Trying to use the DOMParser with node js
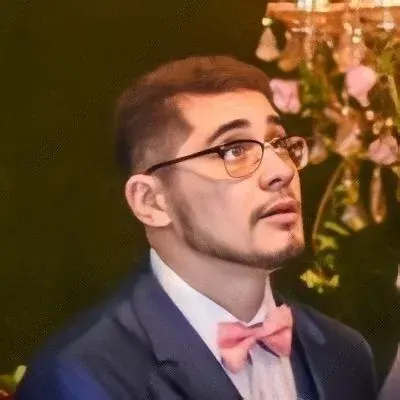
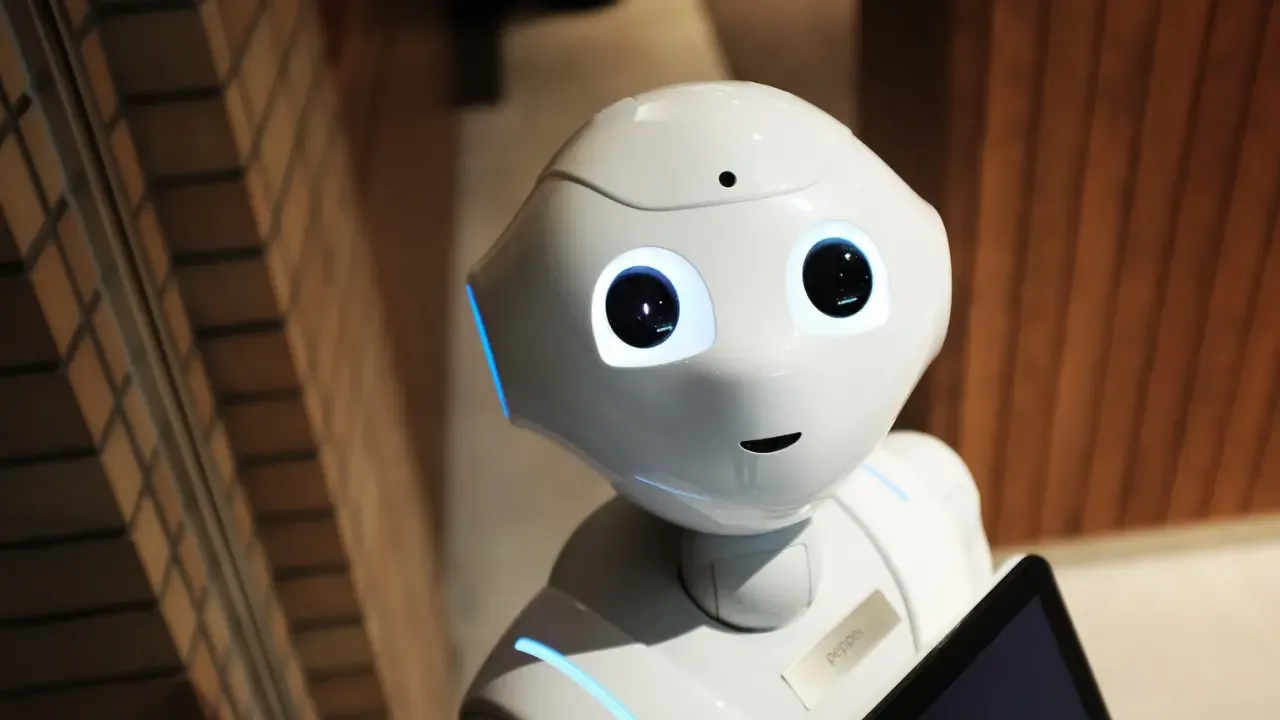
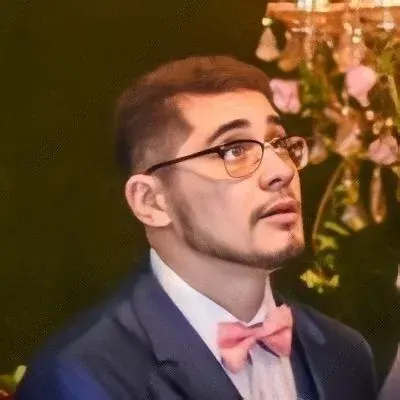
🔍 Understanding DOMParser in Node.js
Have you ever come across the error message "ReferenceError: DOMParser is not defined" when trying to use the DOMParser in your Node.js code? Don't worry - you're not alone! In this blog post, we'll dive into common issues with using the DOMParser in Node.js and explore easy solutions to help you overcome them.
🤷♂️ Why am I getting this error?
The error message you encountered occurs because the DOMParser is not a built-in module in Node.js like it is in browser environments. This means that the DOMParser is not readily available for you to use out of the box.
⚙️ Easy solution 1: Using a DOM implementation library
To use the DOMParser in Node.js, you'll need to install a DOM implementation library such as xmldom
. This library provides a DOM implementation that simulates the browser's DOM environment, including the DOMParser.
To install xmldom
, open your terminal and run the following command:
npm install xmldom
Once installed, you can import and use the DOMParser in your Node.js code like this:
const { DOMParser } = require('xmldom');
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlString, 'text/xml');
🙌 Easy solution 2: Using xml2js library
Another popular approach to parse XML into JSON format in Node.js is to use the xml2js
library. This library simplifies XML parsing by converting it directly into a JavaScript object.
To install xml2js
, run the following command in your terminal:
npm install xml2js
Here's an example of how to use xml2js
to convert XML to JSON:
const parser = require('xml2js').parseString;
parser(xmlString, (err, result) => {
if (err) {
console.error(err);
} else {
console.log(result);
}
});
📣 Join the conversation
We hope these easy solutions help you resolve the "DOMParser is not defined" error in your Node.js code. If you have any questions or other solutions you'd like to share, we'd love to hear from you!
💡 Have you encountered any other challenges with Node.js development? Let us know in the comments below!
✨ References:
Keep coding and happy parsing! 🚀✨