to call onChange event after pressing Enter key
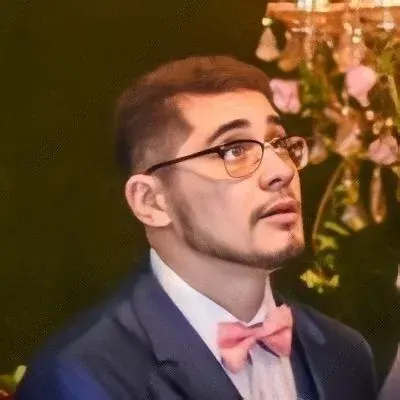
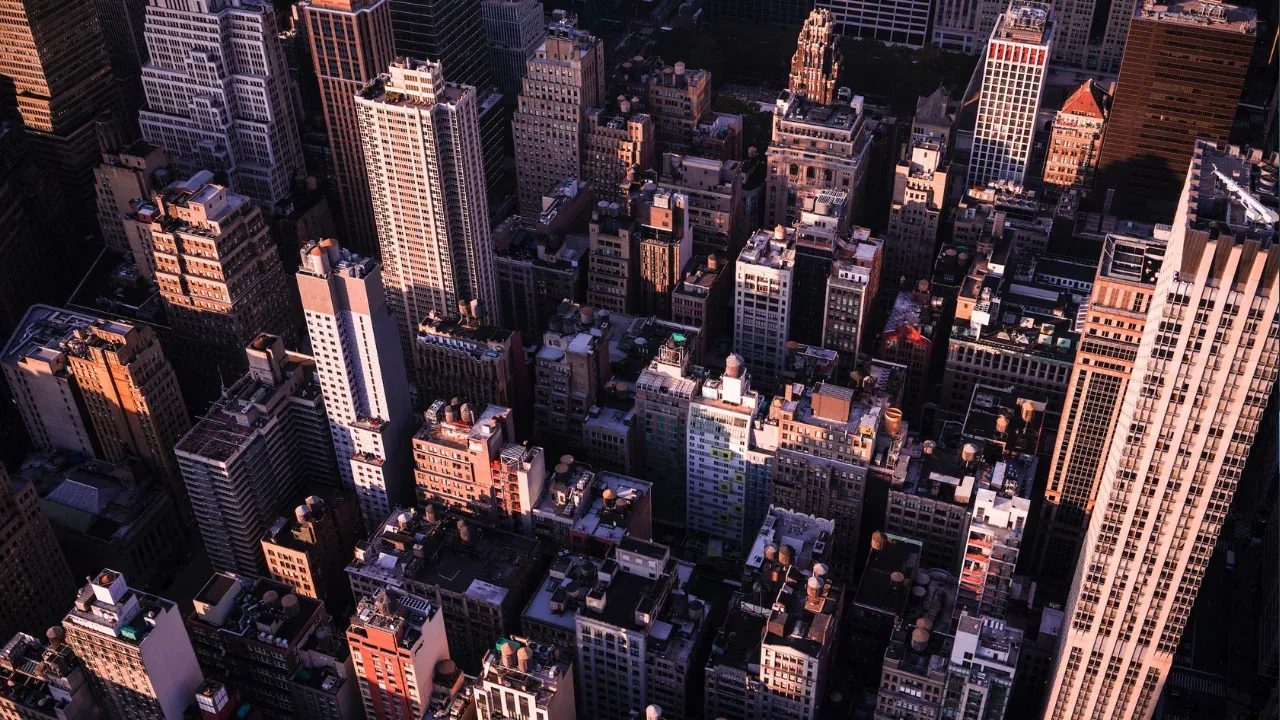
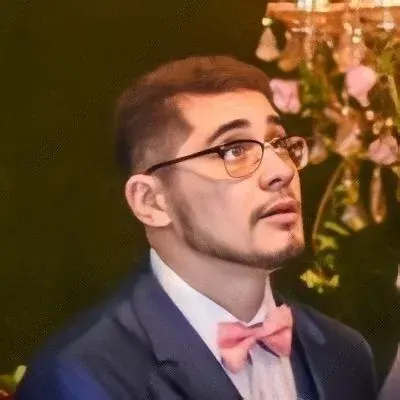
Calling the onChange Event after pressing Enter Key in React
So, you're new to Bootstrap and React and you're facing an issue where the onChange event is triggered as soon as you enter just one digit in the input field. This post will guide you through the process of calling the onChange event after pressing the Enter key or when the whole number has been entered, in a simple and easy way.
The Problem
The code snippet provided indicates that you are using React and Bootstrap to create an input field. However, the generated code does not show any specific handling of the Enter key or validation functionality.
The issue you're facing is that the onChange event is triggered immediately upon entering each digit in the input field. This behavior may not be desired, especially if you want to trigger an action only after the complete number has been entered or when the Enter key is pressed.
The Solution
To tackle this problem, we can use the onKeyDown event instead of the onChange event. This event allows us to listen for specific key presses, such as the Enter key, and perform an action accordingly.
Here's an updated snippet that demonstrates how you can modify your code to achieve the desired functionality:
var handleEnterKeyPress = (event) => {
if (event.key === 'Enter') {
// Perform your action here
console.log(event.target.value);
}
};
var inputProcent = React.CreateElement(bootstrap.Input, {
type: "text",
placeholder: this.initialFactor,
className: "input-block-level",
onKeyDown: handleEnterKeyPress,
block: true,
addonBefore: '%',
ref:'input',
hasFeedback: true
});
In this example, we've created a new function called handleEnterKeyPress
that checks if the pressed key is 'Enter'. If it is, it performs the desired action. Here, we've simply logged the entered value to the console for demonstration purposes, but you can replace it with your specific functionality.
By using the onKeyDown event, you have complete control over when the action should be triggered, in this case, after pressing the Enter key. Feel free to customize the handleEnterKeyPress
function to suit your needs.
Summary
To recap, to call the onChange event after pressing the Enter key or when the whole number has been entered in React, you can use the onKeyDown event and check for the 'Enter' key specifically. This allows you to control the timing of your desired action based on user input.
Remember to modify the code provided to include your own functionality where indicated.
Your Turn
Now that you have learned how to solve this problem, give it a try in your own code and see if it works for you. If you encounter any issues or have any questions, feel free to leave a comment below. Let's discuss and help each other out!
Happy coding! 😄👩💻👨💻