typescript - cloning object
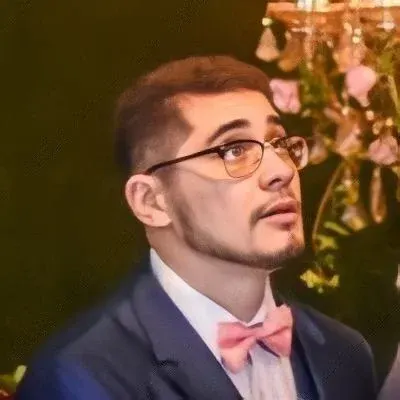
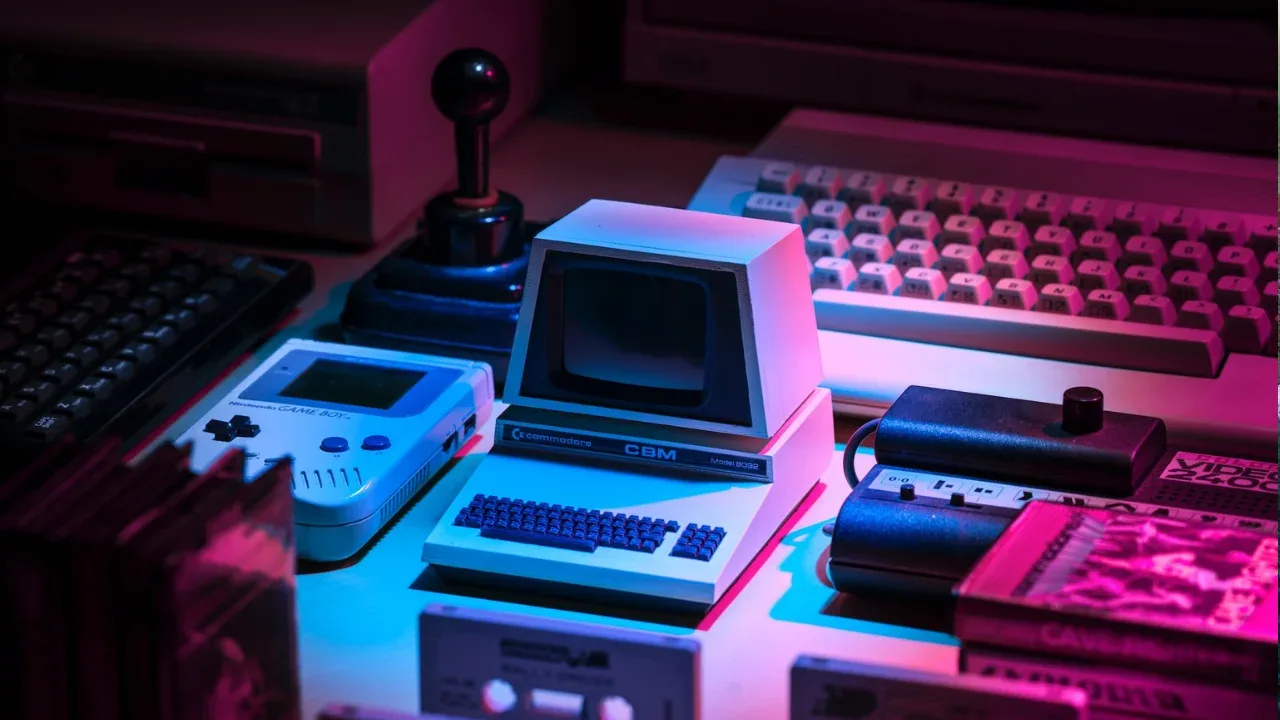
🚀📝 A Guide to Cloning Objects in TypeScript 🔄
Cloning objects with TypeScript can be tricky, especially when dealing with nested objects and inheritance. In this blog post, we'll address a common issue when trying to clone objects and provide easy solutions to help you overcome it. Let's get started! 🙌
The Problem: Cloning Objects in TypeScript
You have a superclass called Entity
, which serves as the parent for many subclasses, such as Customer
, Product
, and ProductCategory
. You want to clone an object that contains different subobjects dynamically. For example, you have a Customer
object that has multiple Product
objects, each of which has a ProductCategory
. 🧩
Here's an example of how your code might look:
var cust: Customer = new Customer();
cust.name = "someName";
cust.products.push(new Product(someId1));
cust.products.push(new Product(someId2));
To clone the entire object tree, you've created a clone
function in the Entity
class:
public clone(): any {
var cloneObj = new this.constructor();
for (var attribute in this) {
if (typeof this[attribute] === "object") {
cloneObj[attribute] = this.clone();
} else {
cloneObj[attribute] = this[attribute];
}
}
return cloneObj;
}
However, when transpiling to JavaScript, you encounter the following error: error TS2351: Cannot use 'new' with an expression whose type lacks a call or construct signature.
❌
The Solution: Fixing the Transpilation Error
Don't worry! Although the code works, we can refactor it to get rid of the transpiled error and make it even more robust. 👍
To fix the error, we can utilize TypeScript's Object.create
method, which creates a new object with the specified prototype object. Here's an updated version of the clone
function:
public clone(): any {
const cloneObj = Object.create(Object.getPrototypeOf(this));
for (const attribute in this) {
if (typeof this[attribute] === "object") {
cloneObj[attribute] = this[attribute].clone();
} else {
cloneObj[attribute] = this[attribute];
}
}
return cloneObj;
}
By using Object.create
, we create a new object with the same prototype as the original object. This ensures that the cloned object retains the same functionality and properties as the original object. When encountering nested objects, we call the clone
method recursively to handle their cloning. ♻️
Conclusion and Call-to-Action
And there you have it! You now know how to clone objects in TypeScript, even when dealing with nested structures. By utilizing Object.create
and a recursive clone
method, you can easily create copies of your objects without encountering transpilation errors. 🎉
Remember to always test your code thoroughly and adapt it to your specific project's needs. If you found this guide helpful, be sure to share it with your fellow TypeScript developers! 😊
Is there anything else you'd like to learn about TypeScript or any other programming languages? Let us know in the comments below! Let's keep the conversation going! 💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
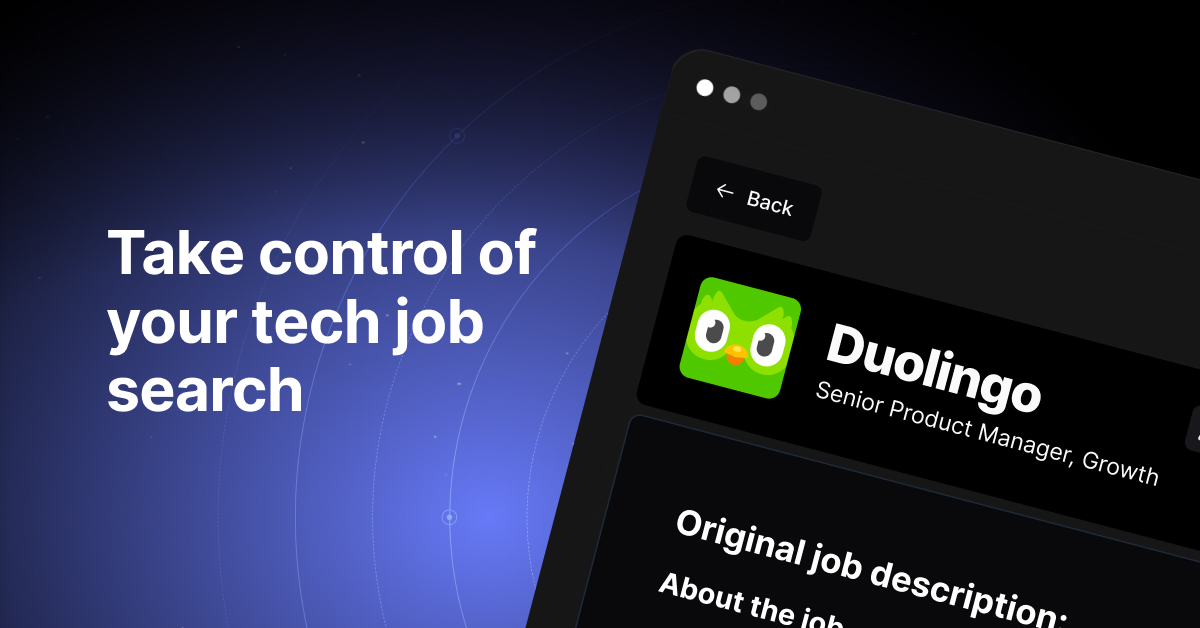