SyntaxError: Cannot use import statement outside a module
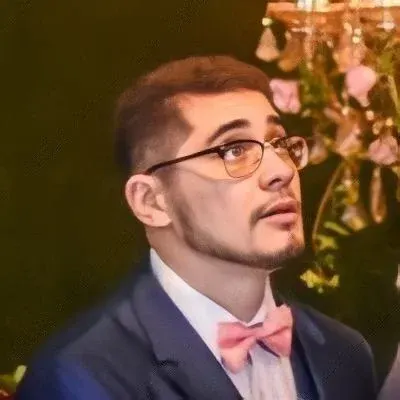
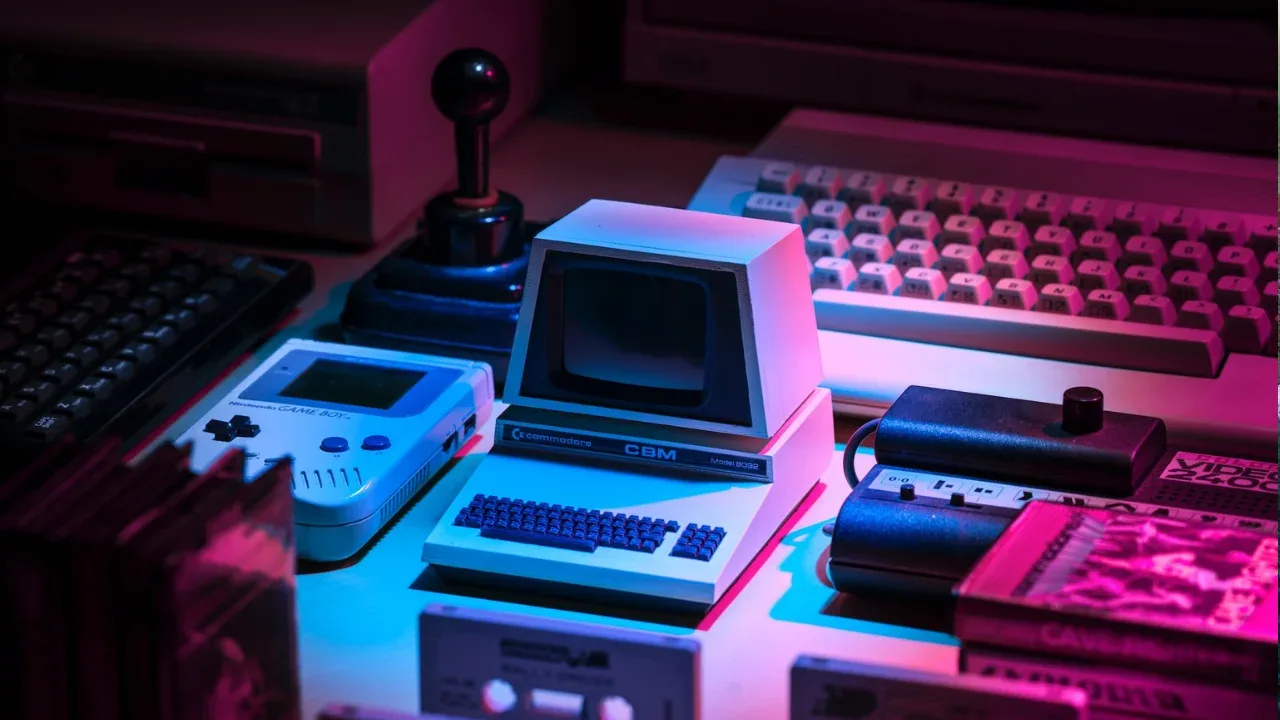
📝 Blog Post: How to Fix the SyntaxError: Cannot use import statement outside a module
Are you encountering the pesky SyntaxError: Cannot use import statement outside a module
error in your Node.js project? 😫 Don't worry, you're not alone! This error often occurs when you try to use the import
statement in a file that is not recognized as a module.
You might have encountered this issue when updating your project and using the latest version of Babel. Let's dive into the problem and explore some simple solutions that will get your project up and running smoothly again! 💪
Understanding the Issue
The error message indicates that the import
statement is not allowed outside of a module. In your case, the file where the error occurs is index.js
. The code snippet causing the issue is as follows:
require('dotenv').config()
import { startServer } from './server'
startServer()
By default, Node.js treats JavaScript files as CommonJS modules, not ECMAScript modules (ESM). However, you are using the import
syntax, which is part of the ESM syntax. Hence, the error is triggered.
Easy Solutions
Solution 1: Change import
to require
As a quick fix, you can replace the import
statement with the require
statement. Here's the updated code:
require('dotenv').config()
const { startServer } = require('./server')
startServer()
Using require
instead of import
is a valid approach for CommonJS modules, which Node.js supports by default. However, keep in mind that this solution is not ideal if your project heavily relies on ECMAScript modules.
Solution 2: Enable ECMAScript Modules (ESM) in Node.js
If you prefer using ECMAScript modules throughout your project, you can enable them in Node.js by adding the --experimental-modules
flag when running your JavaScript file.
Update your start
script in your package.json
file to include the --experimental-modules
flag, like this:
"scripts": {
"start": "node --experimental-modules index.js"
}
Then, you can revert your code back to using the import
statement like before:
require('dotenv').config()
import { startServer } from './server'
startServer()
Now, when you run your project using npm start
, Node.js will recognize the file as an ECMAScript module and handle the import
statement correctly.
Going Beyond the Basics
You might be wondering if the problem is caused by Babel, especially if you recently updated from Babel 6 to Babel 7. While Babel can be a factor, it's not the root cause of the SyntaxError
in this case.
Some solutions found online might suggest adding the type=module
attribute to your HTML file. However, this approach is not applicable to Node.js projects that run on the server-side.
Another suggestion might be to use the old Babel presets (es2015
and stage-2
) in your project's babel.config.js
file. However, using these old presets will likely result in another error: Error: Plugin/Preset files are not allowed to export objects, only functions.
To avoid these complications, stick with the easy solutions provided earlier. They are simple yet effective in resolving the SyntaxError
in most cases.
Ready to Tackle the Problem!
Now that you know how to fix the SyntaxError: Cannot use import statement outside a module
issue, give the suggested solutions a try and see which one fits your project's needs best.
Remember, don't be afraid to experiment and explore different approaches to overcome technical hurdles. If you have any questions or face any difficulties, feel free to share them in the comments section below. Let's learn and grow together! 🌱💡
Keep the Conversation Going!
Have you ever encountered the SyntaxError: Cannot use import statement outside a module
error in your Node.js projects? How did you solve it? Share your experiences, tips, and tricks in the comments below! Let's help each other and make coding easier for all! 🙌✨
✉️ Subscribe to Our Newsletter
Keep up to date with the latest tech tips, tricks, and trends! Sign up for our newsletter and never miss out on actionable insights that will level up your coding skills. Subscribe here!
📣 Share this Post
Did you find this blog post helpful? Don't keep the knowledge to yourself! Share it with your fellow developers and spread the solution to the SyntaxError: Cannot use import statement outside a module
error. Together, we can simplify the coding journey for everyone! 🚀🤝
💬 Join the Discussion
What other common programming errors have you encountered lately? Let's discuss them and find solutions to make coding more enjoyable. Join the conversation on our social media channels or leave a comment below! We can't wait to hear from you! 🗣️💡
Remember, coding problems are just temporary roadblocks on our journey towards greatness. Stay curious, keep learning, and keep coding! Happy coding! 😄👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
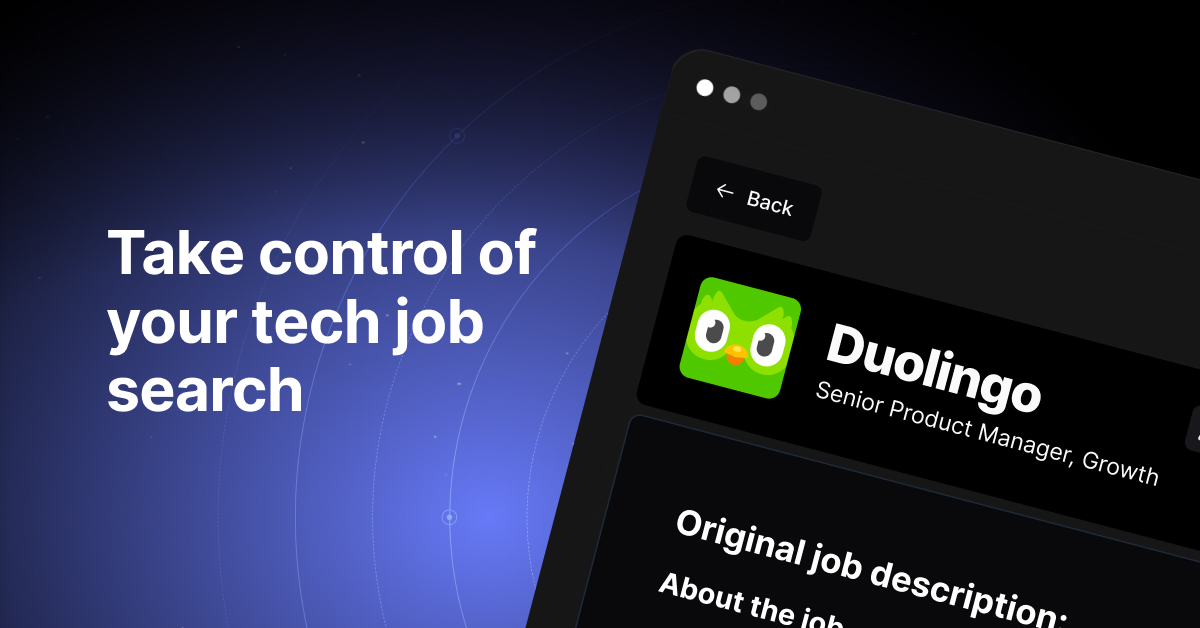