Solve Cross Origin Resource Sharing with Flask
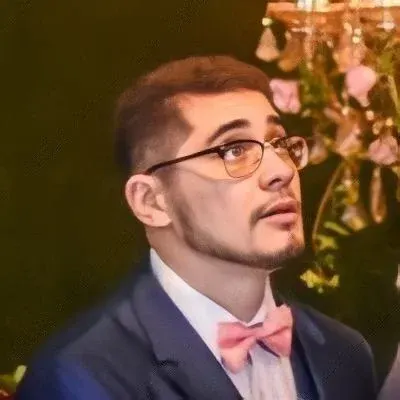
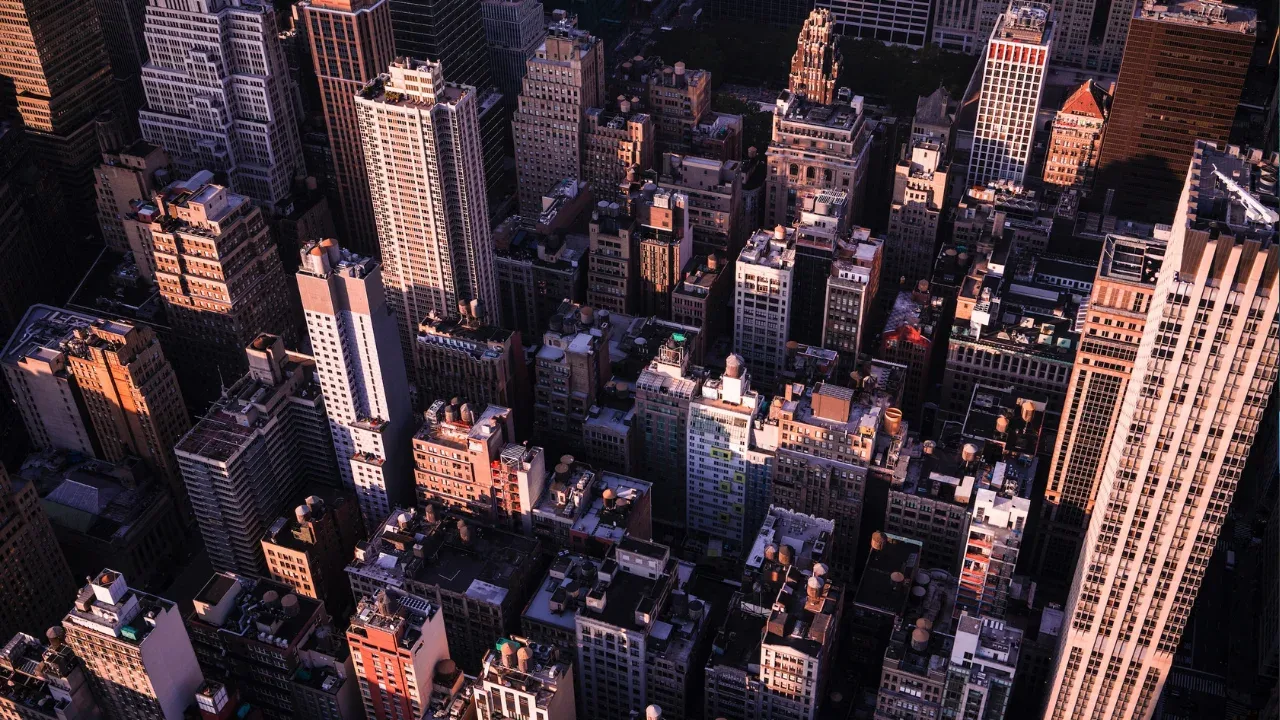
Solve Cross Origin Resource Sharing with Flask
š Cross Origin Resource Sharing (CORS) is a security mechanism implemented in browsers that restricts web pages from making requests to another domain. This is an important security measure to prevent unauthorized access to sensitive data. However, it can sometimes cause issues when making AJAX requests with Flask. If you are receiving a CORS error, such as "No 'Access-Control-Allow-Origin' header is present on the requested resource", here are a few solutions you can try.
Solution 1: Using Flask-CORS
š Flask-CORS is a Flask extension specifically designed to handle CORS and make cross-origin AJAX possible. Follow these steps to implement it:
Install Flask-CORS by running the following command in your terminal:
pip install -U flask-cors
Import the Flask module and Flask-CORS in your
pythonServer.py
file:from flask import Flask from flask_cors import CORS
Create an instance of the Flask app and enable CORS for the desired route:
app = Flask(__name__) cors = CORS(app, resources={r"/foo": {"origins": "*"}})
In this example, we are enabling CORS for the
/foo
route and allowing requests from any origin (*
). Customize this according to your needs.Add the
cross_origin
decorator to your route function:@app.route('/foo', methods=['POST', 'OPTIONS']) @cross_origin(origin='*', headers=['Content-Type', 'Authorization']) def foo(): return request.json['inputVar']
Here we are allowing
POST
andOPTIONS
requests to the/foo
route, and specifying the required headers.Run your Flask app:
python pythonServer.py
This should solve the CORS issue by correctly setting the Access-Control-Allow-Origin
header in the response.
Solution 2: Using a Specific Flask Decorator
š Another alternative is to use a specific Flask decorator that allows CORS on the functions it decorates. Here's how you can do it:
Import the necessary modules in your
pythonServer.py
file:from flask import Flask, make_response, request, current_app from datetime import timedelta from functools import update_wrapper
Define the
crossdomain
decorator function:def crossdomain(origin=None, methods=None, headers=None, max_age=21600, attach_to_all=True, automatic_options=True): # Implementation details here...
This code snippet was taken from the official Flask documentation and provides a decorator to handle CORS.
Add the
@crossdomain
decorator to your route function:@app.route('/foo', methods=['GET', 'POST', 'OPTIONS']) @crossdomain(origin="*") def foo(): return request.json['inputVar']
Here we are allowing
GET
,POST
, andOPTIONS
requests to the/foo
route, and specifying the origin as"*"
to allow requests from any domain.Run your Flask app:
python pythonServer.py
This decorator will handle the necessary headers and ensure the CORS error is resolved.
Conclusion
š” CORS issues can be tricky to solve, but with the help of Flask-CORS or a specific decorator, you can easily enable cross-origin AJAX requests in your Flask app. Give these solutions a try and see which one works for you.
š If you found this guide helpful, make sure to share it with others facing similar challenges. Let us know in the comments if you have any questions or other solutions to share. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
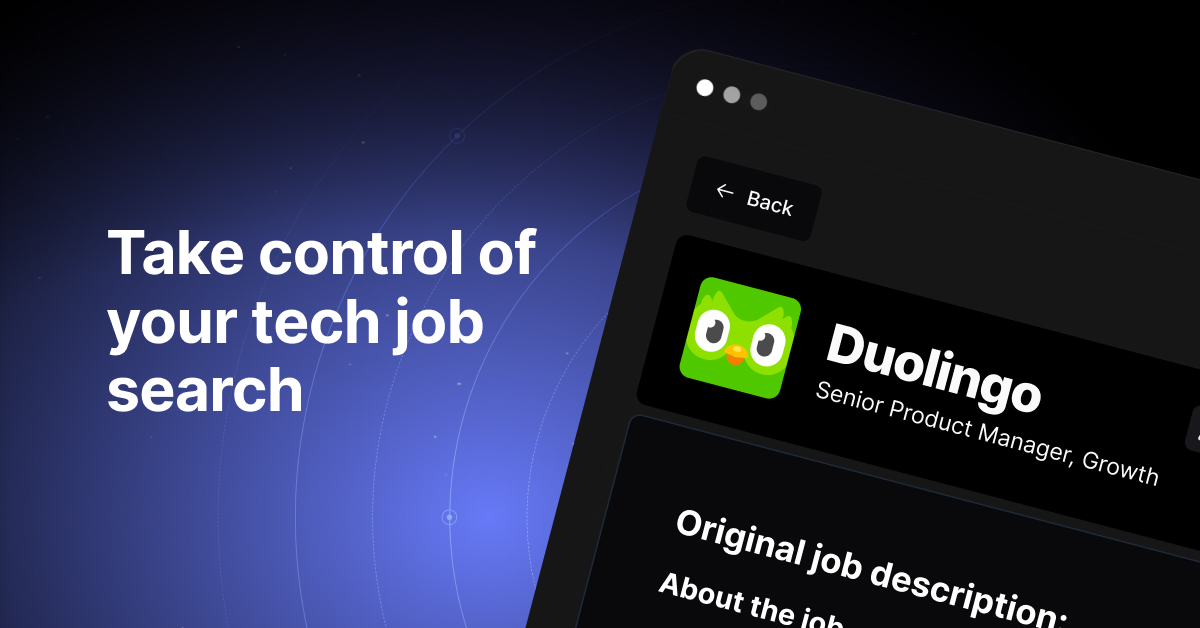