Render basic HTML view?
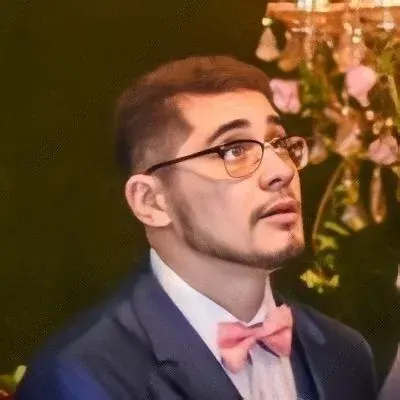
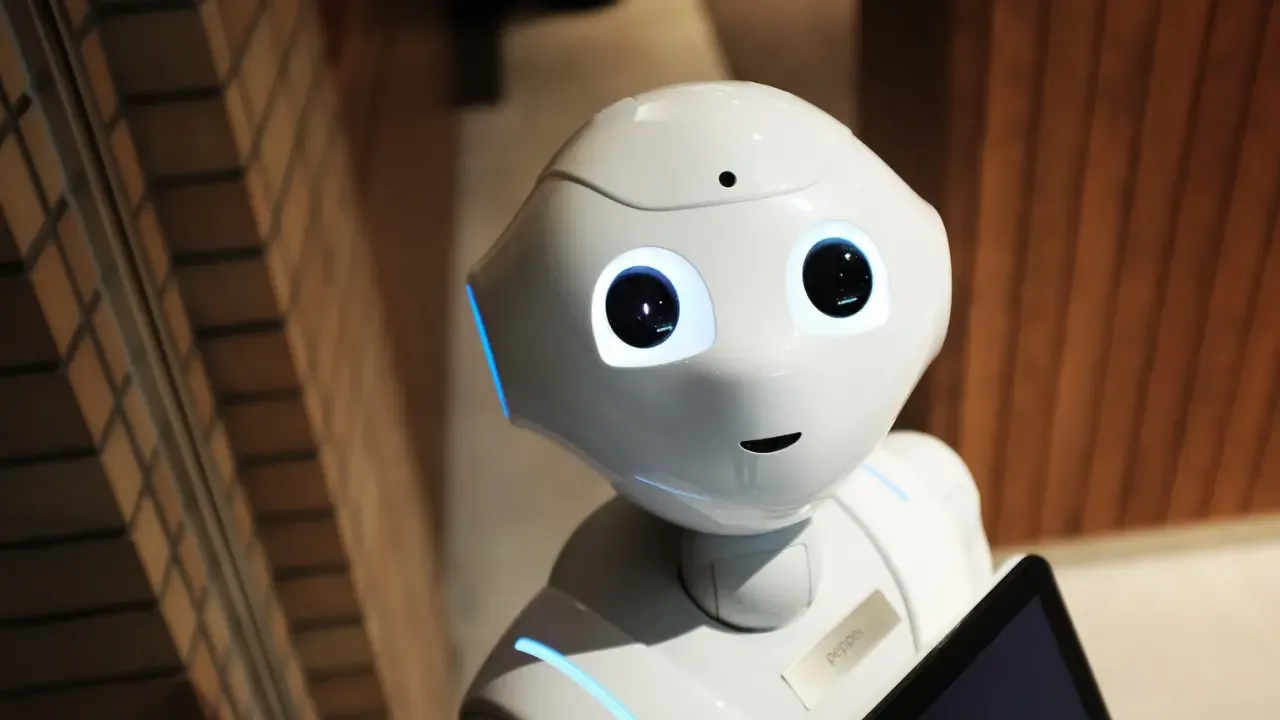
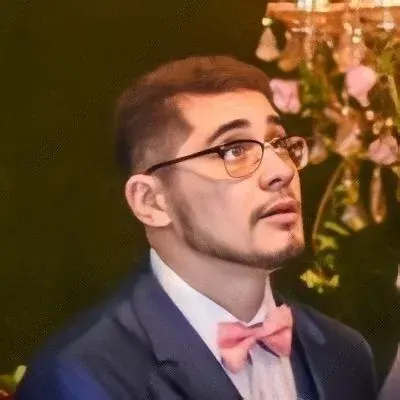
🌟 Rendering Basic HTML View in Node.js with Express 🌟
So, you've been working on a Node.js app using the Express framework, and you're trying to render a basic HTML view. However, you hit a roadblock and encountered the dreaded "Cannot find module 'html'" error. 😱
Don't worry, my friend! I've got your back and will guide you through this common issue step by step. Let's solve it together! 💪
🚦 Error Assessment
First, let's delve into the error message and understand what it's telling us. The error "Cannot find module 'html'" indicates that there's an issue with loading the required module for rendering HTML files.
⚠️ Possible Cause
Based on the context provided, it seems like you might not have set up your environment properly and installed the necessary dependencies.
💡 Solution
Install Dependencies: The simplest solution is to ensure that you have the required dependencies installed. Open your terminal and navigate to your project directory. Then, run the following command to install Express and other necessary packages:
npm install express
This command will install the required dependencies defined in your
package.json
file.Import the HTML Module: Update your code to import the HTML module correctly. Replace:
var express = require('express');
with:
var path = require('path');
The
path
module is a core module in Node.js that handles file and directory paths, including HTML files.Set up the View Engine: To render HTML views in Express, you need to configure a view engine. In your code, add the following line before setting up your routes:
app.set('views', path.join(__dirname, 'views')); app.set('view engine', 'html');
This code sets the
views
directory as the location for your HTML files and specifies the view engine as'html'
. Make sure you have anindex.html
file in theviews
directory.Modify Route Rendering: Update your route to render the HTML file correctly. Replace:
app.get('/', function(req, res) { res.render('index.html'); });
with:
app.get('/', function(req, res) { res.sendFile('index.html', { root: './views' }); });
This change ensures that Express sends the HTML file correctly to the browser.
Start the Application: Save your changes, and in your terminal, start your Node.js application by running:
node app.js
Replace
app.js
with the actual filename if different.
📣 Your Turn!
That's it! You've successfully resolved the "Cannot find module 'html'" error and can now render basic HTML views using Express in your Node.js app. Give it a spin and see your web page in action! 🎉
If you encounter any more issues or have other questions, feel free to leave a comment below. Let's keep the conversation going! 😊
🚀 Call-to-Action: Share Your Experience!
Have you encountered this error before or experienced any other challenges while rendering HTML views in Node.js? Share your stories and lessons learned in the comments section below. Your insights could help fellow developers overcome similar hurdles!
And don't forget to share this blog post with your friends and colleagues who are also diving into the exciting world of Node.js and Express. Sharing is caring, after all! 🤗
Happy coding! 💻✨