Rerender view on browser resize with React
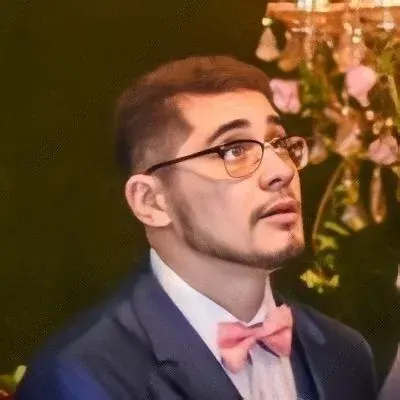
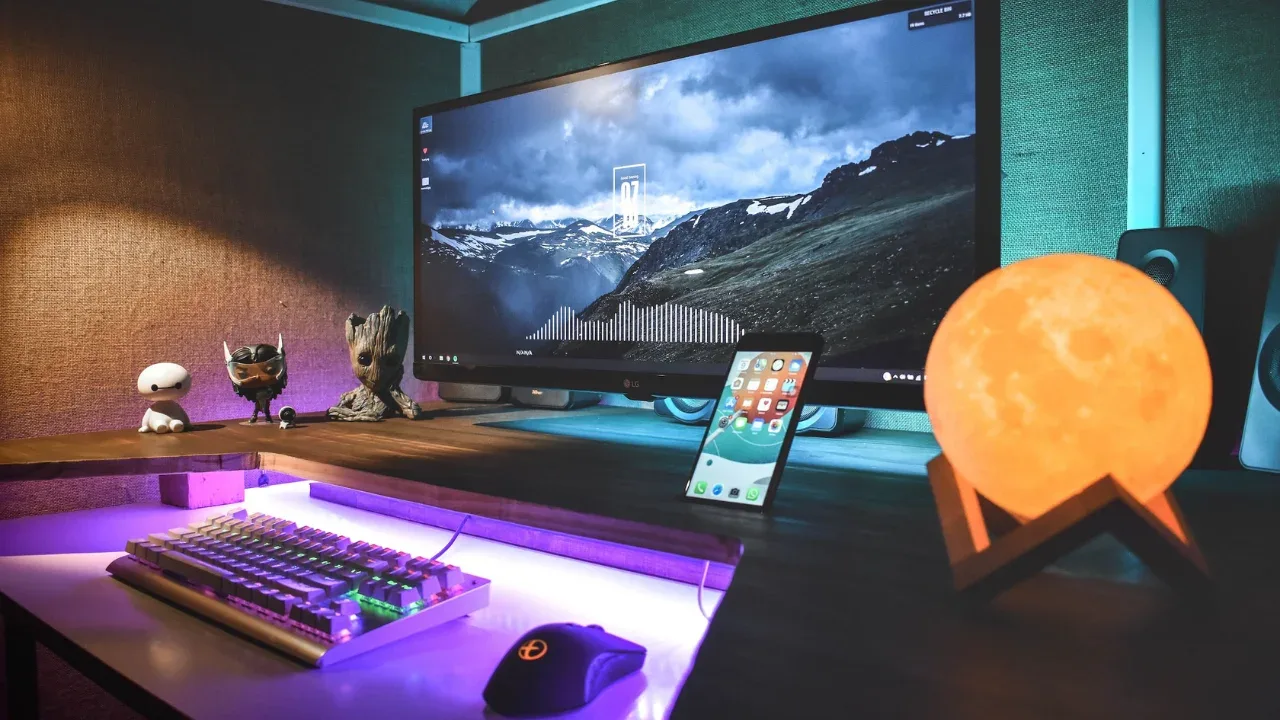
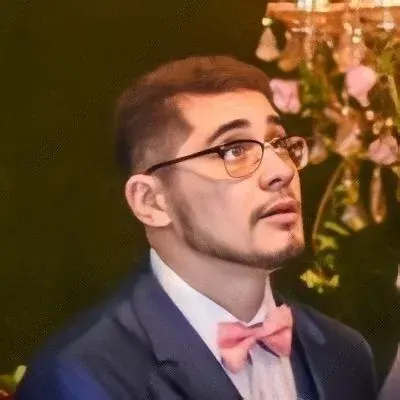
Rerender view on browser resize with React 🔄🖥️
Are you trying to make your React app re-render when the browser window is resized? Do you want your blocks to update and re-layout just like the Pinterest layout by Ben Holland? You're not alone! Many developers face this challenge when building dynamic layouts with React. In this blog post, we'll explore common issues and provide easy solutions to help you achieve the desired effect. Let's dive in! 💪
The Problem 🤔
The issue here is that React doesn't automatically re-render the view when the browser window is resized. By default, React only updates the view when there's a change in component state or props. This means that if you resize the browser window, the layout won't be updated accordingly.
The Solution ✅
Fortunately, there are multiple ways to solve this problem. Let's explore two approaches: one using jQuery's window resize event and another using a more "React" way.
1. Using jQuery's window resize event
One solution is to listen to the window resize event using jQuery and manually trigger a re-render of the component. Here's how you can do it:
componentDidMount() {
$(window).on('resize', this.handleResize);
}
componentWillUnmount() {
$(window).off('resize', this.handleResize);
}
handleResize() {
// re-render the component
this.forceUpdate();
}
In this approach, we add event listeners for the window resize event in the componentDidMount
lifecycle method and remove them in the componentWillUnmount
method to prevent memory leaks. When the window is resized, the handleResize
method is called, and we forcefully update the component by calling this.forceUpdate()
.
2. Using a more "React" way
To achieve a more "React" way of rerendering the view on browser resize, we can leverage React's built-in resize event listener provided by the ResizeObserver
API. This approach doesn't require any additional libraries like jQuery. Here's how you can implement it:
componentDidMount() {
this.resizeObserver = new ResizeObserver(this.handleResize);
this.resizeObserver.observe(window.document.body);
}
componentWillUnmount() {
this.resizeObserver.disconnect();
}
handleResize() {
// re-render the component
this.forceUpdate();
}
In this approach, we create a new instance of ResizeObserver
in the componentDidMount
method and pass the handleResize
method as the callback. We observe the window.document.body
element to listen for resize events. When the resize event is triggered, the handleResize
method is called, and we forcefully update the component using this.forceUpdate()
.
Conclusion and Call-to-Action ✍️🎉
Congratulations! 🎉 You now have two approaches to make React rerender the view when the browser window is resized. Whether you choose to use jQuery's window resize event or the more "React" way with ResizeObserver
, you can now achieve dynamic layouts that adapt to browser changes.
I hope this blog post helped you solve this common challenge in React development. If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 💻💙