Split array into chunks
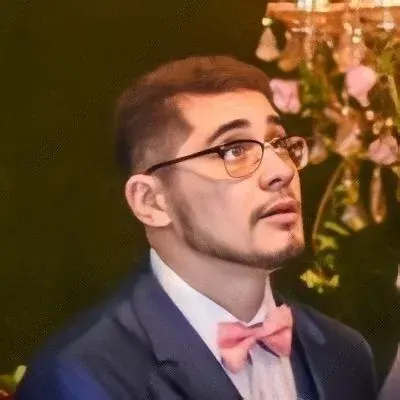
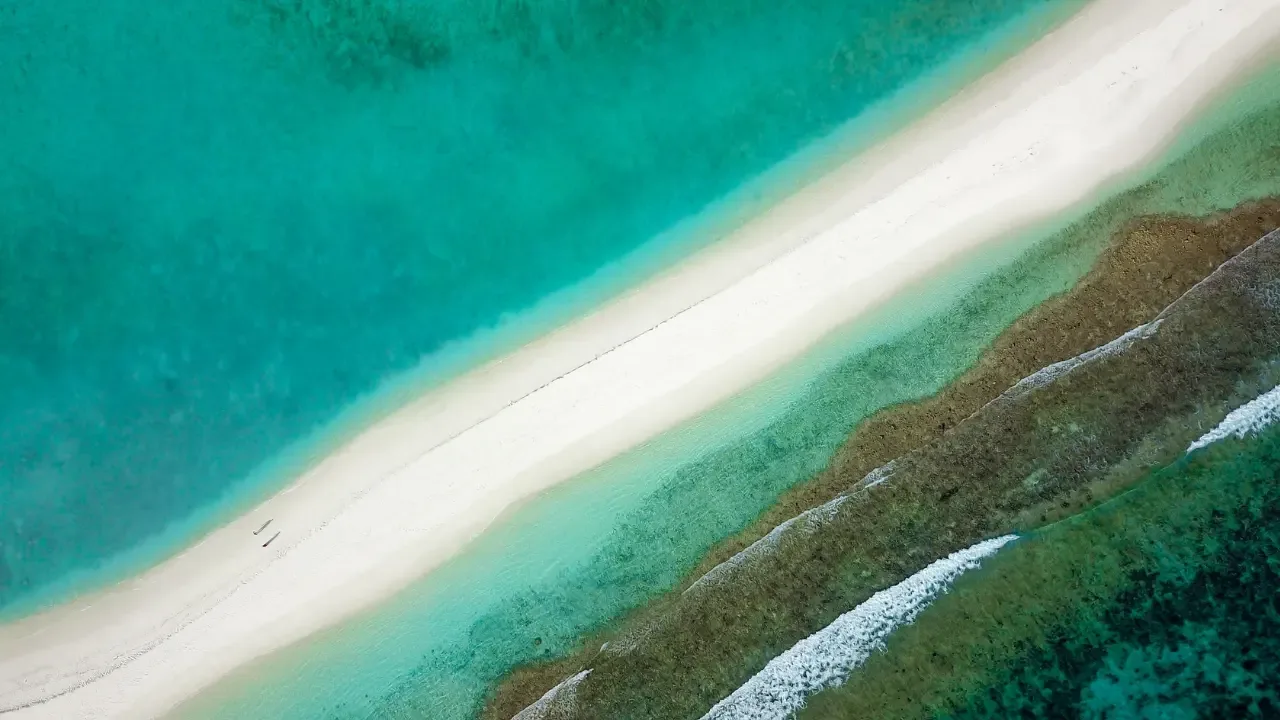
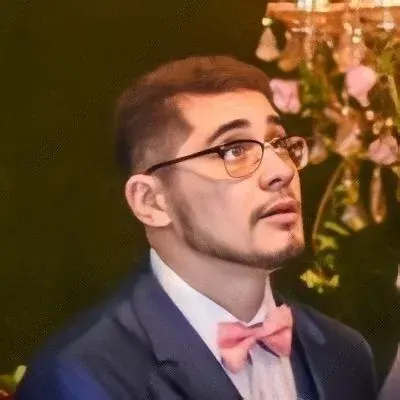
Split Array into Chunks: A Handy Guide with Easy Solutions! 💪
Do you find yourself stuck with a large JavaScript array that needs to be split into smaller, more manageable chunks? 🤔 Don't worry, we've got you covered! In this blog post, we'll explore common issues faced when splitting arrays and provide you with easy solutions. Let's dive in! 🚀
The Challenge: A Humongous Array 📚
Imagine you have a JavaScript array that looks something like this:
["Element 1","Element 2","Element 3",...]; // with close to a hundred elements.
You want to split this array into smaller arrays, each containing a maximum of 10 elements. How can you achieve this in a clean and efficient manner? Let's find out together! 😎
Easy Solution #1: The Slice Method 🍕
One approach to splitting an array into chunks is by utilizing the slice
method. This method allows you to extract a portion of an array and returns it as a new array.
const originalArray = ["Element 1","Element 2","Element 3",...];
const chunkSize = 10;
const chunks = [];
for (let i = 0; i < originalArray.length; i += chunkSize) {
const chunk = originalArray.slice(i, i + chunkSize);
chunks.push(chunk);
}
console.log(chunks);
In the code snippet above, we start by defining our original array and the desired chunk size. We create an empty chunks
array to store the smaller arrays. Then, using a for loop, we iterate over the original array, selecting chunks of size chunkSize
using the slice
method. Finally, we add each chunk to the chunks
array.
👉 Pro Tip: If the original array's length is not divisible by the chunk size, the last chunk will have fewer elements. Keep this in mind when working with arrays that are not evenly divisible.
Easy Solution #2: The Reduce Method 💡
Another elegant solution for splitting an array into chunks is by leveraging the reduce
method. This method applies a function to each element of an array, resulting in a single output value. In our case, the output value will be an array of smaller arrays.
const originalArray = ["Element 1","Element 2","Element 3",...];
const chunkSize = 10;
const chunks = originalArray.reduce((resultArray, item, index) => {
const chunkIndex = Math.floor(index / chunkSize);
if (!resultArray[chunkIndex]) {
resultArray[chunkIndex] = []; // Create a new chunk
}
resultArray[chunkIndex].push(item); // Add item to the chunk
return resultArray;
}, []);
console.log(chunks);
In this solution, we use the reduce
method on the original array and supply an empty array as the initial value. Within the reducer function, we calculate the chunk index based on the current item's index. We then create a new chunk if it doesn't exist already and add the current item to the respective chunk. Finally, we return the updated resultArray
to continue the reduction process.
Showtime! Get Chunking! 💥
Now that you have these easy solutions at your disposal, it's time to get cracking and split those arrays like a pro! Experiment with both the slice
and reduce
methods to see which one suits your needs and coding style best. Remember, it's all about finding what works best for you! 💯
We hope this blog post has been helpful in demystifying the array splitting process. If you have any questions or additional tips, please let us know in the comments section below. Let's chunk up those arrays together! ✌️
📢 Calling All Readers!
Have you ever had to deal with large arrays and splitting them into smaller chunks? How did you approach the problem? We would love to hear your experiences and insights! Share your thoughts in the comments and let's spark a discussion. Don't forget to share this post if you found it valuable! 🔥
Happy coding! 💻✨