Sending command line arguments to npm script
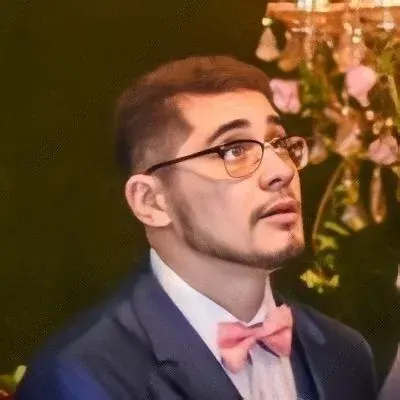
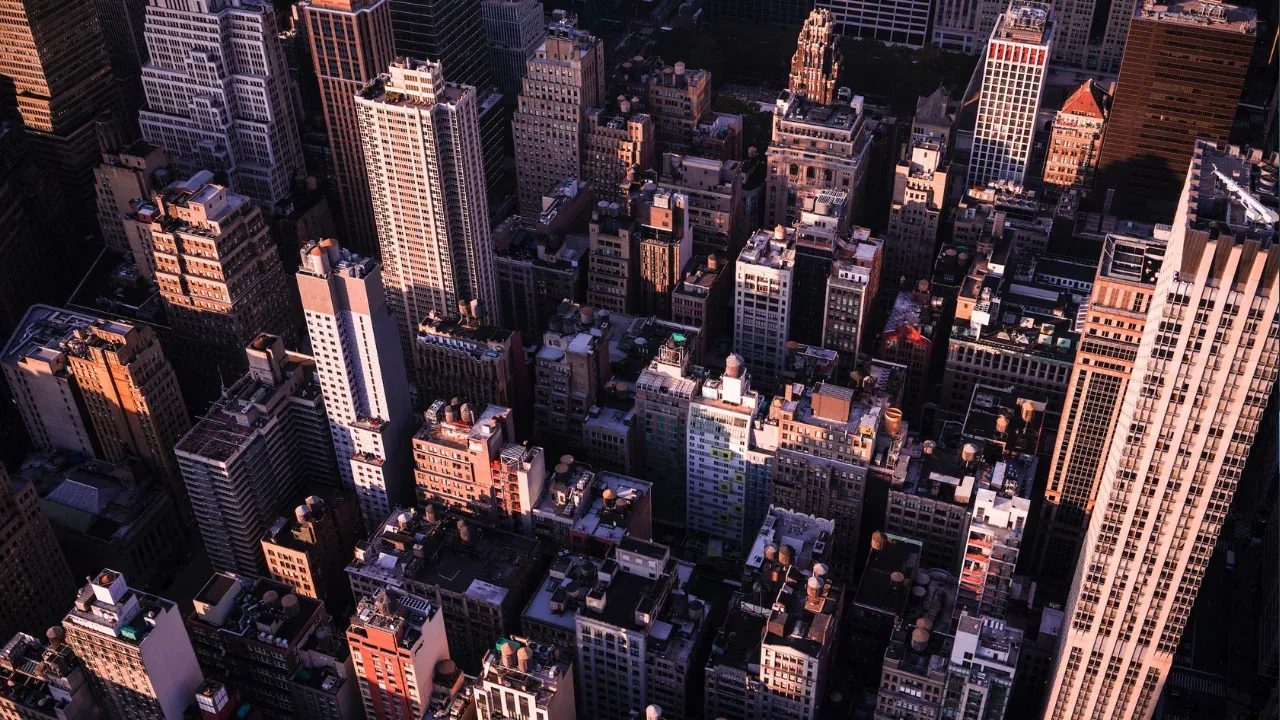
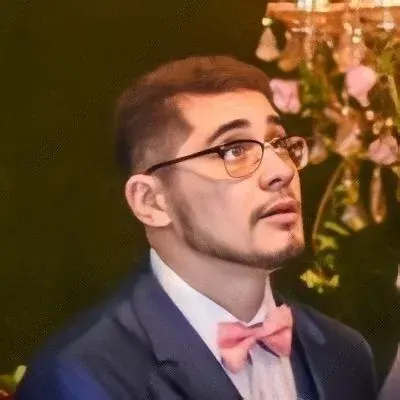
Sending Command Line Arguments to npm Script
So, you want to send command line arguments to your npm script, huh? You're in the right place! 😎
The Problem
As a developer, you may have encountered a situation where you want to pass command line arguments to your Node.js script when running it with npm. By default, npm does not provide a straightforward way to achieve this. 😕
The Solution
Fortunately, there are a couple of easy solutions to address this problem. Let's dive right in! 💪
Option 1: Use the --
Delimiter
One way to pass command line arguments to an npm script is by using the --
delimiter. This delimiter separates the npm command from the actual arguments.
Let's update the scripts
section in your package.json
file to include the --
delimiter:
"scripts": {
"start": "node ./script.js server --"
}
Now, you can run your script with additional arguments by appending them after the --
delimiter:
npm start -- 8080
This command will translate to node ./script.js server -- 8080
, allowing you to access the 8080
argument in your script.
Option 2: Use the env
Property
Another way to pass command line arguments to an npm script is by using the env
property. This property allows you to define environment variables, including the command line arguments.
Update the scripts
section in your package.json
file as follows:
"scripts": {
"start": "node ./script.js server"
},
"start-args": "--"
To run your script with additional arguments, use the npm run-script
command with the --
delimiter followed by your desired arguments:
npm run-script start -- 8080
This command will execute node ./script.js server
and pass 8080
as an argument.
Option 3: Use a Third-Party Solution
If you find the above options cumbersome or they don't meet your requirements, you can opt for a third-party package like npm-run-all
or cross-env
.
These packages provide more advanced options for running scripts with command line arguments and offer additional features that may be useful in complex setups.
Conclusion
Sending command line arguments to npm scripts is now within your grasp! 🚀 You can choose between using the --
delimiter or the env
property in your package.json
file. If needed, you can also explore third-party solutions like npm-run-all
or cross-env
.
So, what are you waiting for? Go ahead and give it a try! Let us know in the comments below if you found this guide helpful or if you have any other questions. Happy coding! 💻❤️