Show or hide element in React
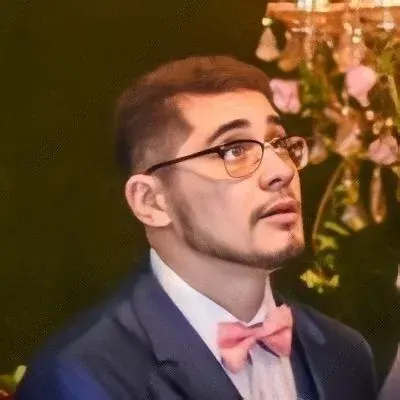
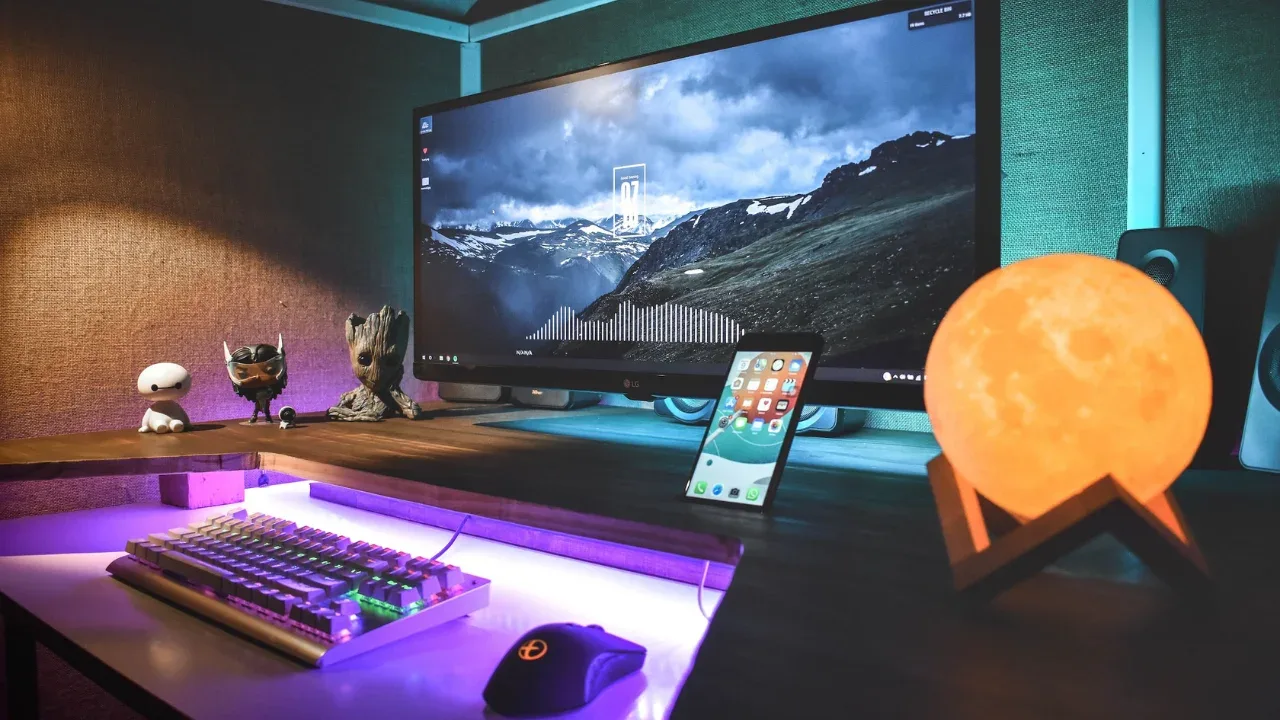
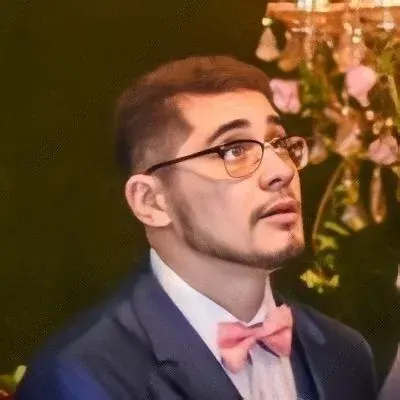
Show or Hide Element in React: A Simple Guide 👀✨
So, you're using React.js for the first time and want to show or hide an element on your page whenever a click event occurs. No worries, my friend, I've got you covered! In this guide, I'll walk you through the process of achieving this using React.
The Problem 🤔
Imagine you have a button, and whenever it's clicked, you want to show or hide a specific element on your page. In this case, you want to show the "results" div when the click event fires.
Based on the code snippet you provided, you're on the right track! We just need to make a couple of tweaks.
The Solution 💡
Here's what you can do to accomplish your goal:
First, let's define a state property within your
Search
component to keep track of whether the results should be shown or hidden. We'll call itshowResults
and initialize it asfalse
. YourSearch
component should look like this:
var Search = React.createClass({
getInitialState: function() {
return {
showResults: false,
};
},
handleClick: function() {
this.setState({ showResults: !this.state.showResults });
},
render: function() {
return (
<div className="date-range">
<input
type="submit"
value="Search"
onClick={this.handleClick}
/>
{this.state.showResults && <Results />}
</div>
);
},
});
In the above code, we've added a new condition (
{this.state.showResults && <Results />}
) within therender
method. This condition checks ifshowResults
istrue
, and if so, it renders theResults
component.Lastly, we need to update the
Results
component to reflect the changes. It should remain the same:
var Results = React.createClass({
render: function() {
return (
<div id="results" className="search-results">
Some Results
</div>
);
},
});
Conclusion 🎉
That's it! You've successfully learned how to show or hide an element in React using a click event. Pretty neat, right? Now you can apply this knowledge to create more dynamic and interactive UIs with React.
If you have any questions or run into any issues, don't hesitate to reach out. I'd be happy to help you out!
Your Turn! 🚀
Try implementing this solution in your code and see it in action. Click that button and watch the results div magically show and hide on your page. Once you've done it, share your experience in the comments section below. I'd love to hear your thoughts and insights!
Also, if you found this guide helpful, don't forget to share it with your fellow React enthusiasts. Together, we can make the React community thrive! ✨
Happy coding! 💻🔥