Scroll to an element with jQuery
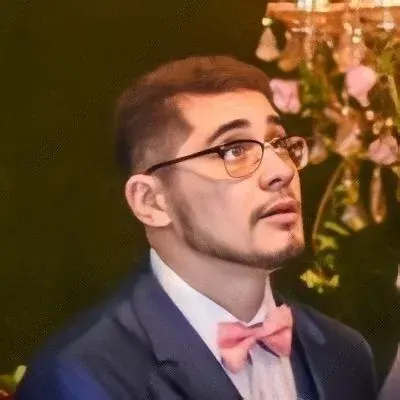
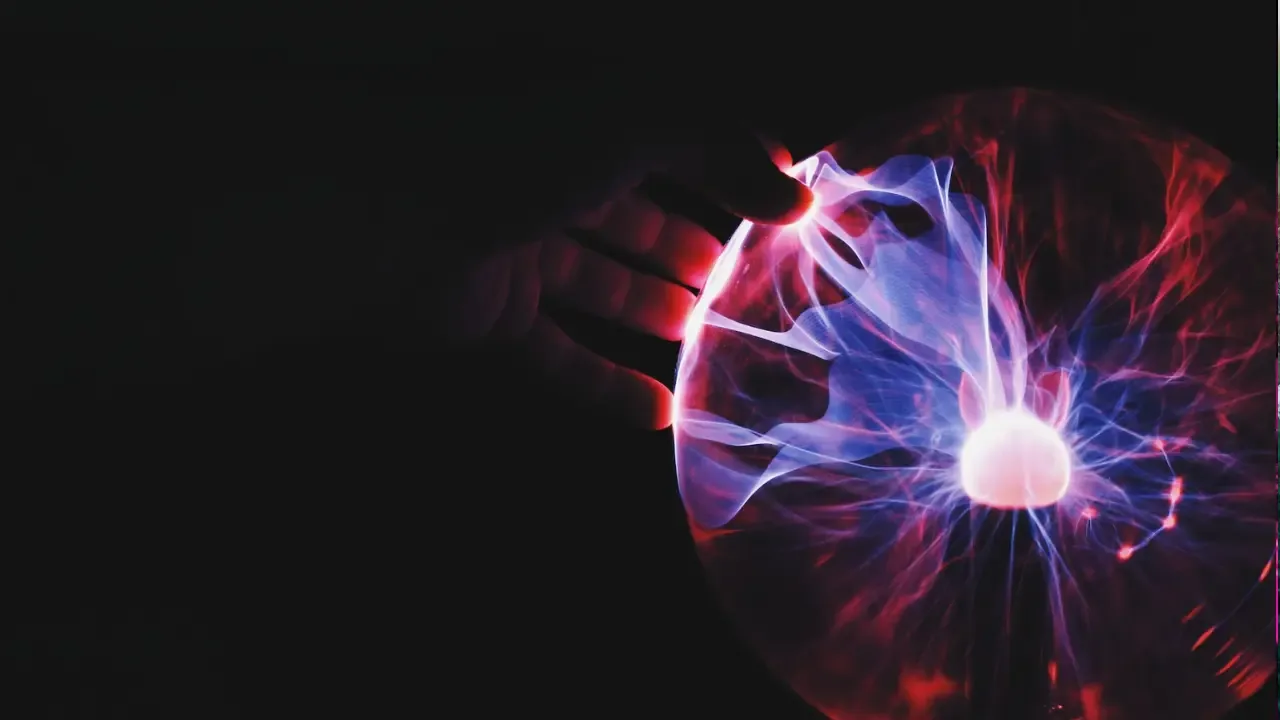
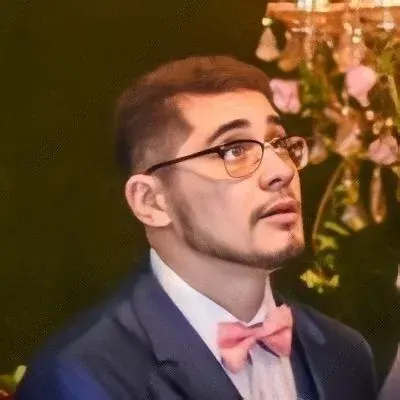
A Complete Guide: How to Scroll to an Element with jQuery 📜✨
Scrolling to an element on a web page can enhance the user experience and provide a smooth navigation flow. In this guide, we'll explore how to scroll to an element using jQuery. We'll address common issues, provide easy solutions, and give you the tools you need to implement this feature with a touch of finesse. Let's dive in! 💻🚀
The Setup 🔧
To begin, let's assume you have an HTML page with various elements, including an <input>
element with the id subject
and a submit button with the id submit
. Our goal is to make the page scroll smoothly to the submit
button when the user clicks on the subject
input field. We'll achieve this using jQuery's built-in features and without the need for any additional plugins.
Solution Steps 💡
Step 1: Include the jQuery Library 📚
First and foremost, make sure you have the latest version of jQuery included in your HTML file. You can either download it locally or use a CDN:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 2: Implement the Scroll Functionality ⬇️
To scroll to the desired element smoothly, we'll use jQuery's .animate()
method. This method allows us to create animations in a fluid and controlled manner. Here's an example of how to implement the scroll functionality:
$(document).ready(function() {
$('#subject').click(function() {
$('html, body').animate({
scrollTop: $('#submit').offset().top
}, 1000); // Adjust the duration (in milliseconds) as needed
});
});
In the above code snippet, we attach a click
event listener to the subject
input field using $('#subject').click()
. When the input field is clicked, the animation begins. The scrollTop
property is set to the top offset of the submit
button ($('#submit').offset().top
), ensuring that the page scrolls to the button. The duration of the animation is set to 1000
milliseconds, but feel free to adjust it to suit your needs.
Step 3: Test and Iterate ✅🔄
Once you've implemented the code, test it by clicking on the subject
input field. The page should smoothly scroll to the submit
button with the desired animation. If you encounter any issues, double-check that you've followed the steps correctly and review your code for any potential mistakes.
Conclusion and Call-to-Action 🎉🔗
Congratulations! You've successfully learned how to scroll to an element using jQuery. By incorporating this feature into your web pages, you can enhance the user experience and create a more fluid navigation flow. Feel free to experiment with the code and adapt it to your specific needs.
If you found this guide helpful, don't forget to share it with your friends and colleagues. Have you used jQuery to implement scroll functionality on your website? Share your experiences and tips in the comments below. Let's keep the conversation going! 👇😊