Stop setInterval call in JavaScript
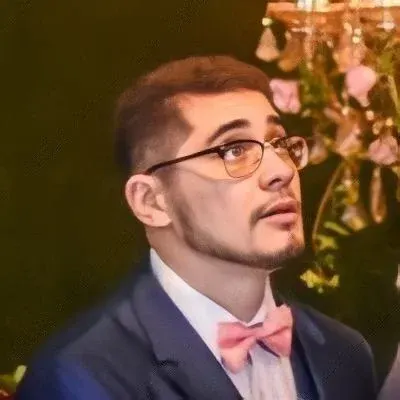
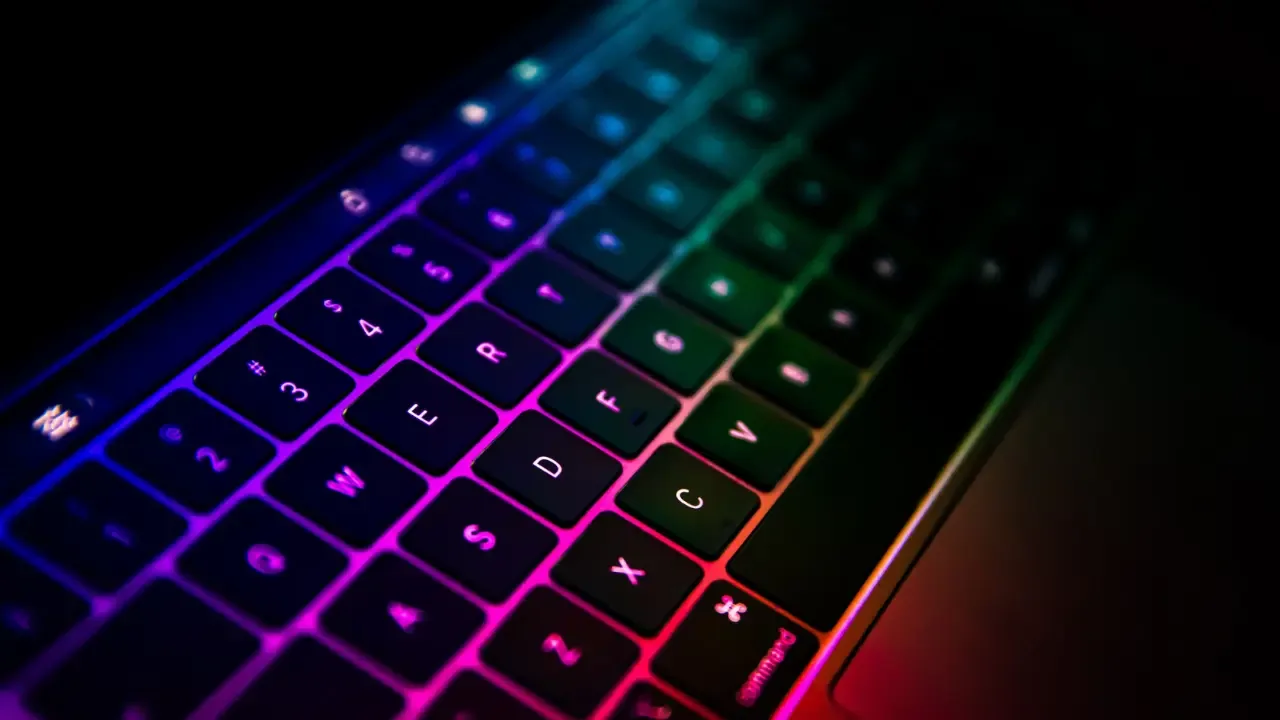
Stop setInterval call in JavaScript: How to Refresh Data on Demand 🔄⏰
Are you tired of the never-ending cycle of data refresh in your JavaScript app? 😫 Whether you're building a real-time chat application or a dynamic dashboard, stopping the setInterval
call on some event can be a lifesaver! 💪 In this blog post, we will explore common issues, provide easy solutions, and empower you to take control of your data refresh.
The Problem: Continuous Data Refresh 😩
Let's say you want to display live data updates in your app, with a function called refreshData
, every 10 seconds ⏰. You might start with this line of code:
setInterval(refreshData, 10000);
But what if the user wants to put a pause on the auto-refresh? 🤔
The Solution: clearInterval to the Rescue 🚫⏰
Thankfully, JavaScript provides a simple solution to stop the setInterval
call: the clearInterval
function! With this handy tool, you can regain control over your data refresh and let the user decide when to update. Here's how you can implement it:
// Store the interval ID in a variable
const intervalId = setInterval(refreshData, 10000);
// Stop the interval on some event
function stopRefresh() {
clearInterval(intervalId);
}
In the code snippet above, we assign the setInterval
call to a variable called intervalId
. This ID allows us to later reference and stop the interval using clearInterval(intervalId)
. The stopRefresh
function showcases an example of stopping the refresh on a specific event.
Now, let's add a button in our HTML to allow users to easily stop the refresh:
<button onclick="stopRefresh()">Stop Refresh</button>
Whenever the user clicks the "Stop Refresh" button, the stopRefresh
function will be triggered, preventing further data refreshes. 🛑🔄
Take It Further: Enhancements and Improvements 💡
While the basic solution outlined above is effective, you can take it a step further to enhance usability and add a touch of interactivity. Here are a couple of ideas to get you started:
1. Toggle On/Off Button 🔄✅🚫
Instead of just stopping the refresh altogether, you can toggle the auto-refresh feature on and off. Modify the stopRefresh
function to toggle the refresh based on the current state:
let isRefreshOn = true;
const intervalId = setInterval(refreshData, 10000);
function toggleRefresh() {
if (isRefreshOn) {
clearInterval(intervalId);
} else {
intervalId = setInterval(refreshData, 10000);
}
isRefreshOn = !isRefreshOn;
}
2. User-Defined Refresh Interval ⏲️🔄
Allowing users to define their own refresh interval adds a personal touch to your app. You can achieve this by introducing an input field on your page and using the value to update the interval duration:
<input type="number" id="refreshInterval" value="10" min="1">
<button onclick="updateRefreshInterval()">Update Interval</button>
let refreshInterval = 10000; // default value of 10 seconds
let intervalId = setInterval(refreshData, refreshInterval);
function updateRefreshInterval() {
const userInput = parseInt(document.getElementById('refreshInterval').value);
if (!isNaN(userInput)) {
refreshInterval = userInput * 1000; // convert seconds to milliseconds
clearInterval(intervalId);
intervalId = setInterval(refreshData, refreshInterval);
}
}
With this functionality, users can customize the refresh interval according to their needs. ⚙️⏰
Conclusion: Empower Users with Control 🚀💪
Don't let your JavaScript app be a victim of perpetual data refresh! ⏰ Give your users the power to decide when to update with the clearInterval
function. We've explored the easy solutions to stopping the setInterval
call, and you've seen how to enhance the feature further. Now it's time to take action! Implement this solution in your app and witness the boosted user experience. 🚀✨
Got any questions or thoughts? Share them in the comments below and let's level up our JavaScript game together! 💬🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
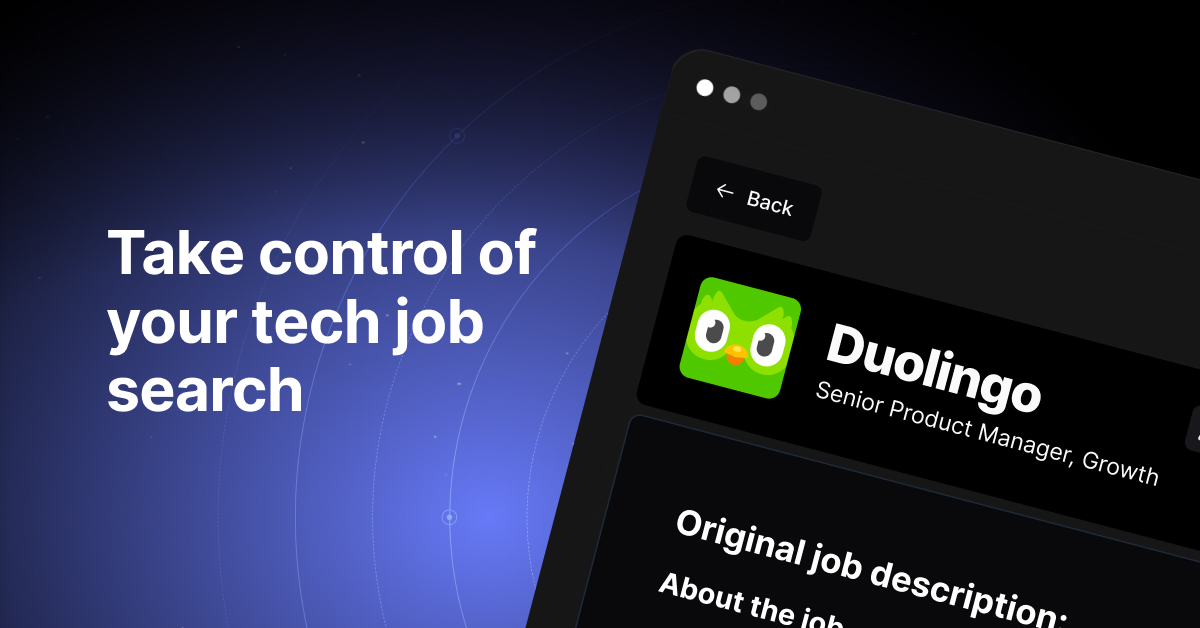