Share data between AngularJS controllers
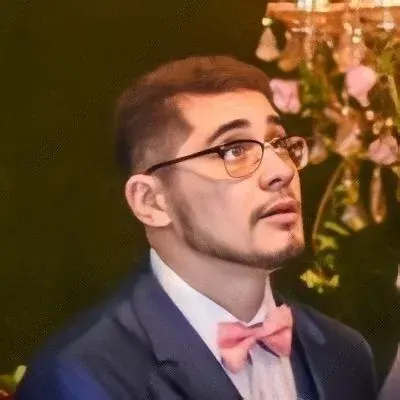
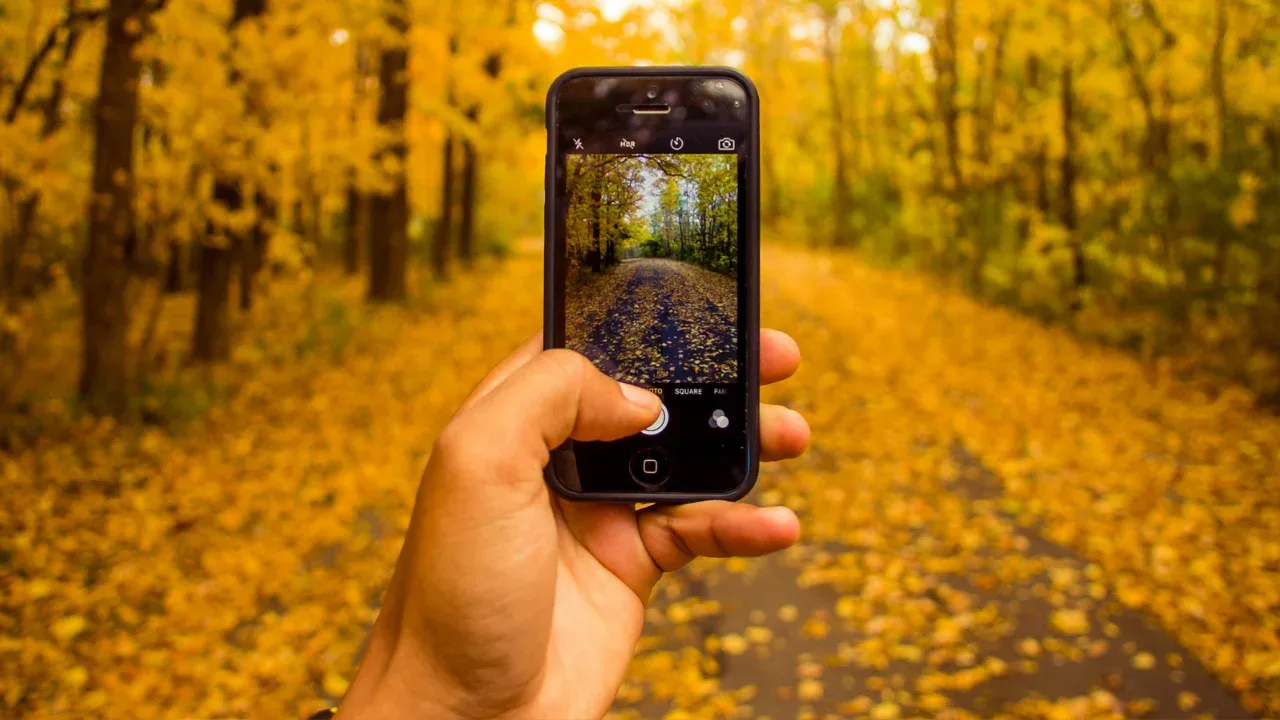
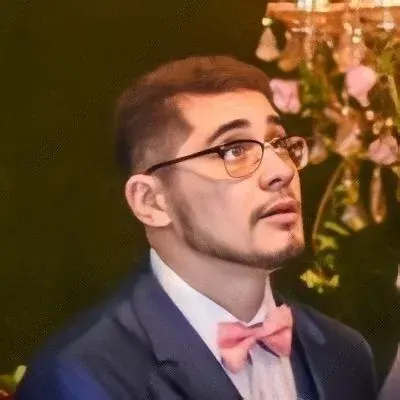
Share Data Between AngularJS Controllers - The Ultimate Guide! 📊
Are you struggling to share data between AngularJS controllers? 🤔 Don't worry, you're not alone! Many developers face this issue when working with multi-step forms or when data entered in one controller needs to be accessed in another controller. In this blog post, we'll explore common issues, provide easy solutions, and help you seamlessly share data between AngularJS controllers. Let's dive in! 💪
The Challenge 🚀
Imagine you have a multi-step form where data entered in one input needs to be displayed in multiple locations outside the original controller. In the code snippet provided, the FirstName
input value needs to be automatically updated in the second controller (SecondCtrl
). However, the current implementation is not working as expected. 😩
The Solution 🎯
To share data between controllers in AngularJS, we'll leverage the power of a factory. A factory allows us to create objects that can be shared and injected into different AngularJS components. Let's see how we can modify the code to achieve the desired result. 🛠️
Step 1: Create a Factory
// Declare the app with no dependencies
var myApp = angular.module('myApp', []);
// Create a factory to share data between controllers
myApp.factory('Data', function(){
// Initialize an object with properties to be shared
var data = {
FirstName: ''
};
return data;
});
In this step, we've created a factory called Data
using the factory
function from the AngularJS module. The Data
factory returns an object with a property called FirstName
initialized to an empty string.
Step 2: Update the Controllers
// Step 1 Controller
myApp.controller('FirstCtrl', function($scope, Data){
// Access the factory object and update the 'FirstName' property
$scope.updateFirstName = function(firstName){
Data.FirstName = firstName;
};
});
// Step 2 Controller
myApp.controller('SecondCtrl', function($scope, Data){
// Access the factory object and bind the 'FirstName' property to the scope
$scope.FirstName = Data.FirstName;
});
In the first controller (FirstCtrl
), we've added an updateFirstName
function to update the FirstName
property of the Data
factory. This function can be called from the HTML using ng-click
or any other trigger event.
In the second controller (SecondCtrl
), we're simply binding the FirstName
property of the Data
factory to the scope. Now, any changes made to FirstName
in the first controller will automatically reflect in the second controller.
Step 3: HTML Update
<!-- Step 1 Controller -->
<div ng-controller="FirstCtrl">
<input type="text" ng-model="FirstName" ng-change="updateFirstName(FirstName)">
<br>Input is: <strong>{{FirstName}}</strong><!-- Successfully updates here -->
</div>
<hr>
<!-- Step 2 Controller -->
<div ng-controller="SecondCtrl">
Input should also be here: {{FirstName}}<!-- Data is now automatically updated here! -->
</div>
Finally, we've updated the HTML code to bind the FirstName
model to the input element in the first controller. We've also added the ng-change
directive to call the updateFirstName
function whenever the input value changes.
In the second controller, we're now able to display the updated FirstName
value, which will automatically reflect any changes made in the first controller.
Time to Share and Shine! ✨
You've learned how to seamlessly share data between AngularJS controllers using a factory. No more tearing your hair out trying to access data outside of a controller! 🙌
Feel free to apply these techniques to your own projects and let us know how it goes. Sharing is caring, after all! 😊
If you found this guide helpful, why not share it with your developer friends? Let's spread the knowledge and make AngularJS development a breeze for everyone! 💪
Got any more AngularJS challenges or questions? Drop them in the comments below, and let's collaborate to find the perfect solutions! 👇-