any other challenges with TypeScript dictionaries? Let's discuss it in the comments below! 💬
Don't hesitate to ask questions or provide your own solutions. Let's grow together as a tech community! 👩💻👨💻
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
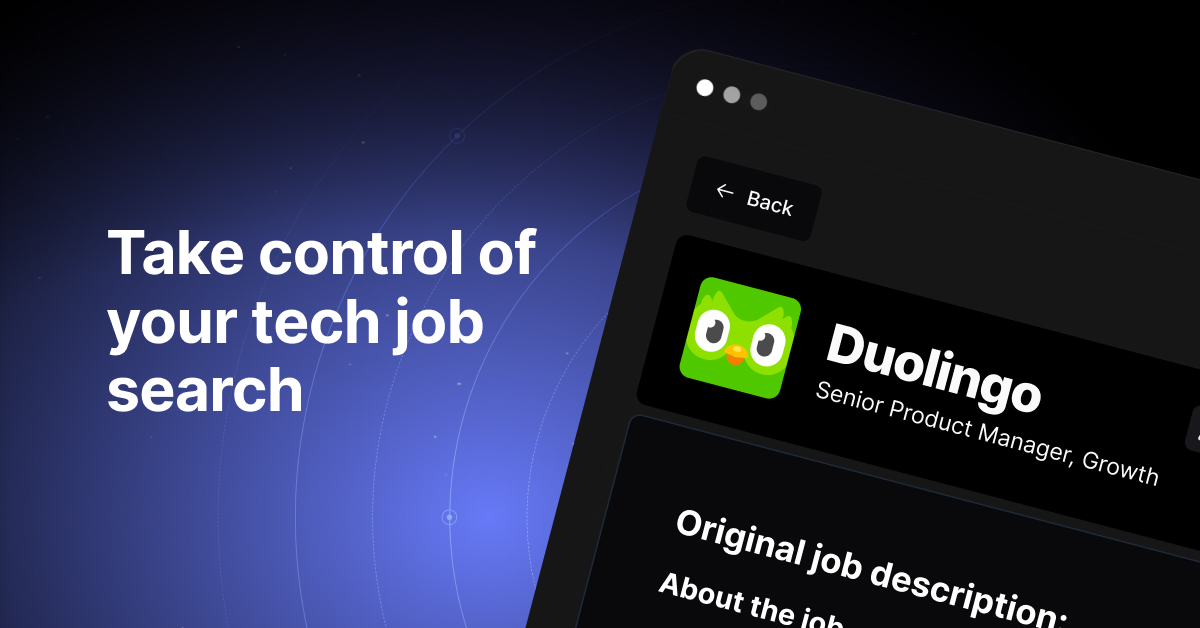