Rendering raw html with reactjs
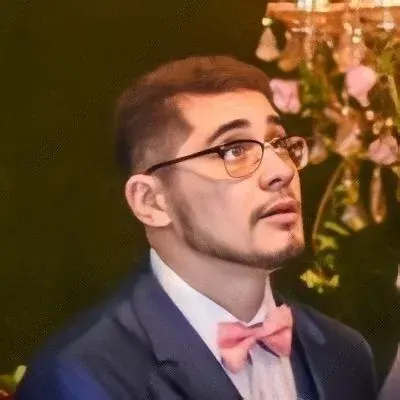
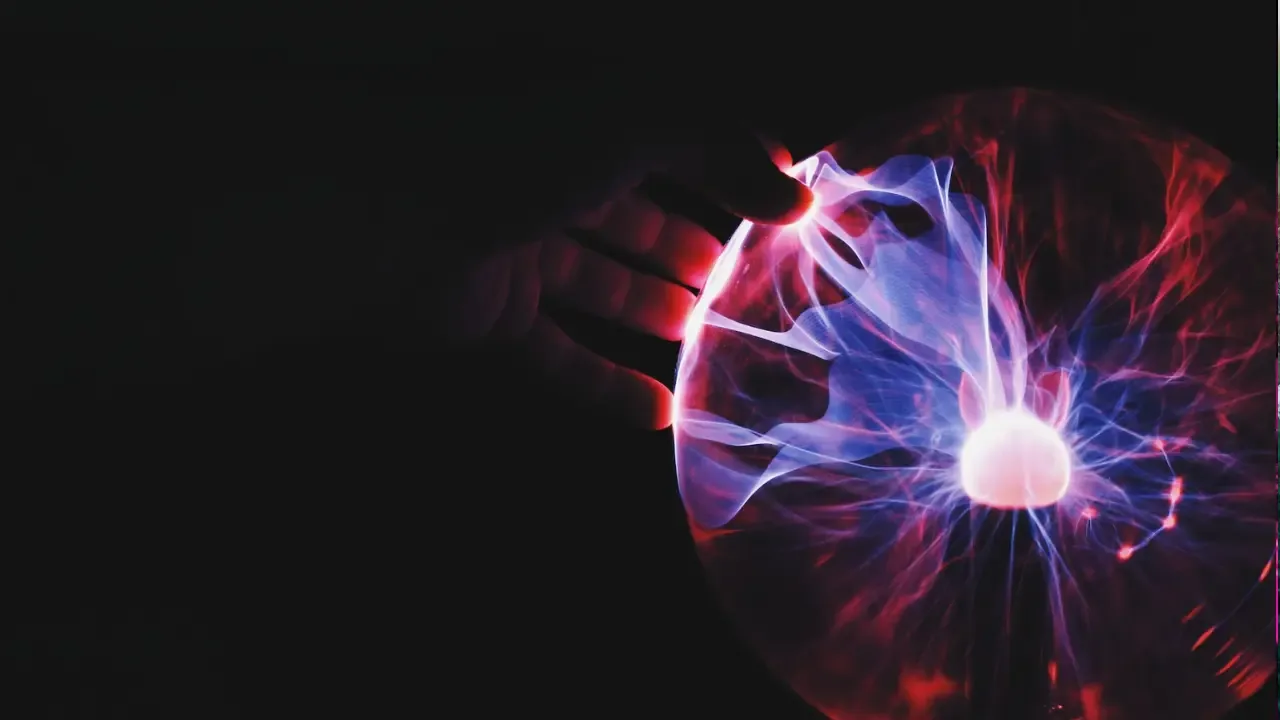
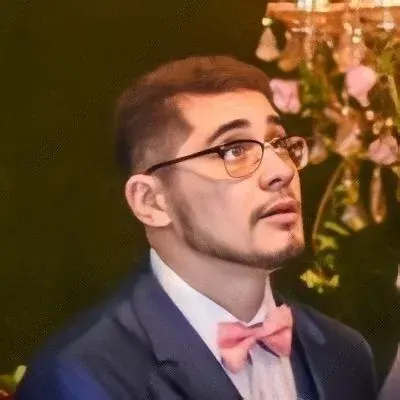
Rendering Raw HTML with ReactJS: A Guide for Easy Markup
Are you trying to render raw HTML with ReactJS, but finding it difficult to preserve all the classes, inline styles, and other elements? You're not alone! In this guide, we'll explore common issues when rendering raw HTML with ReactJS and provide easy solutions that will save you from having to rewrite everything in JSX.
The Challenge
Let's take a look at the code you provided:
// http://facebook.github.io/react/docs/tutorial.html
// tutorial7.js
var converter = new Showdown.converter();
var Comment = React.createClass({
render: function() {
var rawMarkup = converter.makeHtml(this.props.children.toString());
return (
<div className="comment">
<h2 className="commentAuthor">
{this.props.author}
</h2>
<span dangerouslySetInnerHTML={{__html: rawMarkup}} />
</div>
);
}
});
This code demonstrates how to render raw HTML using ReactJS. It utilizes the dangerouslySetInnerHTML
property to inject the raw markup into the component. However, it doesn't directly preserve the classes, inline styles, or other elements in the HTML.
Easy Solutions
Solution 1: Manually Convert HTML to JSX
One way to handle this issue is to manually convert the HTML to JSX. Although this might seem daunting, it can be a simple process if you follow these steps:
Copy the raw HTML you want to render with all the classes, inline styles, etc.
In your React component's render method, paste the HTML as a string.
Make sure to escape any special characters, such as double quotes, by replacing them with their respective HTML entities.
Wrap the HTML string inside a
div
or any other suitable parent element.
Here's an example implementation:
class MyComponent extends React.Component {
render() {
const rawHtml = `
<div class="dropdown">
<button class="btn btn-default dropdown-toggle" type="button" id="dropdownMenu1" data-toggle="dropdown" aria-expanded="true">
Dropdown
<span class="caret"></span>
</button>
<ul class="dropdown-menu" role="menu" aria-labelledby="dropdownMenu1">
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Action</a></li>
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Another action</a></li>
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Something else here</a></li>
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Separated link</a></li>
</ul>
</div>
`;
return <div dangerouslySetInnerHTML={{ __html: rawHtml }} />;
}
}
By manually converting the HTML to JSX, you can achieve the desired result without rewriting everything.
Solution 2: Use a React HTML Parser
If manually converting HTML to JSX feels overwhelming or time-consuming, you can leverage a React HTML parser library that does the heavy lifting for you. These libraries parse the HTML and transform it into JSX elements that can be easily rendered by React.
One popular example is the html-react-parser
library. It allows you to parse raw HTML and render it as JSX in your React components.
Here's an example of how to use html-react-parser
:
Install the library using npm:
npm install html-react-parser
.Import the
parse
function from the library.Pass the raw HTML as a string to the
parse
function, and it will return the corresponding JSX elements.Render the JSX elements as usual in your React component.
import parse from 'html-react-parser';
class MyComponent extends React.Component {
render() {
const rawHtml = `
<div class="dropdown">
<button class="btn btn-default dropdown-toggle" type="button" id="dropdownMenu1" data-toggle="dropdown" aria-expanded="true">
Dropdown
<span class="caret"></span>
</button>
<ul class="dropdown-menu" role="menu" aria-labelledby="dropdownMenu1">
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Action</a></li>
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Another action</a></li>
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Something else here</a></li>
<li role="presentation"><a role="menuitem" tabindex="-1" href="#">Separated link</a></li>
</ul>
</div>
`;
const jsxElements = parse(rawHtml);
return <>{jsxElements}</>; // Render the JSX elements
}
}
By using a React HTML parser like html-react-parser
, you can easily render raw HTML without the hassle of converting it manually to JSX.
Wrapping Up
Rendering raw HTML with ReactJS doesn't have to be a headache. Whether you prefer manual conversion or leveraging a library like html-react-parser
, you have easy solutions at your disposal.
Now that you know how to tackle this challenge, go ahead and give it a try in your React projects. Simplify your workflow and save time by rendering raw HTML effortlessly.
Have you ever encountered difficulties rendering raw HTML with ReactJS? How did you solve them? Share your experiences and insights in the comments below!
🚀 Happy coding! 🎉