TypeScript export vs. default export
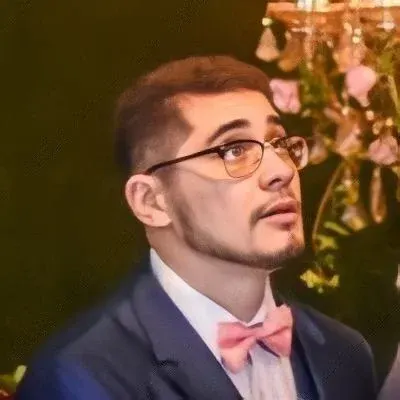
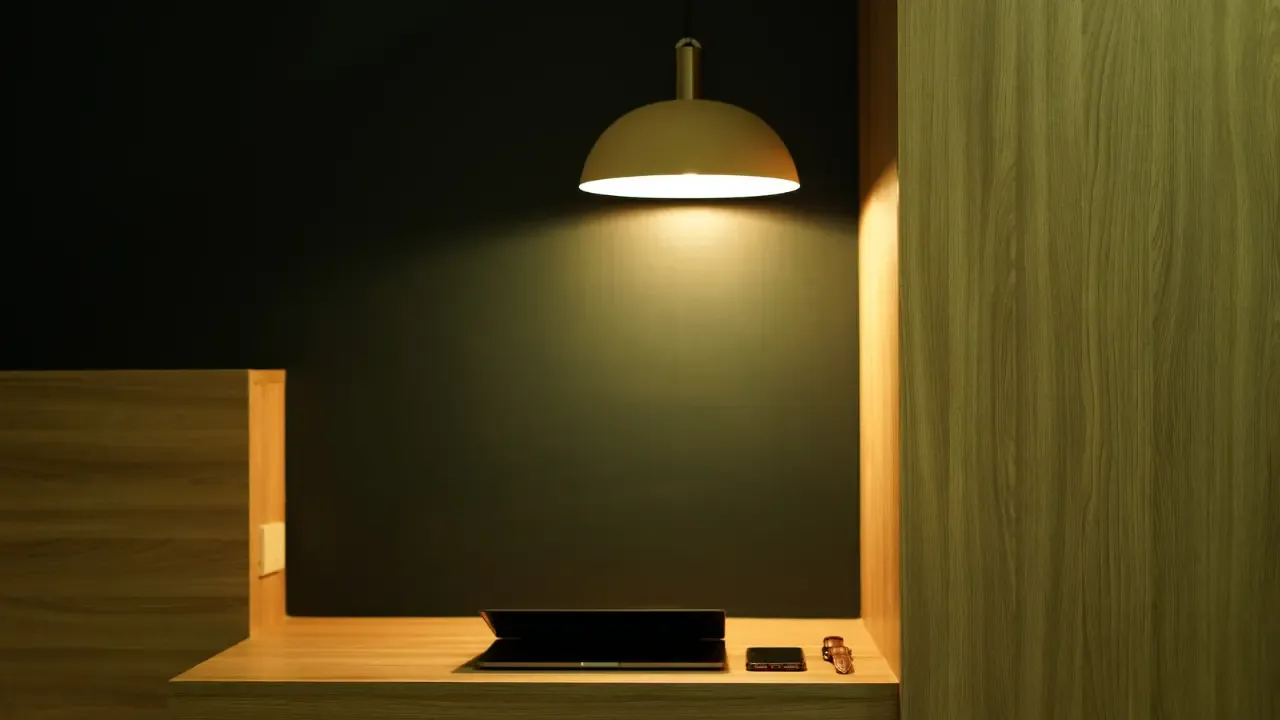
TypeScript Export vs. Default Export: Demystifying the Difference ✨
If you've been dabbling in TypeScript, you may have come across a puzzling dilemma: what is the difference between export
and default export
? 🤔 You've seen examples online where people export their classes using export
, but when you try to compile your code without adding the default
keyword, boom! It's an error party! 🚫😩
Don't fret! 🙅♀️ In this guide, we'll dive into the world of TypeScript exports, explain the difference between export
and default export
, address common issues, and provide easy solutions to get your code compiling. Plus, stick around until the end for an exciting call-to-action that will make you the TypeScript export pro! 👩💻🔥
🌟 Understanding the Difference
Let's start by clarifying the distinction between export
and default export
. The export
keyword is used to make a module's members available outside of the module, while default export
allows you to export a single value from a module.
When you use export
without the default
keyword, you are explicitly exporting that specific member. For example, take a look at this code snippet:
export class MyClass {
collection = [1,2,3];
}
By using export
, we make the MyClass
available for import in other modules. You can import it like this:
import { MyClass } from './path/to/MyClass';
On the other hand, default export
is used when you want to set a default value for a module. Here's an example:
export default class MyClass {
collection = [1,2,3];
}
With default export
, you can import the module's default value without needing to specify its name:
import MyClass from './path/to/MyClass';
🐛 Common Issues and Easy Solutions
Now, let's address the common issue you encountered: the error message Module has no default export
when you try to compile code without using default export
.
The error occurs because some TypeScript configurations (typically in a bundler like Webpack or a module loader like SystemJS) expect a default export by default. If your configuration is set up this way, you'll need to use default export
for your classes to compile successfully.
However, keep in mind that not all TypeScript projects require the use of default export
. If you're working on a smaller project or using a different configuration, you might not encounter this error at all.
If you want to avoid the error message, you have two options:
Update your configuration to accommodate non-default exports. This involves modifying your bundler or module loader configuration to support named exports instead of just default exports. This way, you can continue using
export
without thedefault
keyword.Embrace the
default export
and adjust your code accordingly. If you choose this route, make sure to update your imports throughout the project to match thedefault export
syntax.
📢 Call-to-Action: Become a TypeScript Export Master!
Congratulations, you've made it to the end of this guide! You now have a solid understanding of the difference between export
and default export
in TypeScript. Exciting, right? 😃
Now, it's time to put your knowledge into practice and become a TypeScript export master! Share this blog post with fellow TypeScript enthusiasts, spark engaging discussions, and show off your newfound expertise. Together, let's conquer the TypeScript export challenge and build amazing projects! 💪🚀
Happy exporting! ✨📦
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
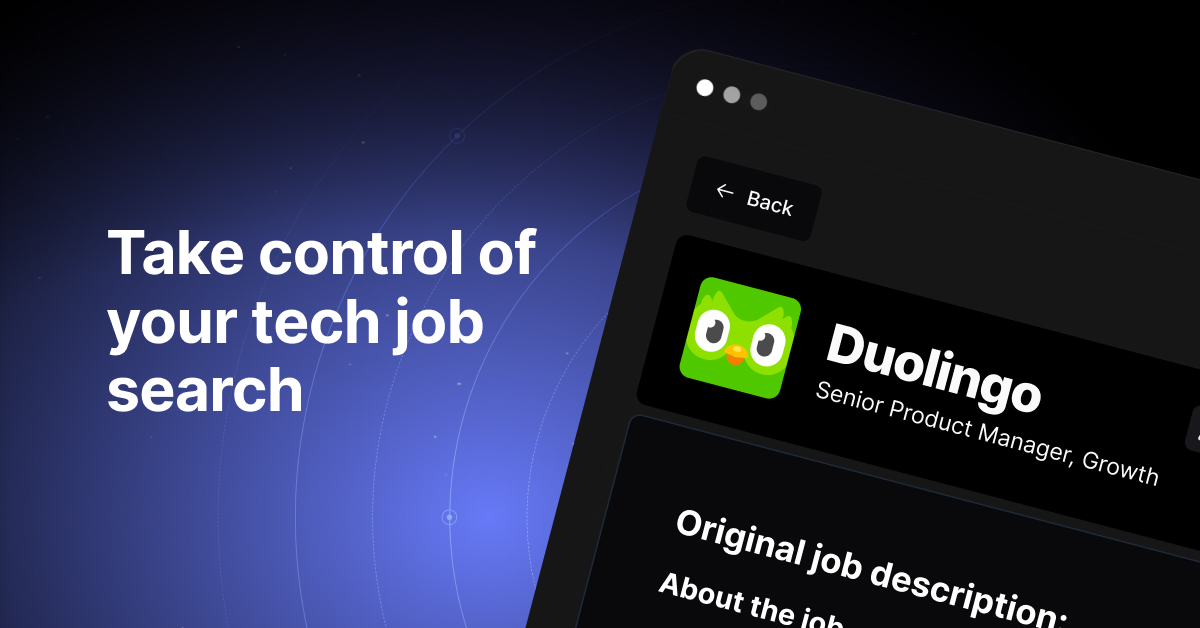