Repeat a string in JavaScript a number of times
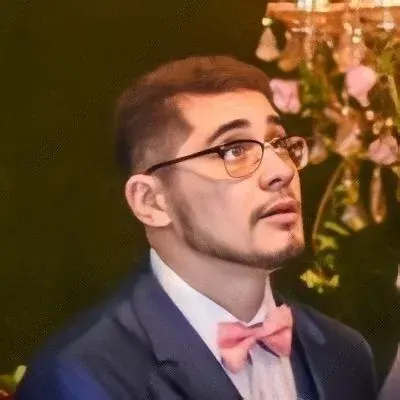
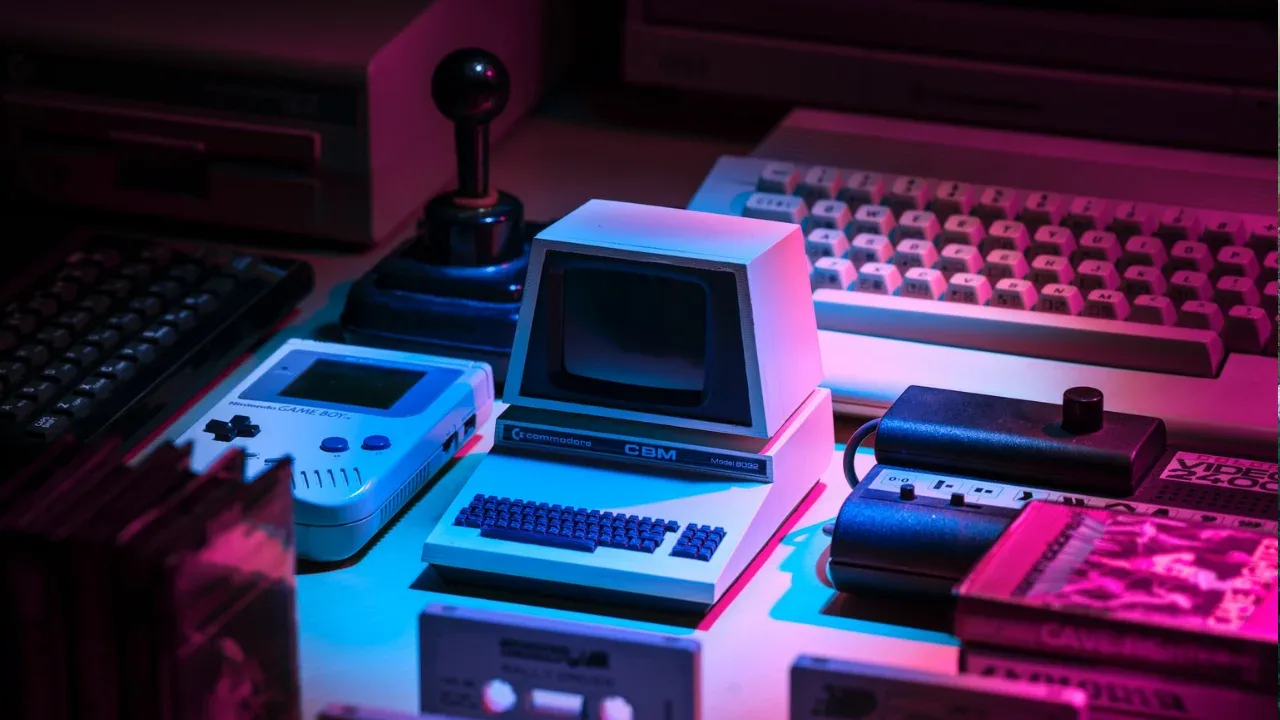
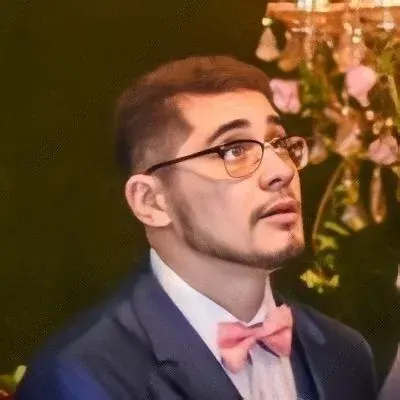
Blog Post Title: "🔁️ How to Repeat a String in JavaScript: The Simple and Clever Way"
Introduction
Are you struggling to repeat a string in JavaScript? 🤔 Fear not, my friend! You're in the right place. In this blog post, we'll explore a simple yet clever way to accomplish this task. Whether you need to duplicate a character multiple times or repeat an entire word, we've got you covered. Let's dive in! 💪
The Problem: Repeating a String
So, you want to repeat a string in JavaScript, just like they do in Perl? We've all been there. Let's consider the following challenge:
"How can I repeat a character or a word multiple times efficiently and without having to write a complex function?"
The Solution: Clever and Built-in Approach
Luckily for you, JavaScript offers a built-in approach to repeat a string. 🎉 And here's the secret:
const repeatedString = "a".repeat(10); // Results in "aaaaaaaaaa"
Yes, it's as simple as that! The repeat()
method, available in JavaScript, allows you to repeat any string a specified number of times. In the example above, we repeat the letter "a" ten times. Super convenient, isn't it? 🙌
Let's break it down:
repeat()
is a method that can be called on any string in JavaScript.Inside the parentheses, you specify the number of times you want to repeat the string.
The method returns a new string with the repeated value.
Common Issues and Additional Tips
While using the repeat()
method is pretty straightforward, let's address some common issues and share a few additional tips to level up your JavaScript skills. 😉
Validating the Number of Repeats
It's important to note that the argument passed to the repeat()
method must be a positive integer. Otherwise, you'll encounter a RangeError
. To avoid this error, you can add a simple check using the Number.isInteger()
method, like this:
const repeatString = (str, times) => {
if (Number.isInteger(times) && times >= 0) {
return str.repeat(times);
} else {
throw new Error("Invalid number of repeats provided. Please use a positive integer.");
}
};
try {
const repeatedString = repeatString("a", 10);
console.log(repeatedString); // "aaaaaaaaaa"
} catch (error) {
console.error(error.message);
}
Duplicating Words and Longer Strings
While our initial example focused on repeating a single character, the repeat()
method works equally well for duplicating words or longer string sequences. Let's see some examples:
const repeatedWord = "Hello ".repeat(3); // Results in "Hello Hello Hello "
const repeatedSequence = "JavaScript is ".repeat(5); // Results in "JavaScript is JavaScript is JavaScript is JavaScript is JavaScript is "
Feel free to unleash your creativity and experiment with various use cases. The possibilities are endless! 🚀
Call-to-Action and Reader Engagement
Now that you've learned this handy trick, it's time to put it into action! Try using the repeat()
method in your next JavaScript project and let us know how it goes. Share your experience in the comments below! We'd love to hear about your creative ideas and use cases. 👇
Conclusion
Repeating a string in JavaScript might have seemed challenging at first, but now you know the simple and clever way to do it. Thanks to the built-in repeat()
method, you can save yourself from writing unnecessary repetitive code. Remember to validate the number of repeats if required, and don't hesitate to explore even more possibilities with longer strings or words. Happy coding! 😄
That's all folks! 🎬 We hope you found this blog post enlightening and engaging. Don't forget to share it with your friends who might also be struggling with string repetition in JavaScript. Until next time, keep coding and enjoy unleashing the power of JavaScript! 💻✨