Retrieve the position (X,Y) of an HTML element
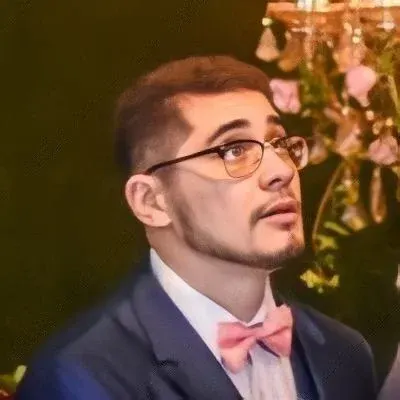
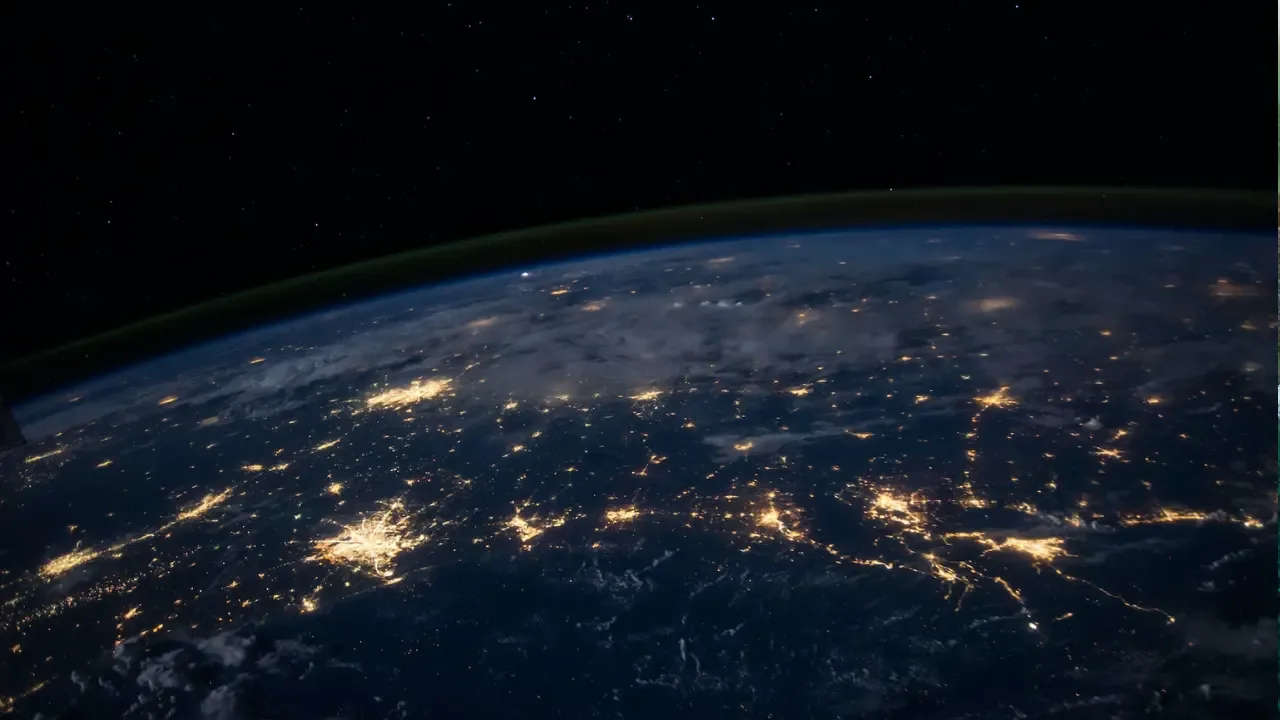
📝 Title: How to Retrieve the Position (X,Y) of an HTML Element in JavaScript
Hey there, tech enthusiasts! 🤗
Do you ever find yourself wondering how to get the position of an HTML element on a webpage? 🤔 Well, wonder no more! In this blog post, we'll dive into the world of JavaScript and uncover the secrets of retrieving the position (X,Y) of HTML elements like images and divs. 🌐📏
The Problem: 🔍
A lot of developers struggle with determining the exact position of an HTML element on a webpage. It can be a real pain, especially when you want to dynamically adjust or manipulate elements based on their position. But fear not, we've got you covered!
The Solution: 💡
To retrieve the position (X,Y) of an HTML element using JavaScript, we can utilize the getBoundingClientRect()
method. This handy method returns a DOMRect object with properties representing the position and size of the element. Let's see it in action with a code example: 🚀
const element = document.querySelector('.my-element');
const rect = element.getBoundingClientRect();
const positionX = rect.left + window.scrollX;
const positionY = rect.top + window.scrollY;
console.log(`X: ${positionX}px, Y: ${positionY}px`);
In the code snippet above, we first select the desired element using querySelector()
. We then call getBoundingClientRect()
on the element to obtain its position and store it in the rect
variable. By adding the window's scroll offsets, we can get the absolute position of the element on the page.
Common Issues: ⚠️
One common mistake is forgetting to include the window's scroll offsets. If your webpage has any vertical or horizontal scrolling, make sure to add window.scrollX
to the element's left
property and window.scrollY
to the element's top
property.
Another potential issue is when you have nested elements inside each other. In this case, make sure you're selecting the correct element to retrieve its position accurately.
Give It a Try! 💪
Now that you know how to retrieve the (X,Y) position of an HTML element, the possibilities are endless! You can use this knowledge to dynamically position elements, create interactive features, or even build custom scroll animations. Don't be afraid to explore and experiment!
🎉 Call-to-Action: Share Your Creations! 🎉
We'd love to see what you create using the position (X,Y) of HTML elements in JavaScript! Share your awesome projects, insights, or code snippets in the comments section below. Let's inspire and learn from each other! 💡🤩
That's a wrap, folks! 🎬 We hope this guide has helped demystify the process of retrieving the position (X,Y) of an HTML element using JavaScript. Remember, with a little bit of code and a lot of curiosity, you can achieve great things.
Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
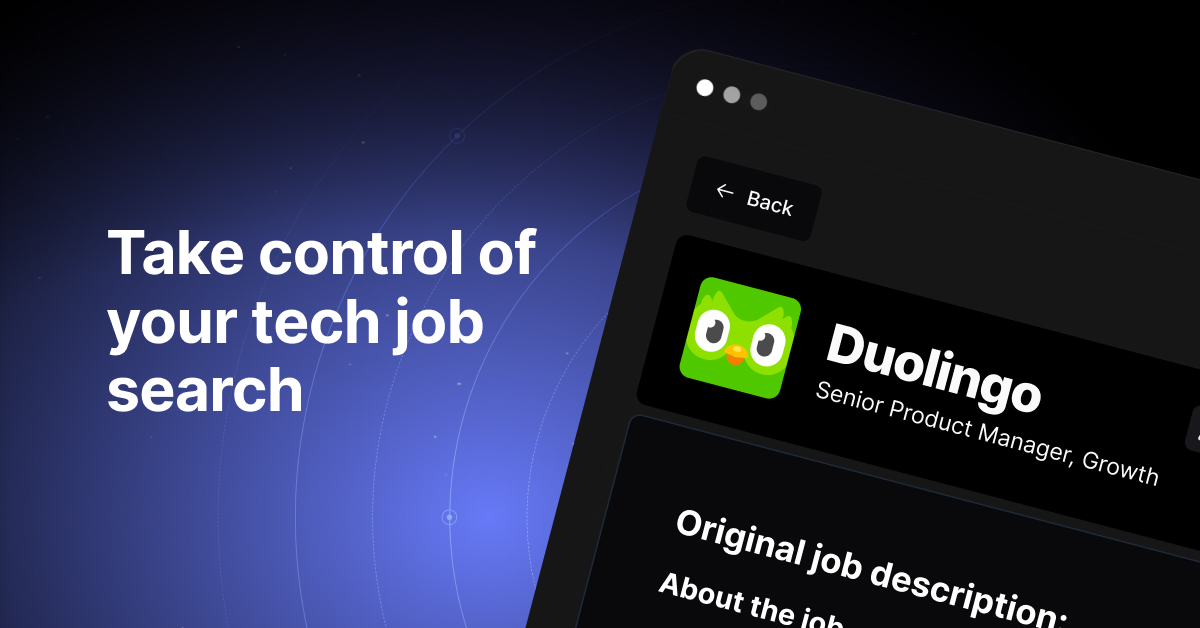