Safely turning a JSON string into an object
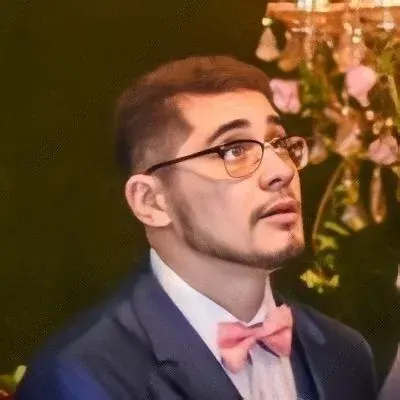
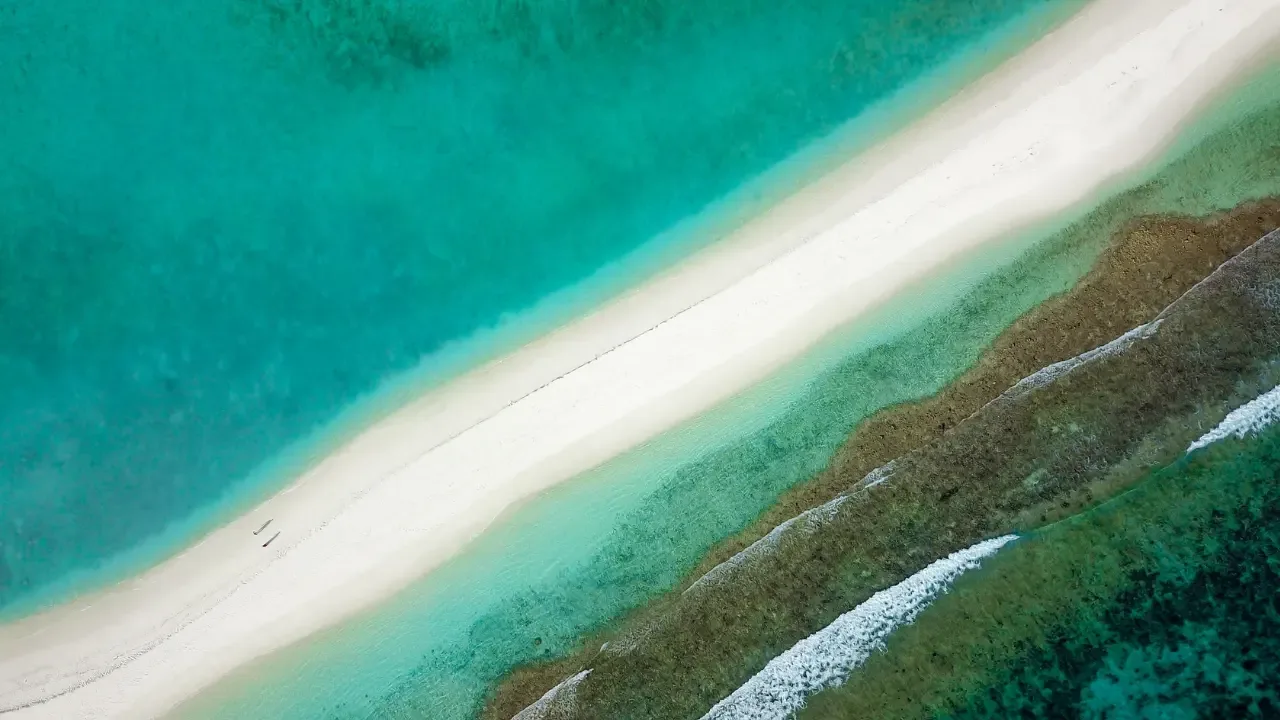
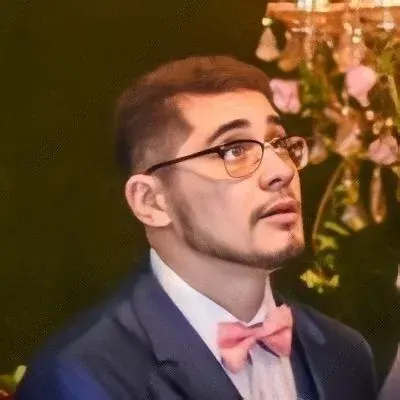
Safely turning a JSON string into an object: A Beginner's Guide 📝💡
So, you have a string of JSON data and you want to turn it into a JavaScript object. Easy peasy, right? Well, not so fast! While there are a few quick and dirty methods like using eval()
to achieve this, they can leave you vulnerable to potential security risks 😱. In this blog post, we'll explore the common issues and provide you with easy solutions for safely converting a JSON string into an object. Let's dive in! 🌊🏊♀️
The Danger of Using eval()
🤔🚫
Before we jump into the safer alternatives, let's take a moment to understand why using eval()
can be risky. When you use eval()
to parse a JSON string, you're essentially evaluating any code within that string. This means that if the JSON string contains malicious code, it can be executed in your application, opening the door to potential security vulnerabilities and nasty consequences. So, we definitely want to avoid that! 🙅♀️🔒
The Safe and Easy Solutions 🔐✔️
1. Using JSON.parse()
✨✅
JSON.parse()
is the go-to method for safely converting a JSON string into a JavaScript object. It parses the JSON data and returns a JavaScript object representing the JSON content. The best part? It doesn't execute any code, making it a secure choice.
Here's an example of how you can use JSON.parse()
:
var jsonString = '{"name":"John", "age":30, "city":"New York"}';
var obj = JSON.parse(jsonString);
console.log(obj.name); // Output: John
By using JSON.parse()
, you're ensuring that only valid JSON data is converted into an object, and all potential security risks are mitigated. It's simple, safe, and efficient! 🙌🔒
2. Libraries and Frameworks 💪📚
If you're working with larger or more complex JSON structures, it might be a good idea to leverage existing libraries or frameworks that provide additional features and security layers. Popular choices include jQuery's $.parseJSON()
or lodash's _.parseJSON()
. These tools not only handle the safe parsing of JSON, but also offer additional functionalities to make your life easier. Just make sure to include the relevant library or framework in your project.
Your Turn to Dive in Safely! 🚀💻
Now that you've learned about the potential dangers of using eval()
and the safe alternatives like JSON.parse()
and libraries, it's time to put your knowledge into action! The next time you encounter a JSON string that needs to be transformed into a JavaScript object, remember to choose the safe path. By doing so, you'll protect your application and your users from potential security threats.
Do you have any questions or other cool tips for working with JSON data? Share them in the comments below! Let's create a safe and thriving community of JSON enthusiasts! 🌟💬💙
Happy coding! 🎉💻