Run javascript function when user finishes typing instead of on key up?
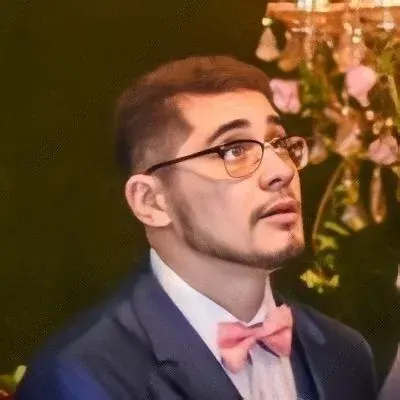
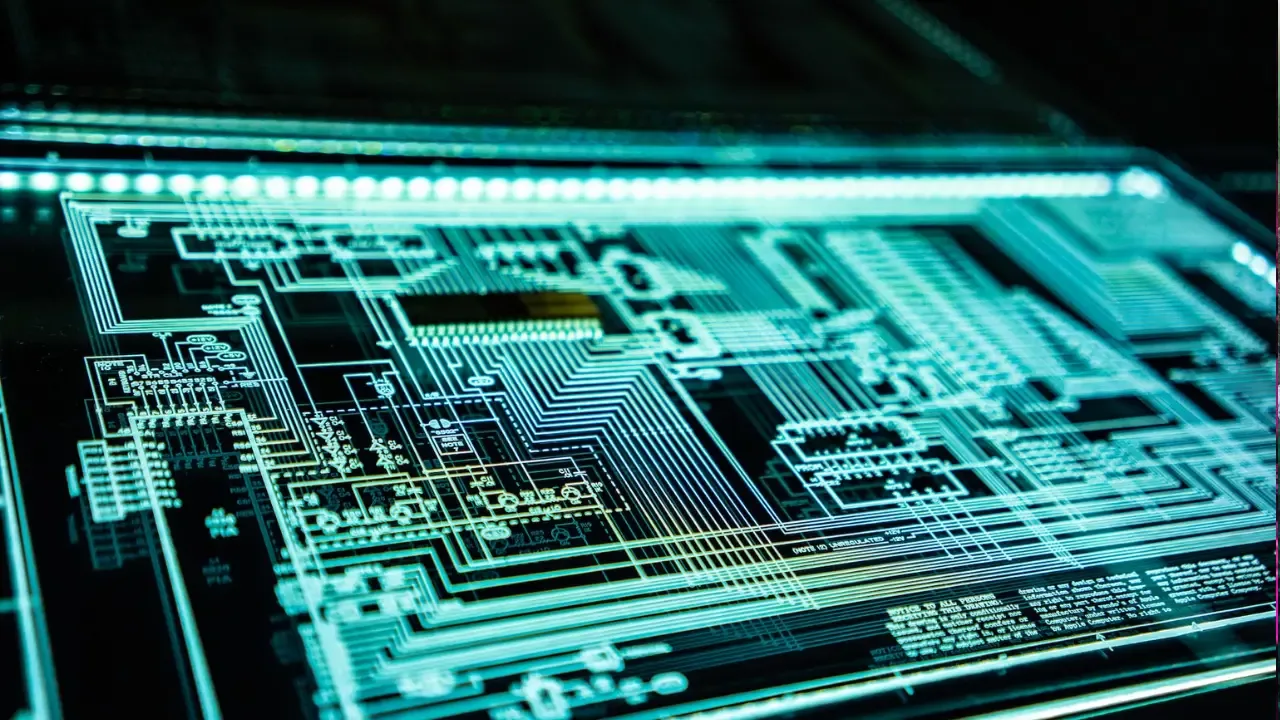
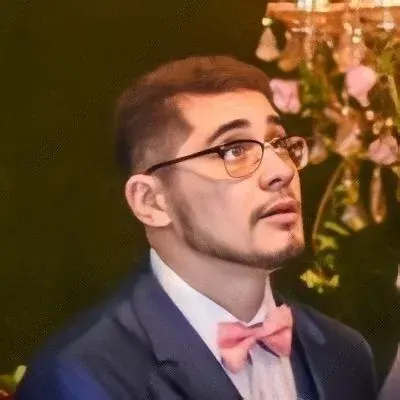
👋 Hey there! Are you tired of triggering an AJAX request every time a user types a single letter? 🤔 Don't worry, because I have a solution for you! 💡 In this blog post, we'll dive into how to run a JavaScript function when the user finishes typing instead of on key up. Let's get started! 🚀
The Problem
The issue here is that if we bind a function to the keyup
event, it will trigger every time a user types a letter, resulting in multiple AJAX requests 🔄. On the other hand, waiting for the user to hit the "Enter" key is not ideal either, as we want the request to happen dynamically as they type. So, what's the solution? 🤔
The Solution: Debouncing
The answer lies in using a technique called "debouncing." 🙌 Debouncing allows us to delay the execution of a function until the user has stopped typing for a specified amount of time. This way, we can avoid excessive AJAX requests while still providing a smooth user experience.
Implementing Debouncing with jQuery
Since you mentioned that you're using jQuery, I'll show you how to do it with this powerful library. Here's an example code snippet that demonstrates debouncing in action:
let timeout;
$('#yourTextBox').on('input', function() {
clearTimeout(timeout);
timeout = setTimeout(function() {
// Perform your AJAX request here
console.log('AJAX request triggered!');
}, 500); // Adjust the delay time as per your requirements
});
Explanation
In the code above, we attach an event listener to the input
event of your text box (replace #yourTextBox
with the appropriate selector). Whenever the user types or deletes something, the event is triggered.
Inside the event listener, we first clear any existing timeouts by calling clearTimeout(timeout)
. This ensures that the AJAX request is only triggered once the user has finished typing.
Then, we set a new timeout using setTimeout
. The function inside the timeout is where you can perform your AJAX request. Adjust the delay time (currently set to 500 milliseconds) according to your needs. Feel free to experiment with different values to find the sweet spot for your application.
That's it! You've successfully implemented debouncing with jQuery to trigger your AJAX request only when the user has finished typing.
Conclusion
By using the debouncing technique, you can save yourself from making unnecessary AJAX requests and improve the overall user experience 💁♂️. Incorporate this solution into your code, and your text box will trigger the AJAX request only after the user has stopped typing for a certain period of time 📝.
So go ahead, give it a try! Your server will thank you, and your users will appreciate the increased performance. If you have any questions or other cool tips to share, feel free to leave a comment below. Happy coding! 😊✨
📣 Call to Action: Have you struggled with excessive AJAX requests or other JavaScript woes? Check out our blog for more helpful tips and tricks! Don't forget to subscribe to stay up-to-date with the latest tech insights. Let's level up our coding skills together! 🚀🤩