Short circuit Array.forEach like calling break
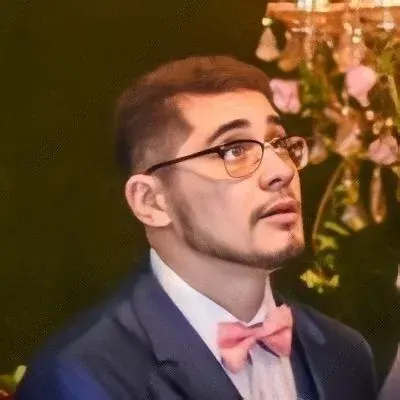
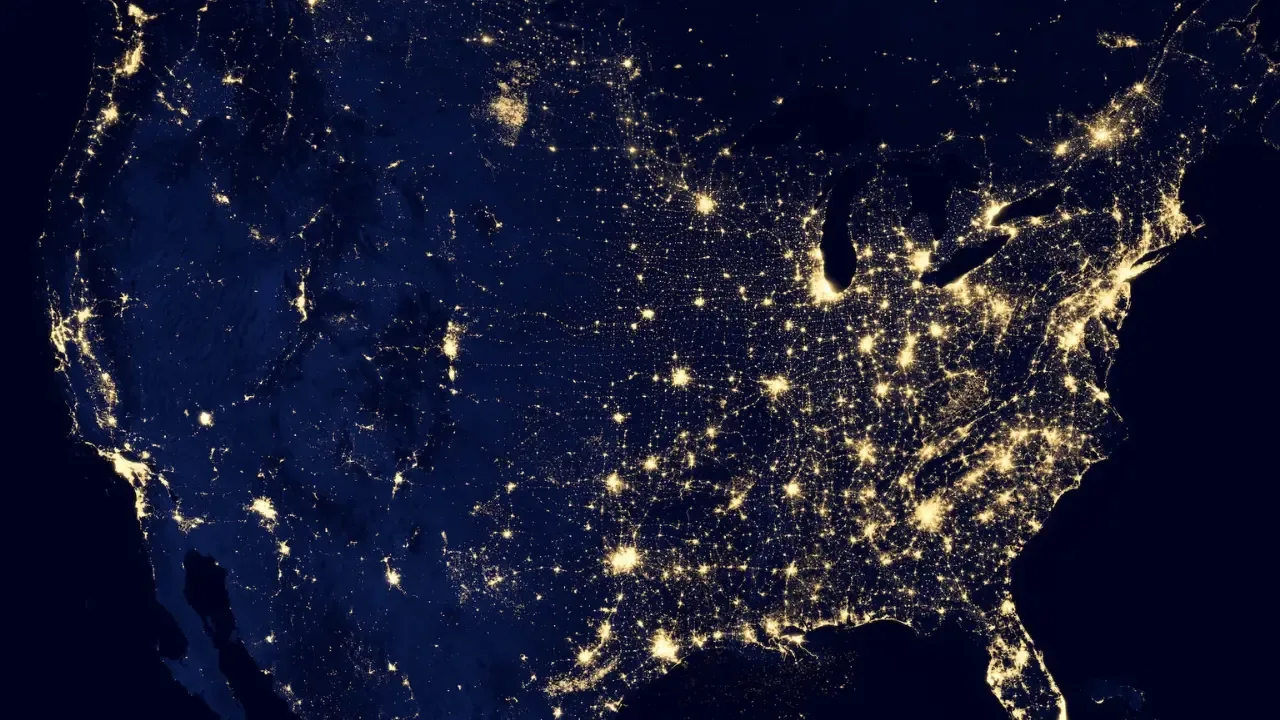
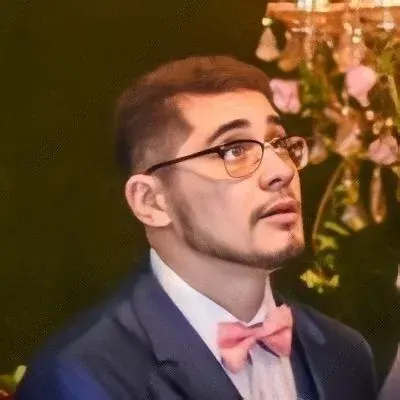
💥 Short Circuit Array.forEach
like calling break
Have you ever wanted to prematurely exit a loop in JavaScript when using the forEach
method? Maybe you've tried using return;
, return false;
, or even the infamous break
keyword, but none of them seem to work as expected. In this blog post, we'll explore the limitations of the forEach
method and provide easy solutions to mimic the behavior of a traditional break
.
The Problem
Let's take a look at an example to understand the issue:
[1, 2, 3].forEach(function(el) {
if(el === 1) break;
});
When you run this code, you'll encounter a syntax error. The break
statement is not valid inside a forEach
loop. 😱
Understanding the Limitations
The forEach
loop in JavaScript was designed to iterate over each element of an array and perform a given operation for each element. However, it does not provide a built-in mechanism to break the loop prematurely. This can be quite frustrating, especially if you come from a programming background where break
is a common feature. 😤
Easy Solutions
Using
Array.some
method:
[1, 2, 3].some(function(el) {
if(el === 1) return true; // short-circuit the loop
// perform your logic here
});
The some
method, unlike forEach
, terminates the loop early if the given callback function returns true
. This feature allows you to achieve the desired behavior similar to a break
statement.
Using a simple
for...of
loop:
for(let el of [1, 2, 3]) {
if(el === 1) break; // break the loop
// perform your logic here
}
With a traditional for...of
loop, you have full control over the loop's flow and can break out of it using the break
keyword.
Conclusion
Although the forEach
method in JavaScript lacks a built-in break statement, there are alternatives you can use to achieve similar functionality. By adopting either the Array.some
method or a simple for...of
loop, you can easily short circuit the iteration process.
Don't let the limitations of forEach
hold you back! Give these solutions a try and see how they work for you. Let us know your thoughts in the comments below. 👇
Your Turn
Have you ever run into issues with Array.forEach
and its lack of a break statement? How did you overcome this challenge? Share your experiences in the comments and let's help the community find creative ways to tackle this problem together! 😄