Replace multiple strings with multiple other strings
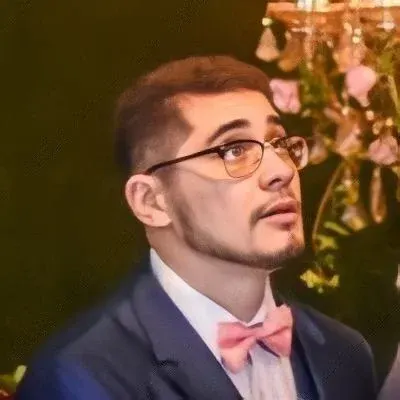
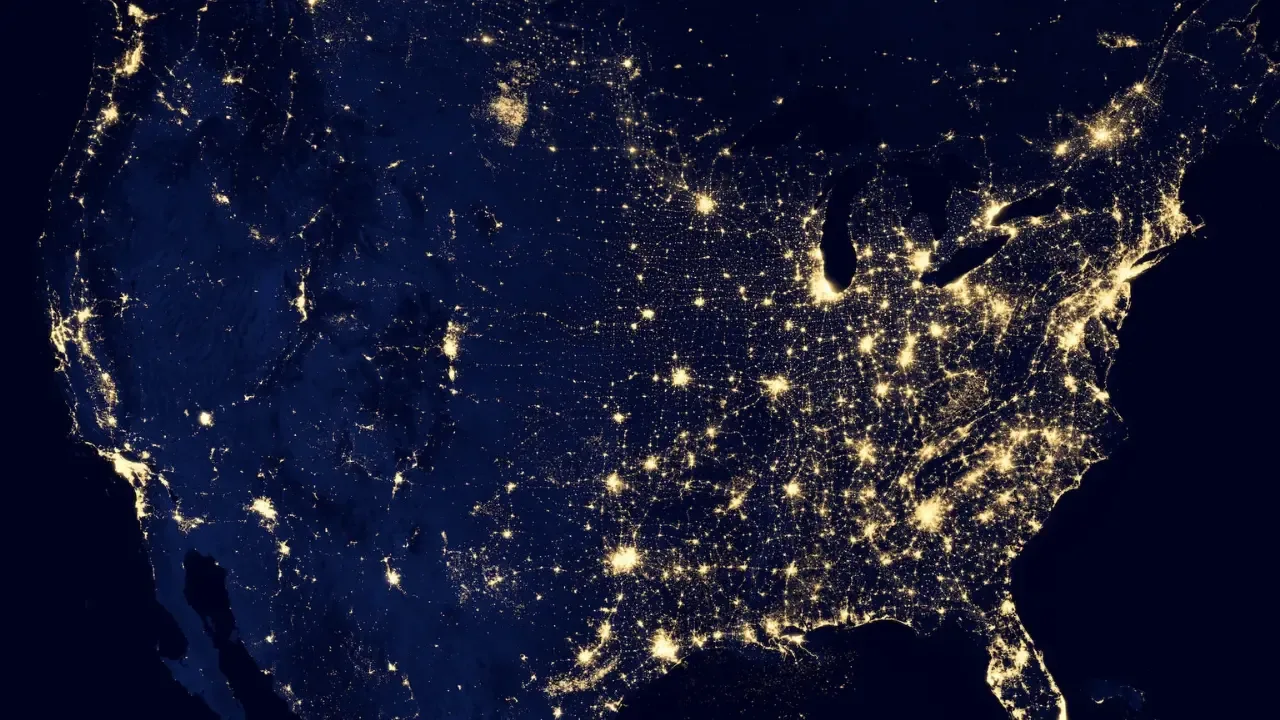
πΆππ Replace Multiple Strings with Multiple Other Strings in JavaScript: The Ultimate Guide πͺ
Are you tired of replacing multiple words in a string one by one and getting unexpected results? π€ Don't worry, I've got your back! In this comprehensive guide, I'll show you how to replace multiple strings with multiple other strings at the same time in JavaScript like a pro! π
πΎ The Problem
Let's start with the problem at hand. Imagine you have the following string: "I have a cat, a dog, and a goat." π±πΆπ And you want to replace "cat" with "dog", "dog" with "goat", and "goat" with "cat". But when you try the straightforward approach, using the replace()
method multiple times, you end up with unexpected results. ππ±
π The Mistaken Attempt
Here's the code snippet that produces the undesired outcome:
var str = "I have a cat, a dog, and a goat.";
str = str.replace(/cat/gi, "dog");
str = str.replace(/dog/gi, "goat");
str = str.replace(/goat/gi, "cat");
// This produces "I have a cat, a cat, and a cat"
// instead of "I have a dog, a goat, and a cat"
As you can see, instead of achieving the desired result, every string is being replaced with the string "cat". This happens because each replace()
method invocation works on the modified string, leading to unexpected substitutions. π΅
π οΈ The Solution
To replace multiple strings with multiple other strings simultaneously, we need to take a different approach. π Luckily, JavaScript provides us with a clever technique using a function as the second argument of the replace()
method. Let's dive into the solution step by step:
Replace each target word with a unique placeholder. Here's an updated version of the code snippet:
var str = "I have a cat, a dog, and a goat.";
str = str.replace(/cat/gi, "{%placeholder1%}");
str = str.replace(/dog/gi, "{%placeholder2%}");
str = str.replace(/goat/gi, "{%placeholder3%}");
Create an object that maps each placeholder to its corresponding replacement word:
var replacements = {
"{%placeholder1%}": "dog",
"{%placeholder2%}": "goat",
"{%placeholder3%}": "cat"
};
Use a single
replace()
method call, utilizing a callback function, to replace the placeholders with their respective replacement words:
str = str.replace(/(\{%placeholder\d+%\})/gi, function(match) {
return replacements[match];
});
VoilΓ ! You've replaced multiple strings with multiple other strings simultaneously! π
π‘ Example Result
After applying the solution to our initial problem, the updated code will look like this:
var str = "I have a cat, a dog, and a goat.";
str = str.replace(/cat/gi, "{%placeholder1%}");
str = str.replace(/dog/gi, "{%placeholder2%}");
str = str.replace(/goat/gi, "{%placeholder3%}");
var replacements = {
"{%placeholder1%}": "dog",
"{%placeholder2%}": "goat",
"{%placeholder3%}": "cat"
};
str = str.replace(/(\{%placeholder\d+%\})/gi, function(match) {
return replacements[match];
});
// The result is "I have a dog, a goat, and a cat"
π£ Call-to-Action: Join the Conversation! π£οΈ
Now that you know the secret to replacing multiple strings with multiple other strings at the same time in JavaScript, put your knowledge into action and share your experience with others. Have you encountered any other interesting string substitution challenges? Let me know in the comments section below! πβ¨
Remember, sharing is caring! If you found this blog post helpful, don't hesitate to spread the word by sharing it with your friends and colleagues. Happy coding! ππ₯
Note: This blog post was inspired by a real question posted on a coding forum. The original context and code snippet were modified for clarity and educational purposes.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
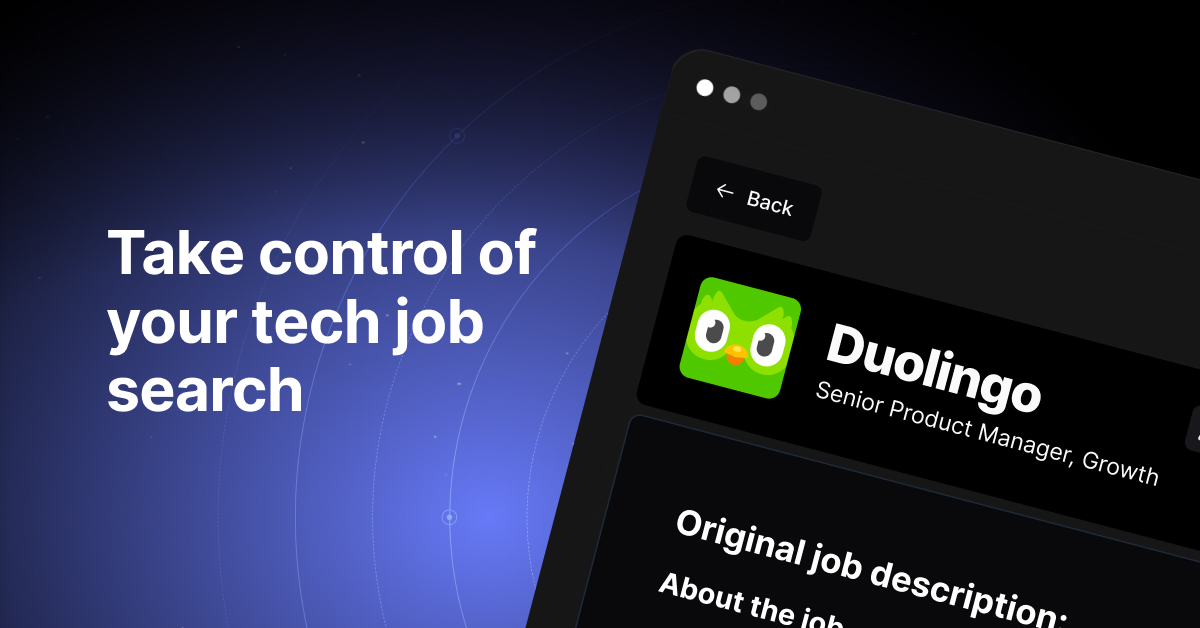