Send POST data using XMLHttpRequest
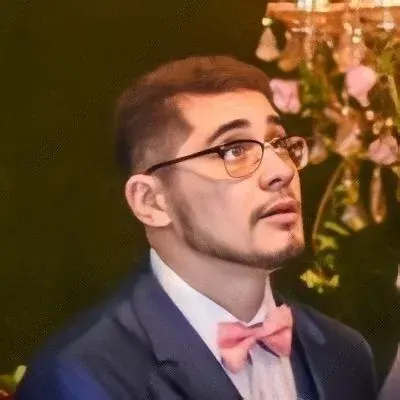
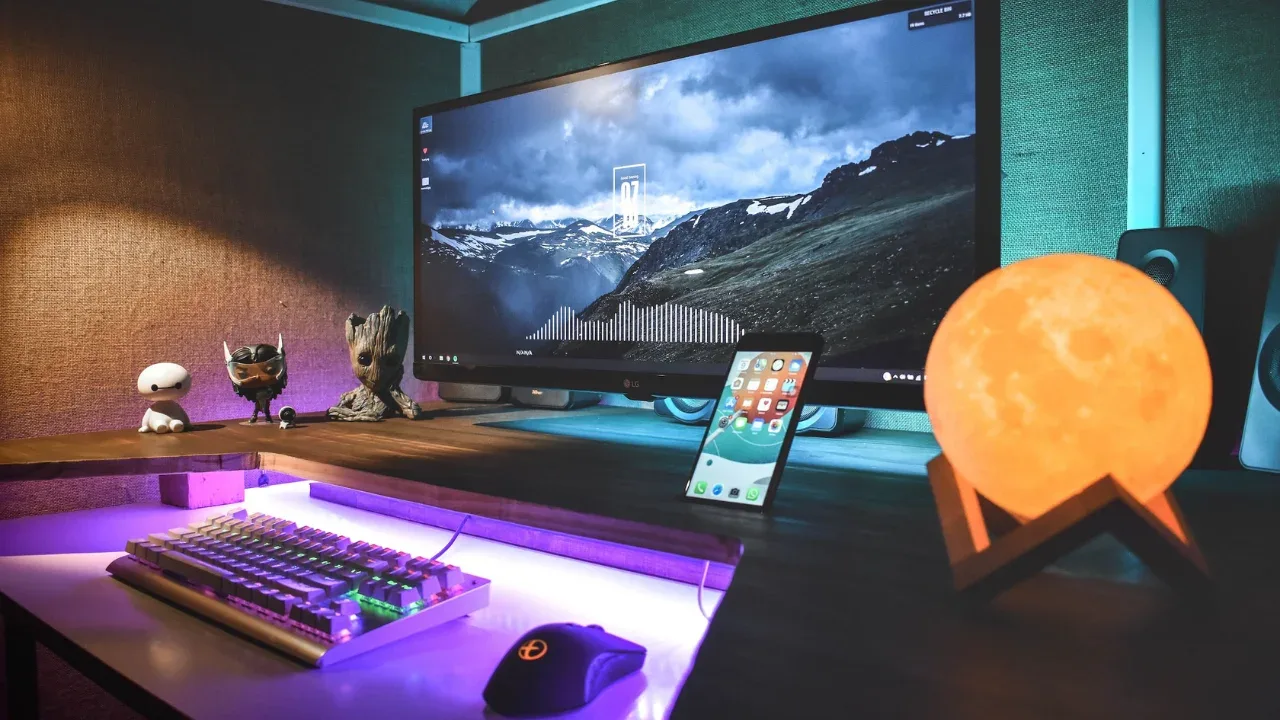
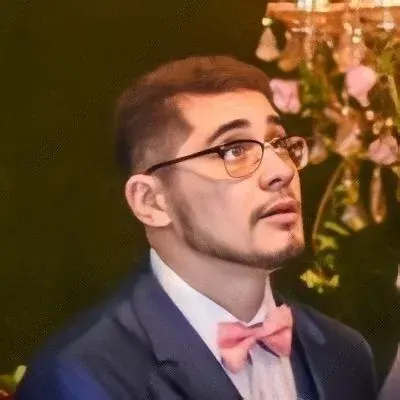
Send POST data using XMLHttpRequest
Are you looking to send data using an XMLHttpRequest
in JavaScript? Look no further! In this guide, we'll walk you through the process, address common issues, and provide easy solutions. Let's get started! 💪
The Scenario 📝
Before we dive into the solution, let's set up the scenario. Assume you have a form in HTML with several hidden fields:
<form name="inputform" action="somewhere" method="post">
<input type="hidden" value="person" name="user">
<input type="hidden" value="password" name="pwd">
<input type="hidden" value="place" name="organization">
<input type="hidden" value="key" name="requiredkey">
</form>
Your goal is to send this form data using XMLHttpRequest
in JavaScript.
The Solution 💡
To achieve this, follow these simple steps:
Create an instance of
XMLHttpRequest
by calling the constructor:const xhr = new XMLHttpRequest();
Open the connection by specifying the method (
POST
) and the URL you want to send the data to:xhr.open('POST', 'your-url-goes-here');
Set the request header to indicate that you are sending form data:
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
Create a variable to hold your form data in the desired format. In this case, we'll use the
FormData
object to automatically serialize the form fields:const formData = new FormData(document.forms.inputform);
Send the form data as the body of the request:
xhr.send(formData);
That's it! Your data will now be sent using the XMLHttpRequest
. 🚀
Common Issues and Solutions 🐛
1. CORS Errors
If you encounter CORS (Cross-Origin Resource Sharing) errors, it means that the destination URL does not allow requests from your domain. You can either configure the server to accept your requests or use a proxy to bypass the CORS restrictions.
2. Handling Server Responses
To handle the response from the server, you can listen for the load
event on the XMLHttpRequest
object. This event will fire when the response is successfully received. You can access the response data using xhr.responseText
or xhr.response
.
Take it a Step Further! ✨
Now that you know how to send POST data using XMLHttpRequest
, why not try implementing it in your own project? Experiment, explore, and have fun!
If you have any questions or want to share your experience, leave a comment below. We'd love to hear from you! 😊
Remember, keep coding and stay curious! 🚀