Scroll Automatically to the Bottom of the Page
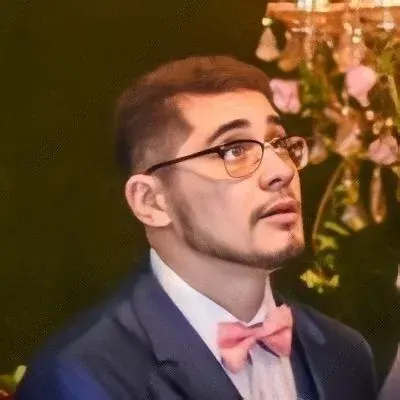
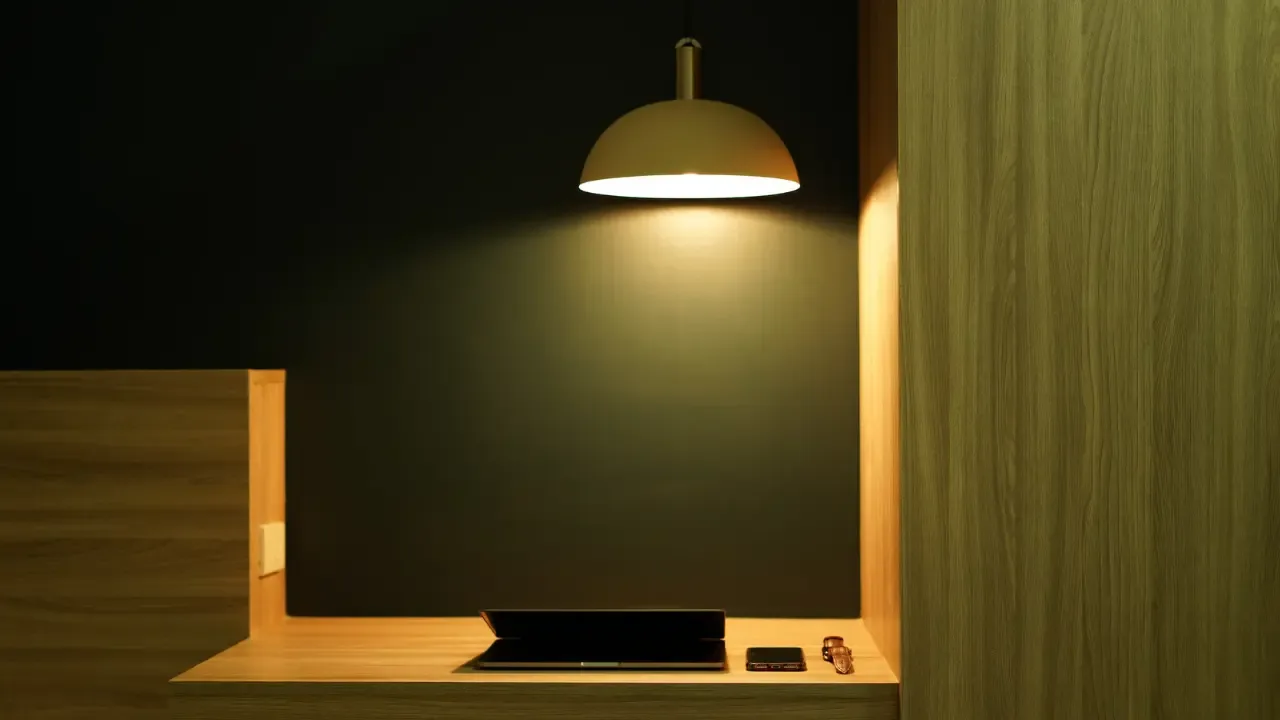
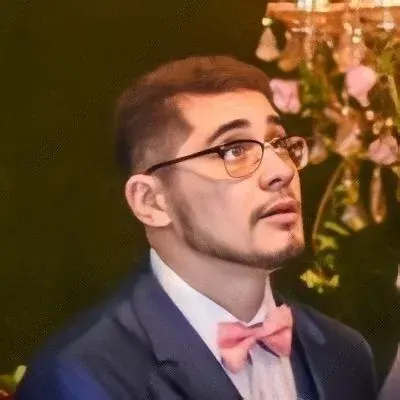
Scrolling Automatically to the Bottom of the Page: Easy Solutions with jQuery! ✨🚀
So, you're working on a page with a list of questions and you want the page to automatically scroll down to a specific element when a question is clicked. Fear not, my tech-savvy friend! We've got you covered with some simple jQuery solutions that will make your page more user-friendly and engaging.
Common Hurdles: Why is this happening? ❓
Before we dive into the solutions, let's take a quick look at some common issues you might encounter when you try to achieve this automatic scrolling effect.
Losing reference to the target element: When you click on a question, the page needs to know which element to scroll to. Losing the reference to the target element can prevent the automatic scrolling from happening.
Smooth scrolling anomalies: You want the scrolling to be smooth and seamless, but sometimes you may experience jerky or unexpected behavior during the scroll animation.
The Magic jQuery Potion: Solutions Galore! 💫🔮
Now that we understand the challenges, let's explore some ways to overcome them using the power of jQuery. Below are two popular approaches to achieve the automatic scrolling effect you desire:
Solution 1: Using jQuery's animate()
Method
$(document).ready(function() {
$(".question").click(function() {
const targetElement = $($(this).attr("data-scroll-to")); // Extract the target element from the clicked question
$("html, body").animate({
scrollTop: targetElement.offset().top // Scroll to the top position of the target element
}, 1000); // Adjust the duration of scroll animation as needed
});
});
In this solution, we use jQuery's handy animate()
method to smoothly scroll the webpage to the top position of the target element. The data-scroll-to
attribute is used to store the ID of the target element in the question. When a question is clicked, we extract the target element ID and scroll to it.
Solution 2: Utilizing jQuery's scrollTop()
Method
$(document).ready(function() {
$(".question").click(function() {
const targetElement = $($(this).attr("data-scroll-to")); // Extract the target element from the clicked question
$("html, body").scrollTop(targetElement.offset().top); // Scroll to the top position of the target element
});
});
In Solution 2, we use jQuery's scrollTop()
method to instantly scroll the page to the top position of the target element. Note that this solution doesn't provide smooth scrolling animation like Solution 1, but it can still get the job done if you prefer instant scrolling.
Try It Yourself: Implement & Explore! 💪🔧
Now that you have both solutions at your disposal, feel free to try them out and see which one suits your needs better. Remember to replace the .question
class selector and the data-scroll-to
attribute with your own class and attribute names!
Additionally, don't forget to tweak the animation duration in Solution 1 (1000
milliseconds in the example). Adjust it to your desired speed to create an engaging user experience.
Share Your Success: Engage & Connect! 📢🤝
We hope these solutions have brought you a step closer to achieving automatic scrolling bliss on your webpage. Now, let's take it a step further and keep the conversation going! Share your experiences, ask questions, or even suggest your own solutions in the comments below. We can't wait to hear from you! 😊💬
So, until next time, happy scrolling and happy coding! Keep exploring and sharing the wonderful world of web development! 🌐💻✨