Selecting last element in JavaScript array
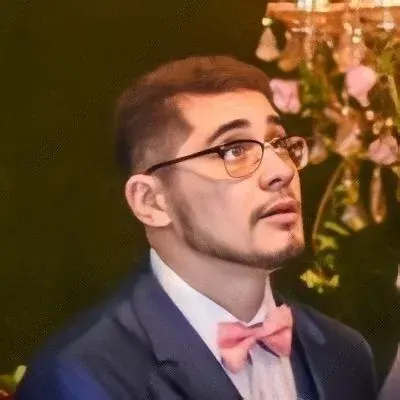
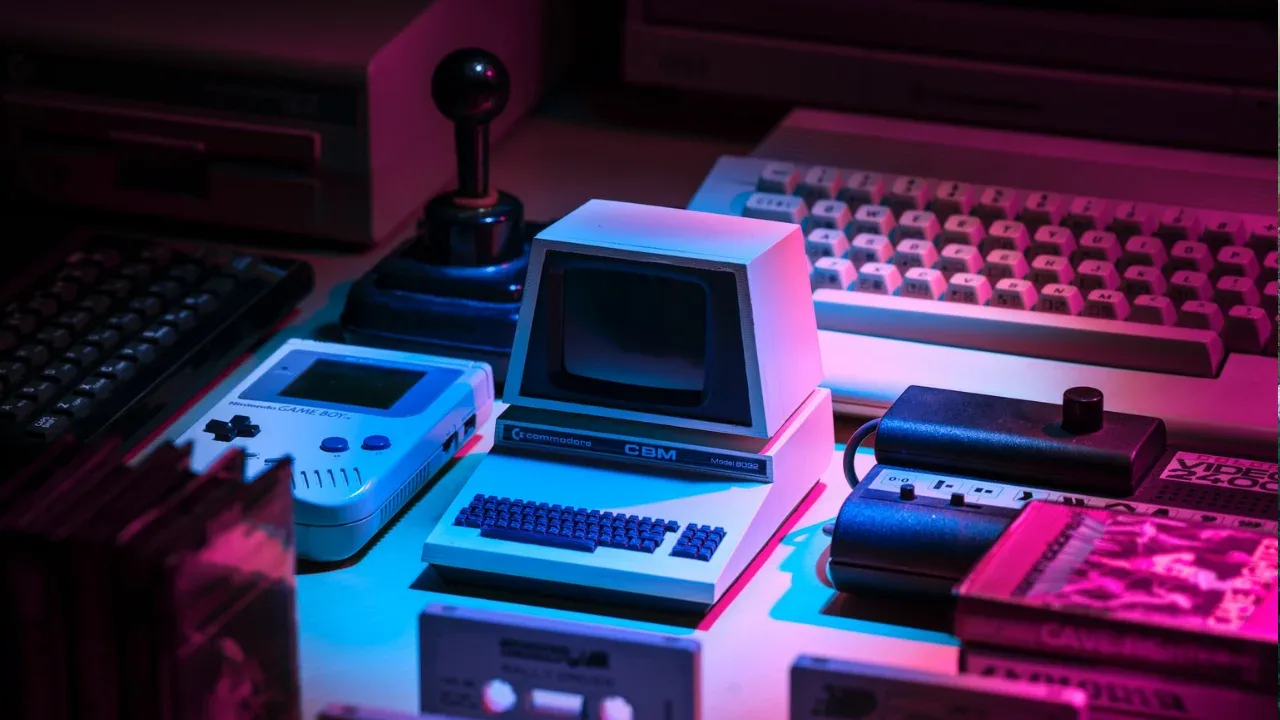
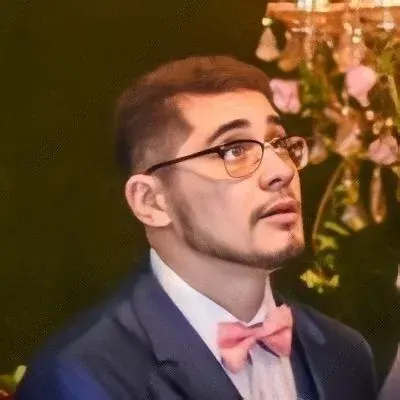
Selecting the Last Element in a JavaScript Array
š Hey there, fellow developer! Have you ever wondered how to select the last element in a JavaScript array? š¤ If you've been struggling with this problem, you're in the right place! In this blog post, we'll address the common issues around selecting the last element and provide you with easy solutions.
The Challenge
Let's begin with some context. Imagine you're working on an exciting application that tracks the location and path of multiple users in real time. šš„ Each user's path is stored as an array of coordinates, represented by their unique ID as the key in an object.
For example, here's a snippet of the data structure you might be working with:
loc = {
'f096012e-2497-485d-8adb-7ec0b9352c52': [
new google.maps.LatLng(39, -86),
new google.maps.LatLng(38, -87),
new google.maps.LatLng(37, -88)
],
'44ed0662-1a9e-4c0e-9920-106258dcc3e7': [
new google.maps.LatLng(40, -83),
new google.maps.LatLng(41, -82),
new google.maps.LatLng(42, -81)
]
};
ā ļø Now, the challenge arises when you want to update the marker's location each time the location changes for a specific user. You want to select the last element in the user's array of coordinates to update the marker accordingly.
The Solution
To select the last element in a JavaScript array, you can use different methods like array[array.length-1]
or array.slice(-1)[0]
. Let's break them down:
Method 1: Using Array Length
const userCoordinates = loc['f096012e-2497-485d-8adb-7ec0b9352c52']; // Get the array for a specific user
const lastCoordinate = userCoordinates[userCoordinates.length - 1]; // Select the last element
// Example usage:
console.log(lastCoordinate); // Returns [new google.maps.LatLng(37, -88)]
⨠By accessing the array using userCoordinates.length - 1
, you can easily grab the last element.
Method 2: Using Array Slice
Alternatively, you can also use the array.slice(-1)[0]
method to achieve the same result:
const userCoordinates = loc['f096012e-2497-485d-8adb-7ec0b9352c52']; // Get the array for a specific user
const lastCoordinate = userCoordinates.slice(-1)[0]; // Select the last element
// Example usage:
console.log(lastCoordinate); // Returns [new google.maps.LatLng(37, -88)]
⨠Using array.slice(-1)[0]
, you can select the last element of the array directly.
š Your Turn!
Now that you know how to select the last element in a JavaScript array, it's time to apply this knowledge to your own projects! š Experiment with the provided code examples and integrate it into your application's logic.
Feel free to share your experiences, questions, or any other thoughts in the comments section below! Let's engage in a lively discussion and learn from each other. š¬š
Happy coding! š»š