Set a default parameter value for a JavaScript function
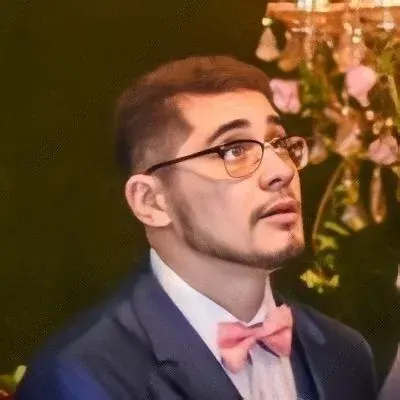
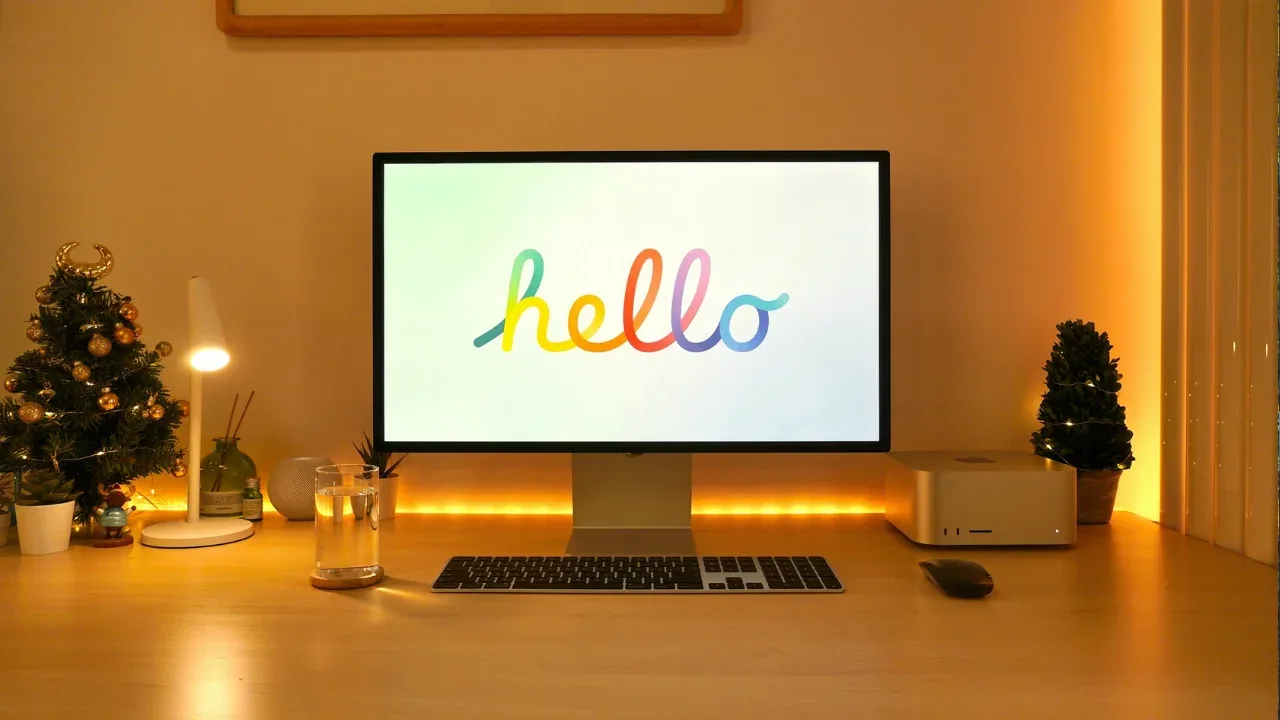
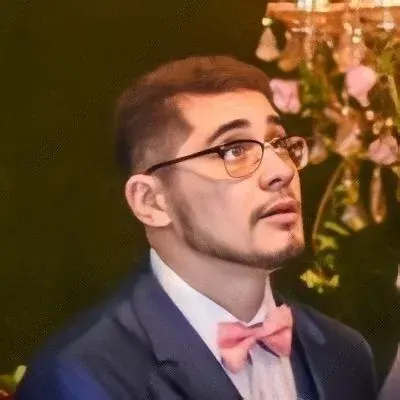
📝 Blog Post: How to Set a Default Parameter Value for a JavaScript Function
Are you tired of dealing with undefined or missing arguments in your JavaScript functions? 🤔 Look no further! In this blog post, we'll explore how to set default parameter values for your JavaScript functions, just like in Ruby! 💎
The Common Problem 😕
Imagine this scenario: you have a JavaScript function, and you want to make some of its arguments optional. In addition, you want to set a default value for these optional arguments, so they have a fallback value if not explicitly provided. But wait, is this even possible in JavaScript? 🤔
The Ruby Inspiration 💡
In Ruby, you can easily define default parameter values for your functions. For example, take a look at this Ruby function:
def read_file(file, delete_after = false)
# code
end
In this example, if the delete_after
argument is not provided when calling the function, it will default to false
. This feature can save you from writing repetitive code. 😌
JavaScript to the Rescue 🚀
Good news! JavaScript has caught up with Ruby and now provides a similar way to set default parameter values. Here's the JavaScript equivalent function:
function read_file(file, delete_after = false) {
// Code
}
Yes, you read it correctly! JavaScript now supports setting default parameter values directly in the function signature. 🎉
In the above example, if the delete_after
argument is not provided, it will default to false
just like in Ruby. So, you can take advantage of this feature to make your code cleaner and more concise.
Compatibility Considerations 🔄
It's important to note that setting default parameter values in JavaScript is a modern JavaScript feature introduced in ECMAScript 2015 (ES6). As a result, it might not work in older browsers or JavaScript environments that don't support ES6.
To ensure compatibility, you can use other techniques like the logical OR (||
) operator or the typeof
operator to achieve similar functionality in older JavaScript versions. However, these techniques can be verbose and less intuitive. Therefore, it's recommended to use default parameters if you can rely on modern JavaScript support. 😉
Conclusion 🎯
Setting default parameter values for JavaScript functions has never been easier! Thanks to modern JavaScript, you can now enjoy the same convenience and clarity found in Ruby when it comes to optional arguments.
So, go ahead and start implementing default parameter values in your functions, and watch your code become more readable and maintainable. 💪
If you found this blog post helpful, don't forget to share it with your fellow JavaScript developers! And if you have any other JavaScript-related questions or suggestions, feel free to leave a comment below. Let's keep the conversation going! 🤗