Selecting and manipulating CSS pseudo-elements such as ::before and ::after using JavaScript
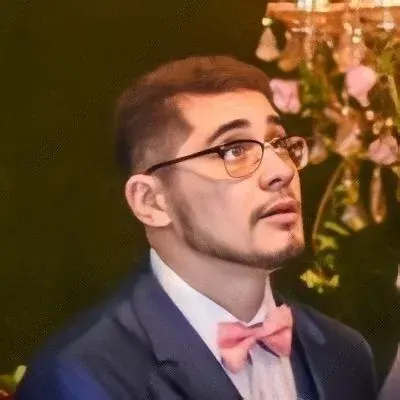
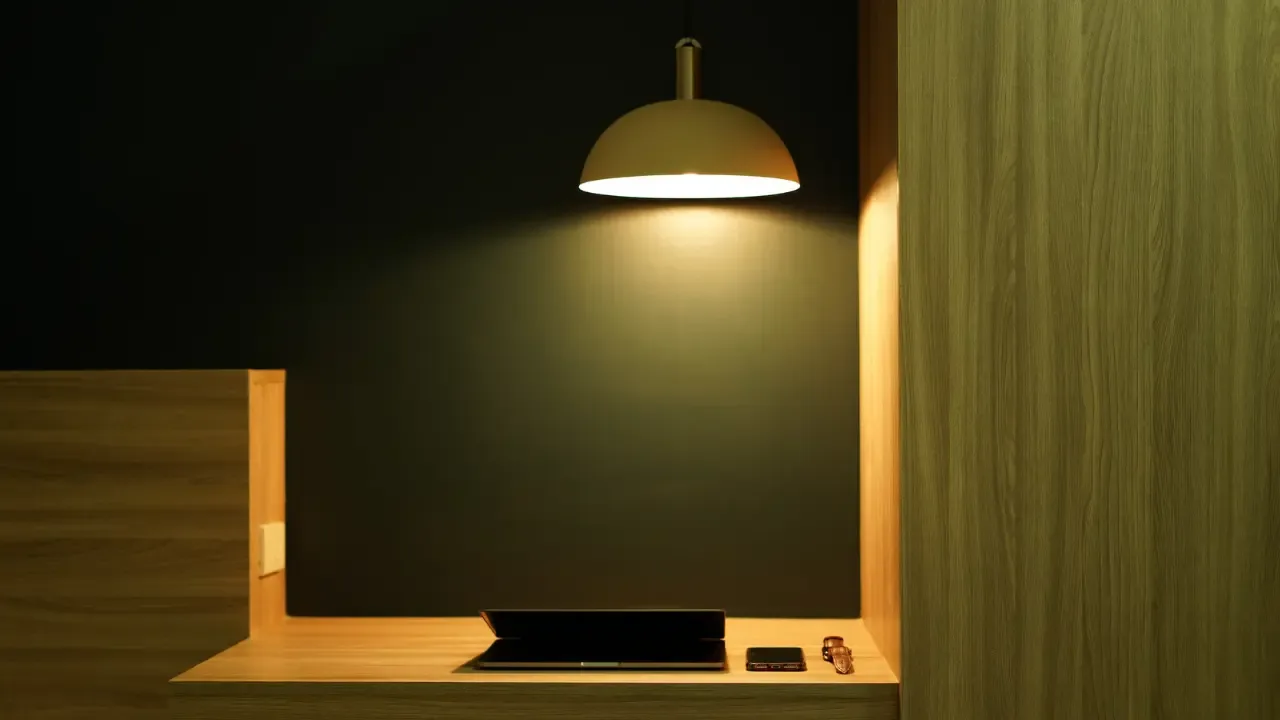
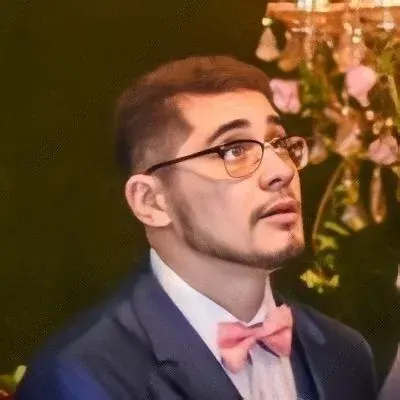
Selecting and Manipulating CSS Pseudo-elements using JavaScript
š¤ Have you ever wondered if it's possible to select and manipulate CSS pseudo-elements, such as ::before
and ::after
, using JavaScript? Well, you're in luck because we have easy solutions for you! š
Understanding the Problem
š First, let's understand the problem. The question posed revolves around changing the content of a pseudo-element using vanilla JavaScript or jQuery. Here's an example stylesheet rule:
.span::after {
content: 'foo';
}
The goal is to change 'foo' to 'bar'. So, how can we achieve this?
Solution #1: Vanilla JavaScript
š¤ Using vanilla JavaScript, it's fairly straightforward to select and manipulate pseudo-elements. Here's how you can achieve it:
const spanAfter = document.querySelector('.span::after');
spanAfter.style.content = "'bar'";
In the code snippet above, we select the ::after
pseudo-element by targeting the parent element with the class .span
. Then, we directly modify its content
property to 'bar'
. Easy peasy! š
Solution #2: jQuery
𤩠If you prefer using the popular jQuery library, fear not! We have a solution for you too. The process is similar to the vanilla JavaScript approach. Here's how you can do it:
$('.span::after').css('content', "'bar'");
In this example, we use the familiar syntax of jQuery to select the ::after
pseudo-element. We then utilize the .css()
method to modify its content
property to 'bar'
.
Wrapping Up
š You've now learned two easy ways to select and manipulate CSS pseudo-elements (::before
and ::after
) using vanilla JavaScript and jQuery. It's always good to have options! š
Remember, you can choose the approach that suits your coding style or aligns with your project requirements. Whether you prefer vanilla JavaScript or jQuery, both methods will help you accomplish the desired outcome.
Feel free to experiment and apply these techniques to your own projects. If you have any questions or other CSS-related problems, don't hesitate to reach out. We're always here to help you out! š
Your Turn!
š Now it's your turn to flex your coding skills. Try implementing the solutions on your own and see the magic happen! Don't forget to share your experience and any interesting findings in the comments section below.
Let's dive in and become pseudo-element masters together! šŖš»