Trim string in JavaScript
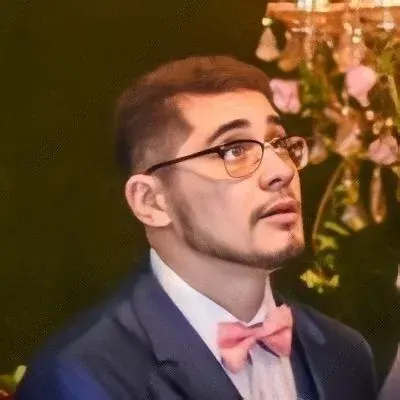
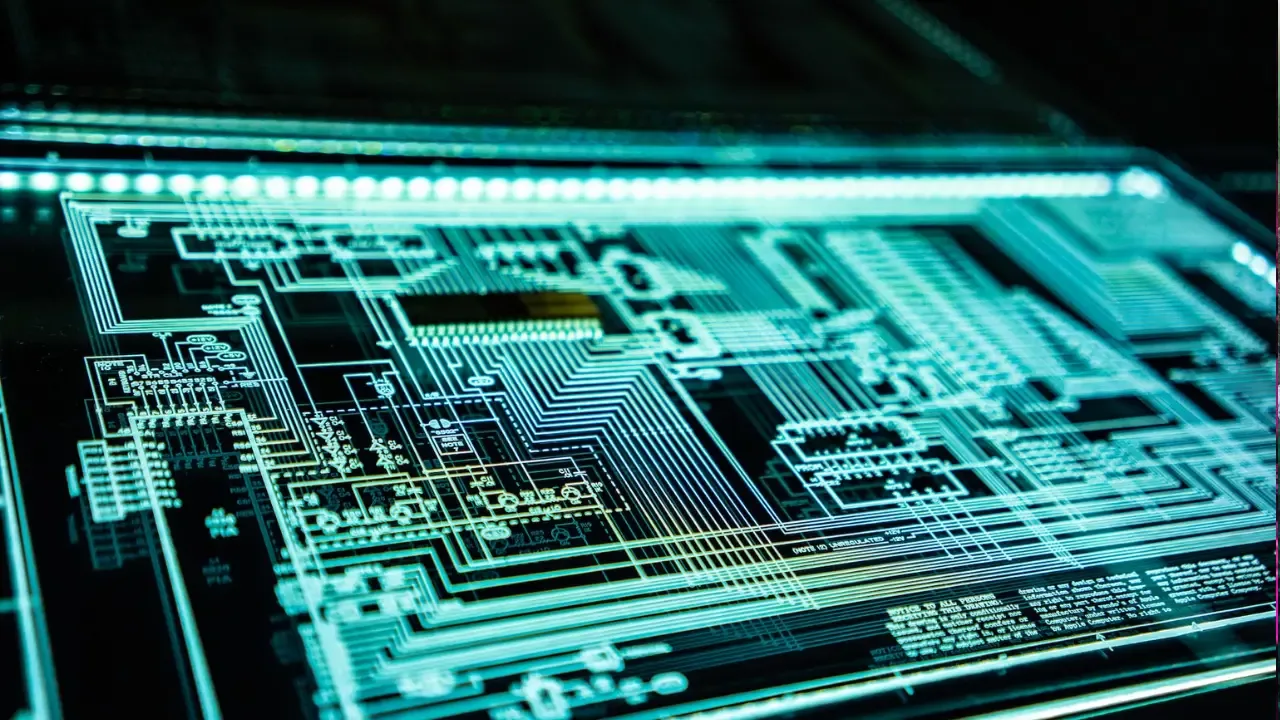
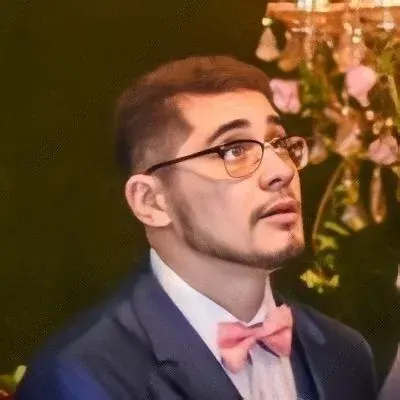
๐งต How to Trim a String in JavaScript: A Handy Guide ๐งต
We all know how frustrating it can be to deal with pesky whitespace at the start and end of a string. But fear not, fellow developers! In this blog post, we'll explore some common issues related to trimming strings in JavaScript and provide you with easy solutions to tackle this problem. Let's dive in! ๐ช
๐ The Problem: Trimming Whitespace
The question at hand is how to remove all whitespace from the start and end of a string in JavaScript. This is a common issue that arises when dealing with user inputs or reading data from external sources. Luckily, JavaScript provides a straightforward solution. ๐
โจ The Solution: Using trim()
One handy method in JavaScript that comes to the rescue is the trim()
method. This magical method eliminates whitespace from both ends of a string, leaving you with a clean and trimmed one. Let's see it in action! ๐
const str = " Hello, World! ";
const trimmedStr = str.trim();
console.log(trimmedStr);
Output:
Hello, World!
As you can see, the trim()
method removes all leading and trailing whitespace, leaving us with the desired result: "Hello, World!". ๐
๐ค What About Inner Whitespace?
If you're dealing with inner whitespace and want to remove that too, JavaScript has got your back! To remove all whitespace within the string, you can use a combination of methods such as replace()
and regular expressions. Let's take a look: ๐ง
const str = "JavaScript is amazing!";
const trimmedStr = str.replace(/\s+/g, '');
console.log(trimmedStr);
Output:
JavaScriptisamazing!
By using the replace()
method and the \s+
regular expression pattern, we match one or more whitespace characters and replace them with an empty string. This results in a string without any whitespace. How cool is that? ๐
๐ Spread the Word and Engage!
Now that you have all the tools to trim strings in JavaScript, it's time to spread the word! Share this blog post with fellow developers and help them simplify their coding lives. โ๏ธ
But we don't stop at sharing knowledge. We want to hear from YOU! Leave a comment below if you have any questions or if you want to share your own tips and tricks. Let's engage and learn from each other! ๐ค
Happy coding! ๐ปโจ