Setting "checked" for a checkbox with jQuery
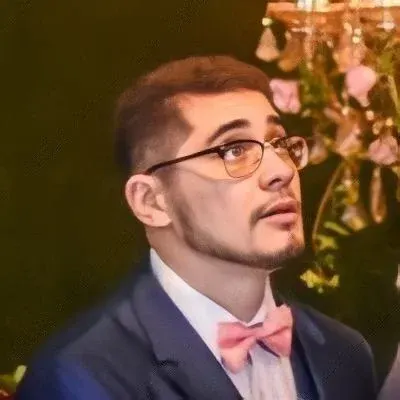
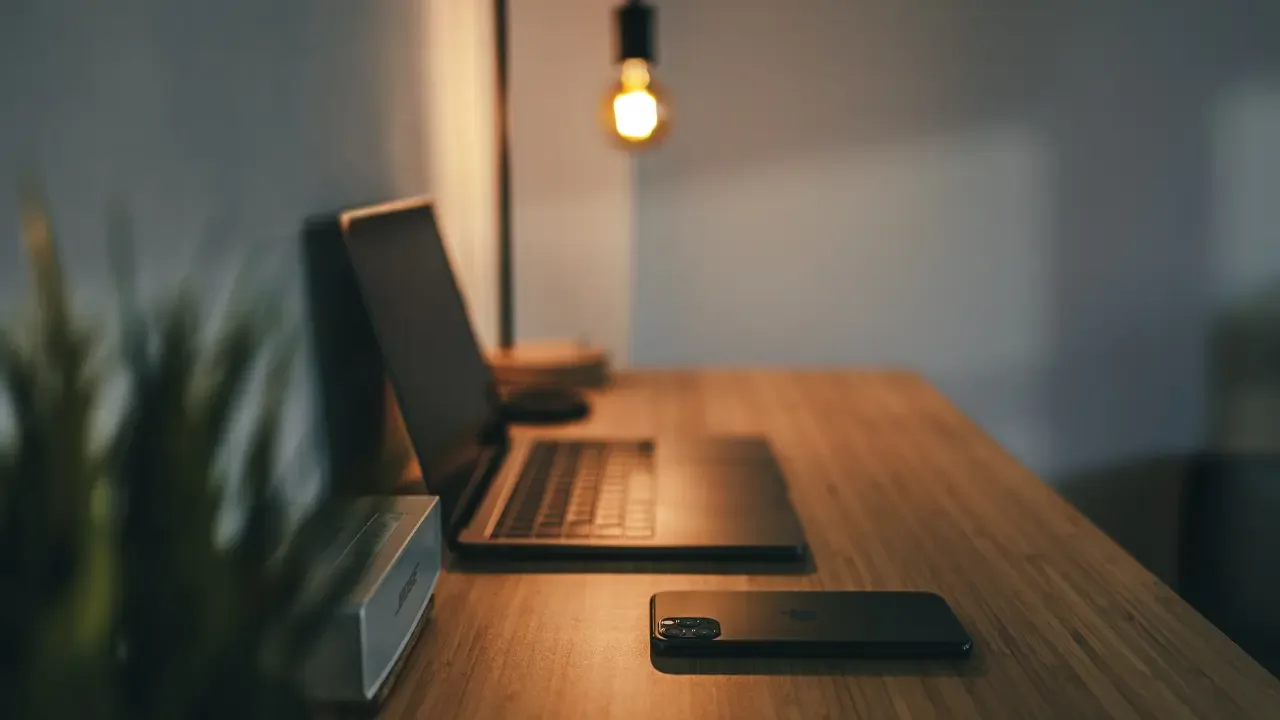
Setting "checked" for a checkbox with jQuery 📝
Have you ever come across the need to programmatically check a checkbox using jQuery? Maybe you have dynamically generated checkboxes or you simply want to manipulate the checkbox state through jQuery. Either way, you'll be glad to know that a solution exists! 😄
The Challenge
In the example you provided, the goal is to check a checkbox using jQuery. Let's take a closer look at the code snippet:
$(".myCheckBox").checked(true);
or
$(".myCheckBox").selected(true);
At first glance, you may think this code will work. But jQuery does not provide a .checked()
or .selected()
method to set the "checked" attribute of checkboxes. So, we need to find an alternative solution.
The Solution
To set the "checked" state of a checkbox using jQuery, we can use the .prop()
method instead. Here's how we can modify the code snippet:
$(".myCheckBox").prop("checked", true);
And there you go! This simple tweak will check the checkbox with the class "myCheckBox" by updating the "checked" property to true
.
But what if you want to uncheck the checkbox? Just change the second argument of .prop()
to false
:
$(".myCheckBox").prop("checked", false);
Now you have the power to check or uncheck checkboxes dynamically using jQuery. 🎉
Take It Further
Now that you know how to set the "checked" state of a checkbox with jQuery, why stop there? You can explore other jQuery methods and events to enhance your checkbox manipulation experience. Here are a few ideas to get you started:
Triggering events – Use the
.trigger()
method to programmatically trigger click events on checkboxes. This can be useful for simulating user interactions.Iterating over multiple checkboxes – If you have a group of checkboxes and want to manipulate them collectively, use the
.each()
method to iterate over each checkbox and apply changes accordingly.Listening for checkbox changes – Use the
.change()
event to listen for changes in checkbox states. You can then perform specific actions based on the current state of the checkboxes.
Remember, always refer to the official jQuery documentation for detailed information about these methods and events. 📚
Conclusion
Setting the "checked" state for a checkbox using jQuery is a common task, but it can sometimes lead to confusion. By using the .prop()
method, you can easily manipulate the checkboxes to match your requirements with just a few lines of code.
Now that you have the knowledge, go ahead and start experimenting with jQuery to level up your checkbox game! 💪 And don't forget to share your experiences and creative solutions in the comments below.
Happy coding! 😊🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
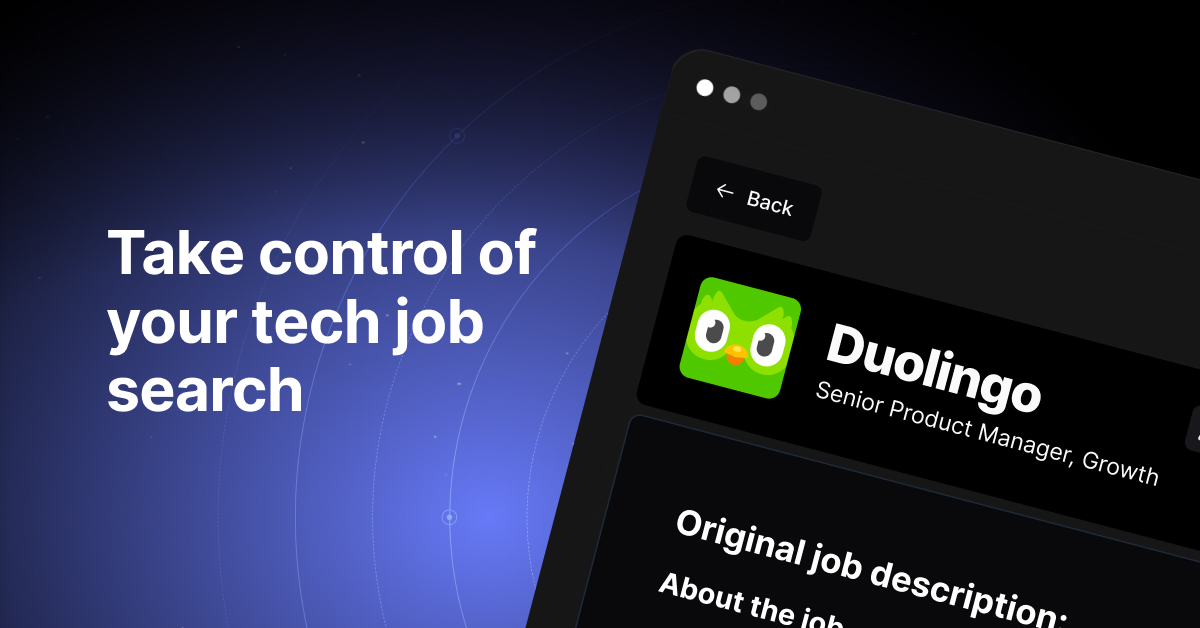