Setting a backgroundImage With React Inline Styles
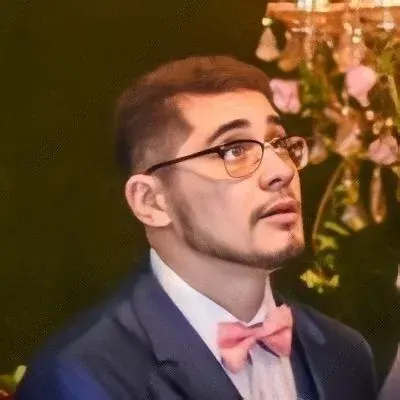
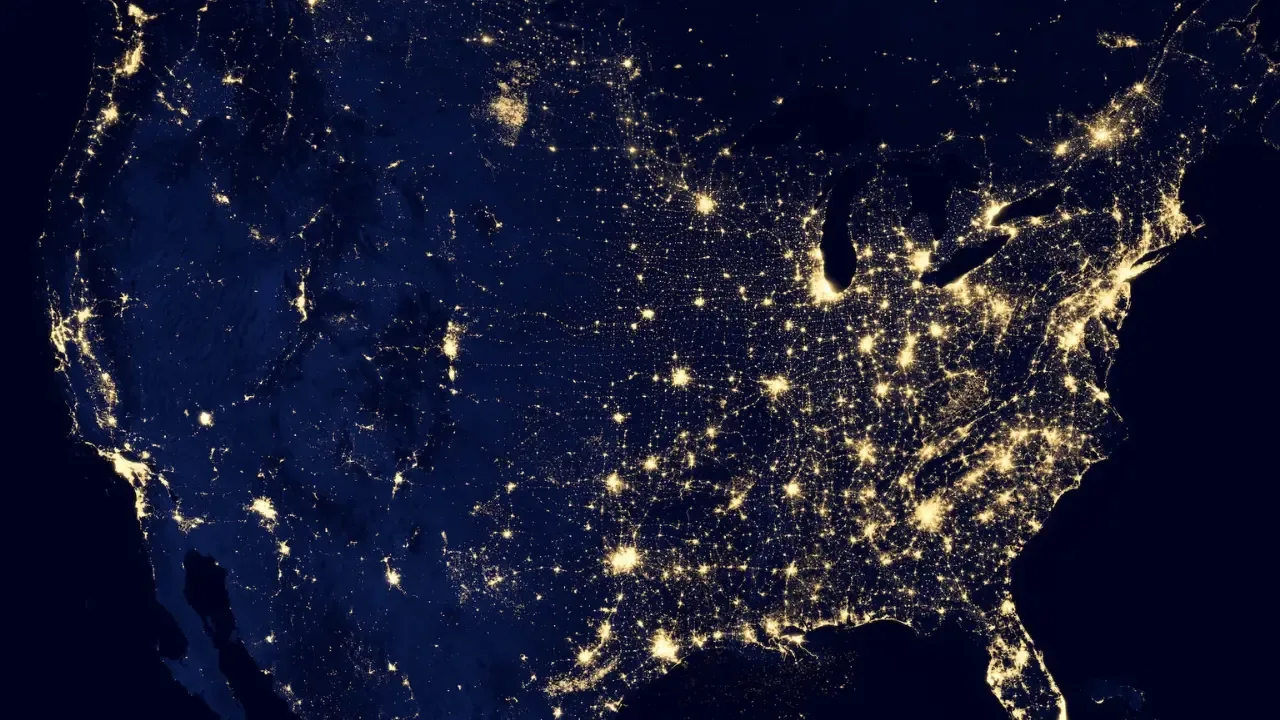
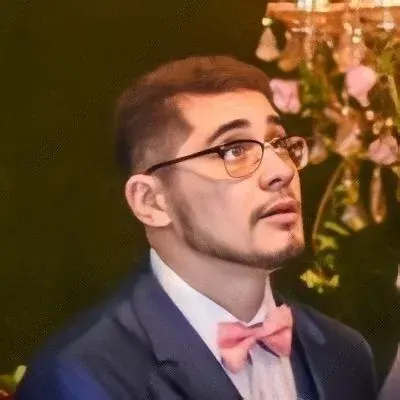
Setting a backgroundImage With React Inline Styles 💥🌈
So, you're trying to set a backgroundImage
using inline styles in React, huh? 🖼️🚀 Don't worry, you're not alone! Many React developers have faced this hurdle. But fear not, my friend! I'm here to guide you through this challenge and help you achieve those beautiful background images effortlessly. Let's dive in! 💪
Understanding the Problem 💡
The first step is understanding the difference between setting an src
attribute for an <img>
tag and setting a backgroundImage
property for an element using inline styles in React. 🤔
When you use the src
attribute for an <img>
tag, React knows how to handle it and includes the image in the final compiled output. Easy peasy! 👍
However, when it comes to setting a backgroundImage
using inline styles, things get a bit trickier. React processes the styles you pass as an object and converts them to valid CSS. But when you try to pass an imported image directly, it won't work as expected. 😫
The Solution 🚀
But worry not, my fellow React enthusiast! There's a simple workaround to make it work. 🎉
Instead of directly passing the imported image as a value for backgroundImage
, you need to convert it to a URL format. And here's how you can do it:
import Background from '../images/background_image.png';
var sectionStyle = {
width: "100%",
height: "400px",
backgroundImage: `url(${Background})`
};
class Section extends Component {
render() {
return (
<section style={sectionStyle}>
</section>
);
}
}
Voilà! 🎩✨ By wrapping the Background
variable with template literals using ${}
within the url()
function, you're now providing a valid URL for the image. React will happily convert it into a valid CSS background image for you. 🙌💅
The Power of Examples 💪🌟
Still not convinced? Let me provide a crystal-clear example for you. 🌠
Imagine you have an image called background_image.png
located in the ../images
directory. In your <Section>
component, you want to set it as the background image using inline styles.
The incorrect way would be to do this:
backgroundImage: "url(" + Background + ")"
However, this won't work as expected. 😕
Now, applying our solution, you'll do this instead:
backgroundImage: `url(${Background})`
And ta-da! 🎉🌈 React will handle it like a charm, and your background image will shine bright and beautiful! ✨🌟
Your Turn to Shine! 🌞
Now that you know how to set a backgroundImage
with React inline styles, it's time for you to unleash your creative genius! 🎨✨ Add stunning backgrounds to your React components and make them shine like never before. 🌈
But don't stop here! Share your experiences, thoughts, and any other cool tips or tricks you've discovered along the way in the comments below. Let's learn and grow together! 🌱🤝
Happy coding! 💻😄