Return an empty Observable
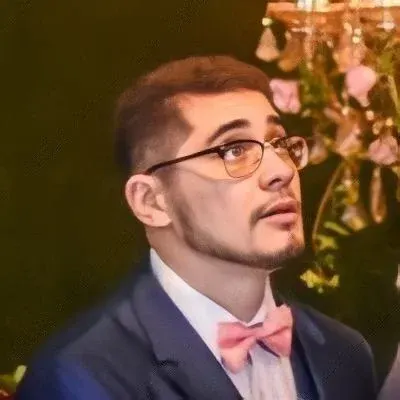
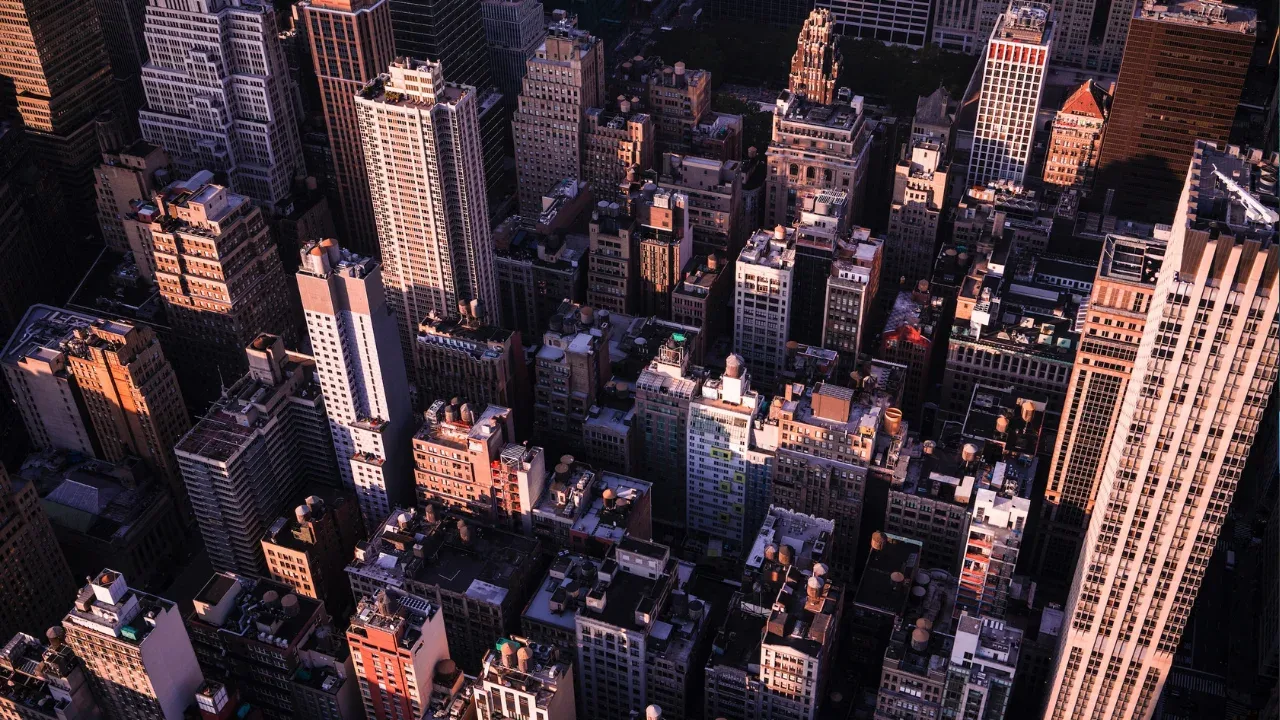
How to Return an Empty Observable in Typescript? 😕🔍
So, you are building an app, and your function more()
is supposed to return an Observable from a GET request. But there's a catch - you can only make the request if hasMore()
is true. Otherwise, you end up with an error when calling subscribe()
.
Don't worry! We've got you covered. In this guide, we'll show you an easy solution to this problem. Let's dive in! 🏊♂️💻
The Problem 😫
Here's your code snippet, where you're facing the issue:
export class Collection {
public more = (): Observable<Response> => {
if (this.hasMore()) {
return this.fetch();
} else {
// return empty observable
}
};
private fetch = (): Observable<Response> => {
return this.http.get("some-url").map((res) => {
return res.json();
});
};
}
As you can see, you want to return an empty Observable when hasMore()
is false. But what's the correct way to do it? Let's find out! 🤔
The Solution 🙌
To return an empty Observable, you can make use of the empty()
function provided by the rxjs
library. This function creates an Observable that immediately completes without emitting any values.
Here's how you can modify your code to return an empty Observable:
import { Observable, empty } from 'rxjs';
export class Collection {
public more = (): Observable<Response> => {
if (this.hasMore()) {
return this.fetch();
} else {
return empty();
}
};
private fetch = (): Observable<Response> => {
return this.http.get("some-url").map((res) => {
return res.json();
});
};
}
With this change, when hasMore()
is false, the more()
function will now return an empty Observable. This will prevent any errors when calling subscribe()
.
Wrapping Up 🎁
Returning an empty Observable in Typescript is not a hard problem when you know the right solution. By using the empty()
function from the rxjs
library, you can easily handle cases where you need to return an empty Observable.
We hope this guide helped you, and you're now able to resolve the issue in your app. Happy coding! 🚀👨💻
If you have any further questions or suggestions, feel free to drop them in the comments below. Let's keep the conversation going! 💬✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
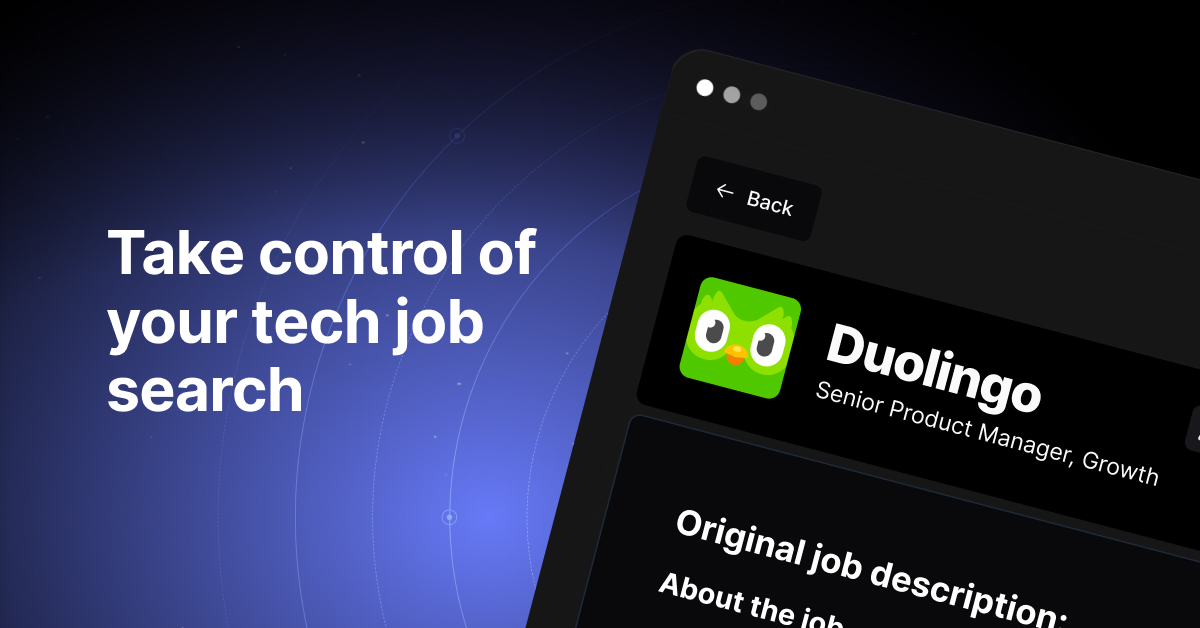