Scroll to bottom of div?
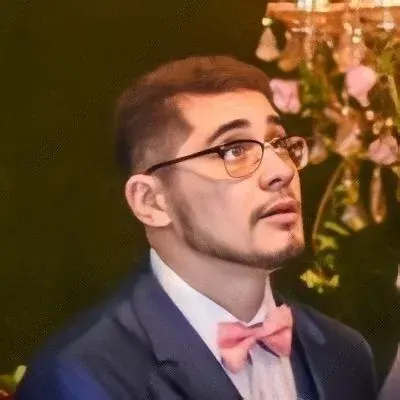
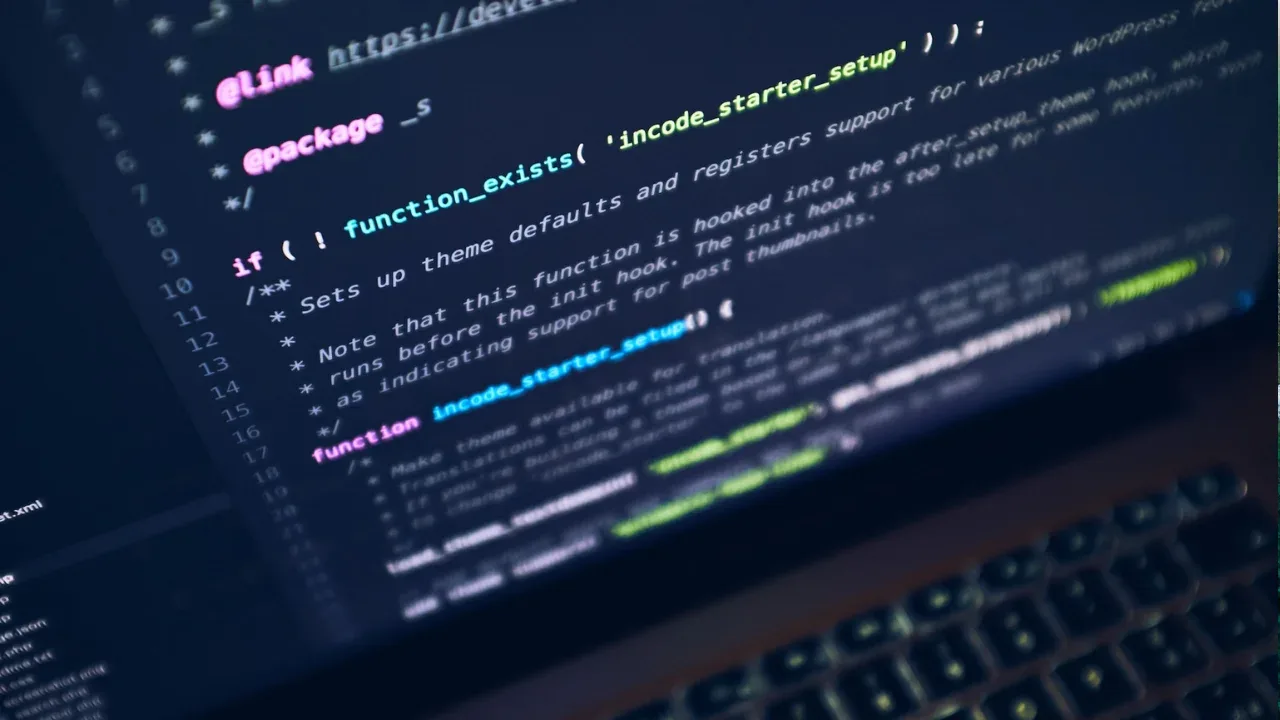
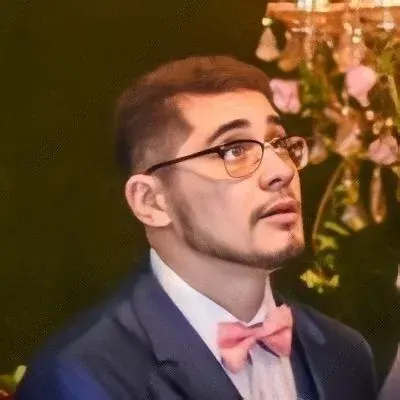
How to Scroll to the Bottom of a Div Using JavaScript
Are you struggling to make a chat application with a scrolling messages div? Do you want the messages div to automatically scroll to the bottom, both by default and after an AJAX request? Look no further! We've got you covered with some simple JavaScript techniques. 😎
The Problem: The Scroll Doesn't Go to the Bottom
You mentioned that you already have a designated div for your chat messages, and you've set its height and overflow properties. However, the scroll doesn't automatically go to the bottom as you'd like. Let's address this issue step by step.
Solution 1: Scroll to Bottom by Default
To ensure that the messages div is scrolled to the bottom by default, even when the page loads or refreshes, you can utilize JavaScript in combination with the div's scrollHeight property. Here's an example:
const messagesDiv = document.getElementById('scroll');
messagesDiv.scrollTop = messagesDiv.scrollHeight;
In this code snippet, we first retrieve the messages div using its ID. Then, we set the scrollTop property, which determines the position of the scrollbar, to the value of scrollHeight. The scrollHeight property represents the entire height of the element.
By applying this code when the page loads, the messages div will automatically scroll to the bottom, making the latest messages visible to the user.
Solution 2: Scroll to Bottom After AJAX Requests
If you want the messages div to scroll to the bottom after an AJAX request, you can extend the previous solution. Let's assume you have a function called loadMessages()
that fetches and appends new chat messages to the div:
function loadMessages() {
// AJAX request to fetch new messages and append them to the messages div
// Scroll to the bottom after appending new messages
messagesDiv.scrollTop = messagesDiv.scrollHeight;
}
By adding the messagesDiv.scrollTop = messagesDiv.scrollHeight;
line after the AJAX request completes and new messages are appended, the messages div will automatically scroll to the bottom. This way, users can see the latest incoming or sent messages without manually scrolling.
Conclusion and Call-to-Action
Congratulations! You now have the knowledge to make your chat application scroll to the bottom of the messages div effortlessly. Apply the "Scroll to Bottom by Default" solution upon page load and the "Scroll to Bottom After AJAX Requests" solution within your AJAX callback functions.
If you found this guide helpful, feel free to share it with others who might benefit from it. Also, we'd love to hear your feedback and answer any questions you might have. So don't hesitate to drop a comment below or reach out to us on social media. Happy scrolling! ✌️