SecurityError: Blocked a frame with origin from accessing a cross-origin frame
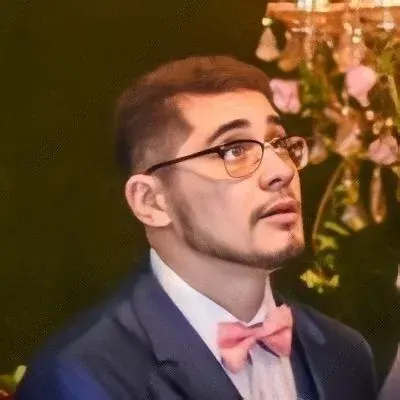
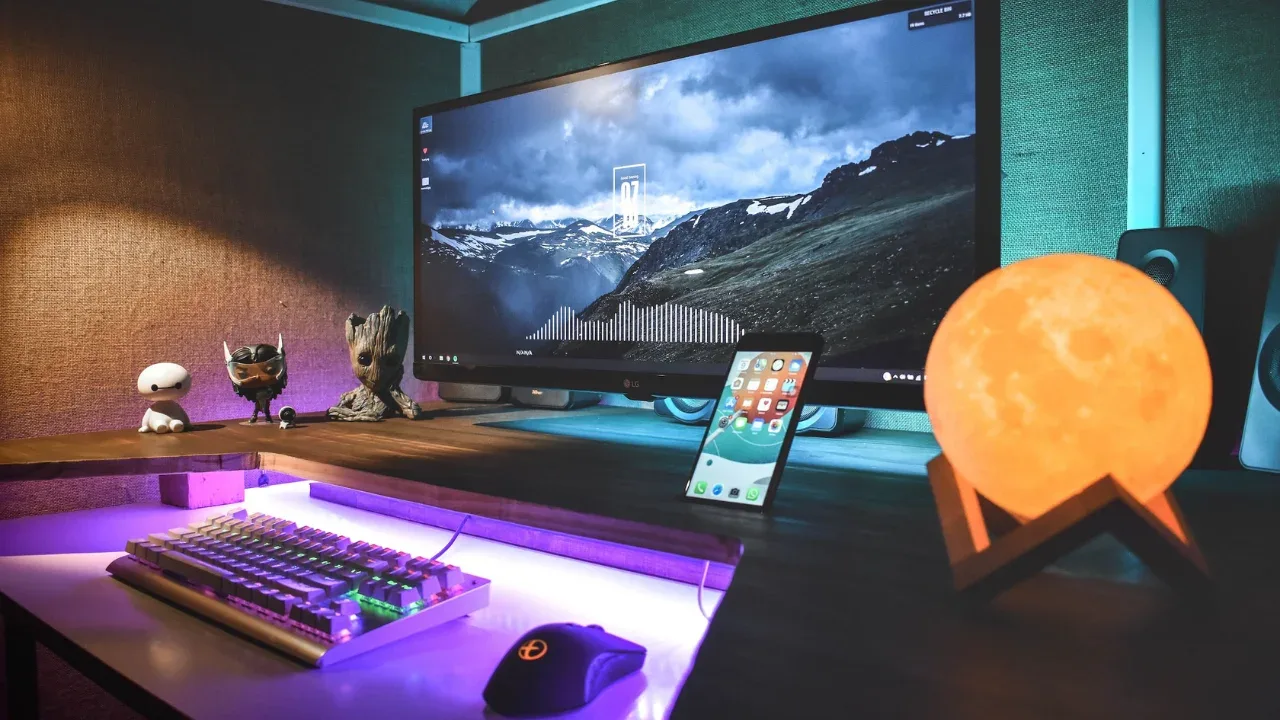
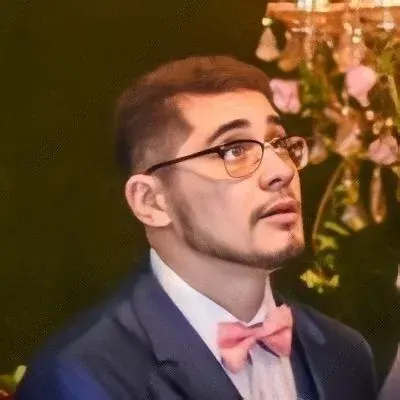
Solving the SecurityError: Blocked a frame with origin from accessing a cross-origin frame
Are you trying to access elements within an <iframe>
using JavaScript, but encountering the dreaded "SecurityError: Blocked a frame with origin from accessing a cross-origin frame" error? Don't fret, you're not alone! This error is a common issue that web developers face when working with cross-origin frames and can be easily resolved. In this blog post, we will delve into the problem, provide you with simple solutions, and help you get back on track with your project. 🚀
Understanding the Error
The error message "SecurityError: Blocked a frame with origin from accessing a cross-origin frame" occurs when you're attempting to access elements within an <iframe>
that originates from a different domain (origin) than the parent page. This restriction exists to prevent malicious scripts from accessing sensitive information across different domains. This security measure is commonly known as the Same-Origin Policy.
The Same-Origin Policy allows scripts on a page to only interact with resources from the same origin. An origin consists of the scheme (e.g., http, https), domain, and port. If any of these elements differ between the parent page and the <iframe>
, you'll encounter the cross-origin error.
Solutions to Access Cross-Origin Frames
1. Enable Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS) is a mechanism that allows controlled access to resources from a different origin. To enable CORS, you need to make changes on both the parent page and the server hosting the <iframe>
.
On the server hosting the <iframe>
, you must add the appropriate CORS headers to the response. These headers include Access-Control-Allow-Origin
, Access-Control-Allow-Methods
, Access-Control-Allow-Headers
, and possibly others depending on your use case. Check the documentation of your server framework or hosting service for specific instructions.
On the parent page, you can specify a wildcard *
to allow access from any origin or specify the exact origin. Here's an example of enabling CORS on the parent page:
const myIframe = document.getElementById("my-iframe-id");
myIframe.contentWindow.postMessage("AccessGranted", "http://www.example.com");
Remember to replace "http://www.example.com"
with the actual origin of your <iframe>
. This code snippet uses the postMessage()
method to send a message to the <iframe>
and establish communication between the parent and child windows.
2. Proxying the Request
If you don't have control over the server hosting the <iframe>
and enabling CORS is not an option, you can use a proxy to bypass the same-origin policy. A proxy acts as an intermediary between your parent page and the <iframe>
, forwarding requests and responses.
To implement this solution, you'll need to set up a server-side script that acts as a proxy and performs the request on behalf of the parent page. The script receives the request from the parent page, forwards it to the <iframe>
's server, and relays the response back to the parent page. This way, the request appears to originate from the same domain as the parent page, bypassing the cross-origin restriction.
3. Use the HTML5 Sandbox Attribute
Another option to consider is to leverage the HTML5 sandbox
attribute on the <iframe>
tag. The sandbox
attribute places restrictions on what the <iframe>
can do, but it allows you to access its content programmatically.
To use the sandbox
attribute, add it to the <iframe>
tag and specify the desired restrictions. Here's an example:
<iframe src="http://www.example.com/myframe.html" sandbox="allow-scripts"></iframe>
The sandbox
attribute with the value "allow-scripts"
grants permission to script within the <iframe>
. This enables you to access its elements using JavaScript while still adhering to the same-origin policy.
Conclusion
While the "SecurityError: Blocked a frame with origin from accessing a cross-origin frame" error may seem intimidating at first, it can be resolved with the right approach. Enabling CORS, proxying requests, or using the sandbox
attribute are effective solutions to access elements within cross-origin frames.
Remember, when working with cross-origin frames, web browsers enforce the same-origin policy for security purposes. By implementing the appropriate techniques, you can overcome this limitation and interact with the content of your <iframe>
seamlessly.
Now that you have solutions to this common problem, go ahead and implement them in your code. Happy coding! 😄👩💻👨💻
Did you find this article helpful in understanding and solving the "SecurityError: Blocked a frame with origin from accessing a cross-origin frame" issue? Share your thoughts and experiences in the comments below. Let's tackle this challenge together! 💬🌟
Stay connected! Follow our blog for more useful guides and tips on web development. Subscribe to our newsletter to receive updates straight to your inbox. And don't forget to share this article with your fellow developers and colleagues. Spread the knowledge! 📚🔗💡